Backtracking Algorithm
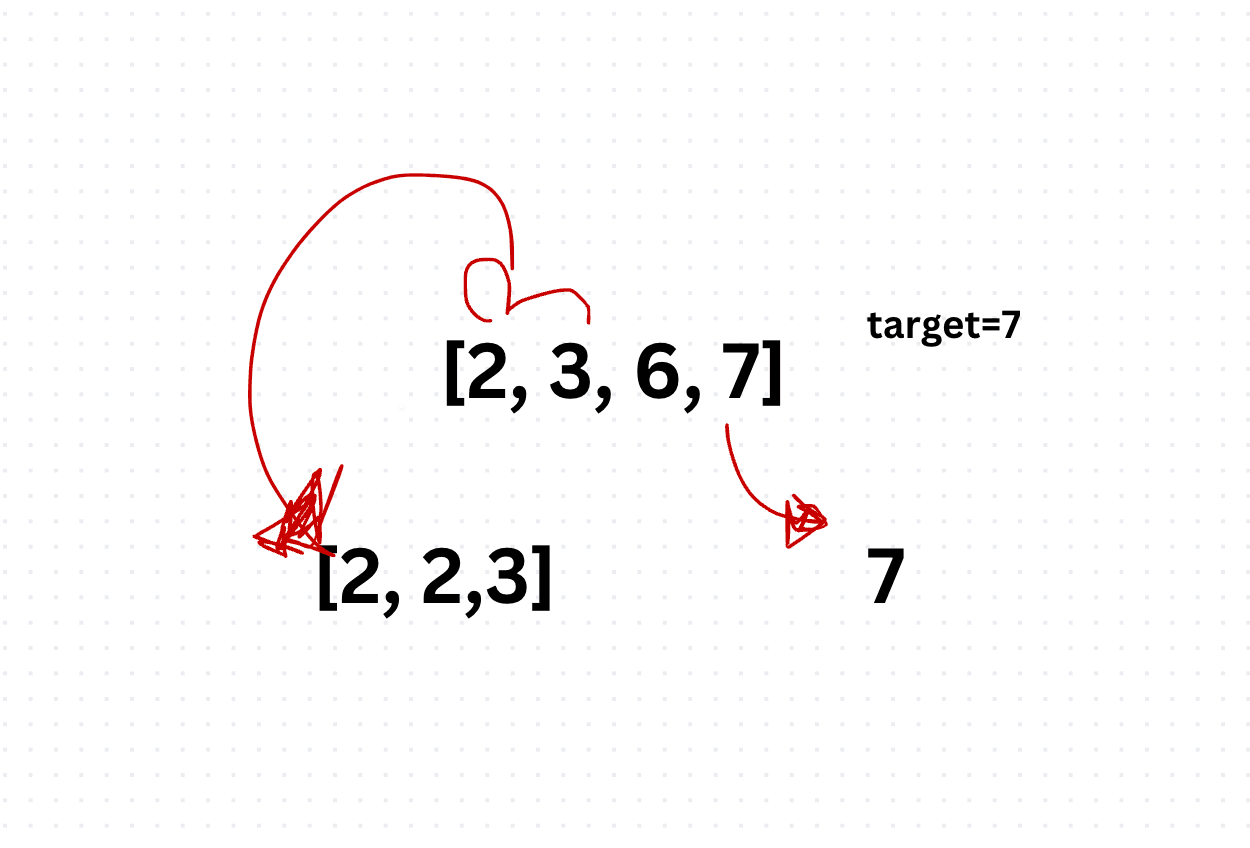
Backtracking is a powerful algorithmic technique used to systematically search for solutions to a problem by trying different sequences of decisions. In this technical article, we will delve into the intricacies of backtracking algorithms, discuss their principles, and provide JavaScript implementations. Real-world examples will illustrate the significance and practical usage of backtracking in solving combinatorial optimization problems.
Backtracking is a systematic way of searching for solutions to problems that involve making a sequence of decisions. It involves exploring all possible solutions and backtracking when a dead-end is reached, i.e., when no further progress can be made.
function backtrack(solution, candidates, target, startIndex, current, results) {
if (current === target) {
results.push(solution.slice());
return;
}
if (current > target || startIndex >= candidates.length) {
return;
}
for (let i = startIndex; i < candidates.length; i++) {
solution.push(candidates[i]);
backtrack(solution, candidates, target, i, current + candidates[i], results);
solution.pop();
}
}
function combinationSum(candidates, target) {
const results = [];
backtrack([], candidates, target, 0, 0, results);
return results;
}
// Example usage:
const candidates = [2, 3, 6, 7];
const target = 7;
console.log("Combination Sum:", combinationSum(candidates, target));
Backtracking algorithms find applications in various domains, including puzzle-solving, constraint satisfaction, and optimization problems such as the Traveling Salesman Problem and Sudoku solving.