Breadth-First Search (BFS) Algorithm
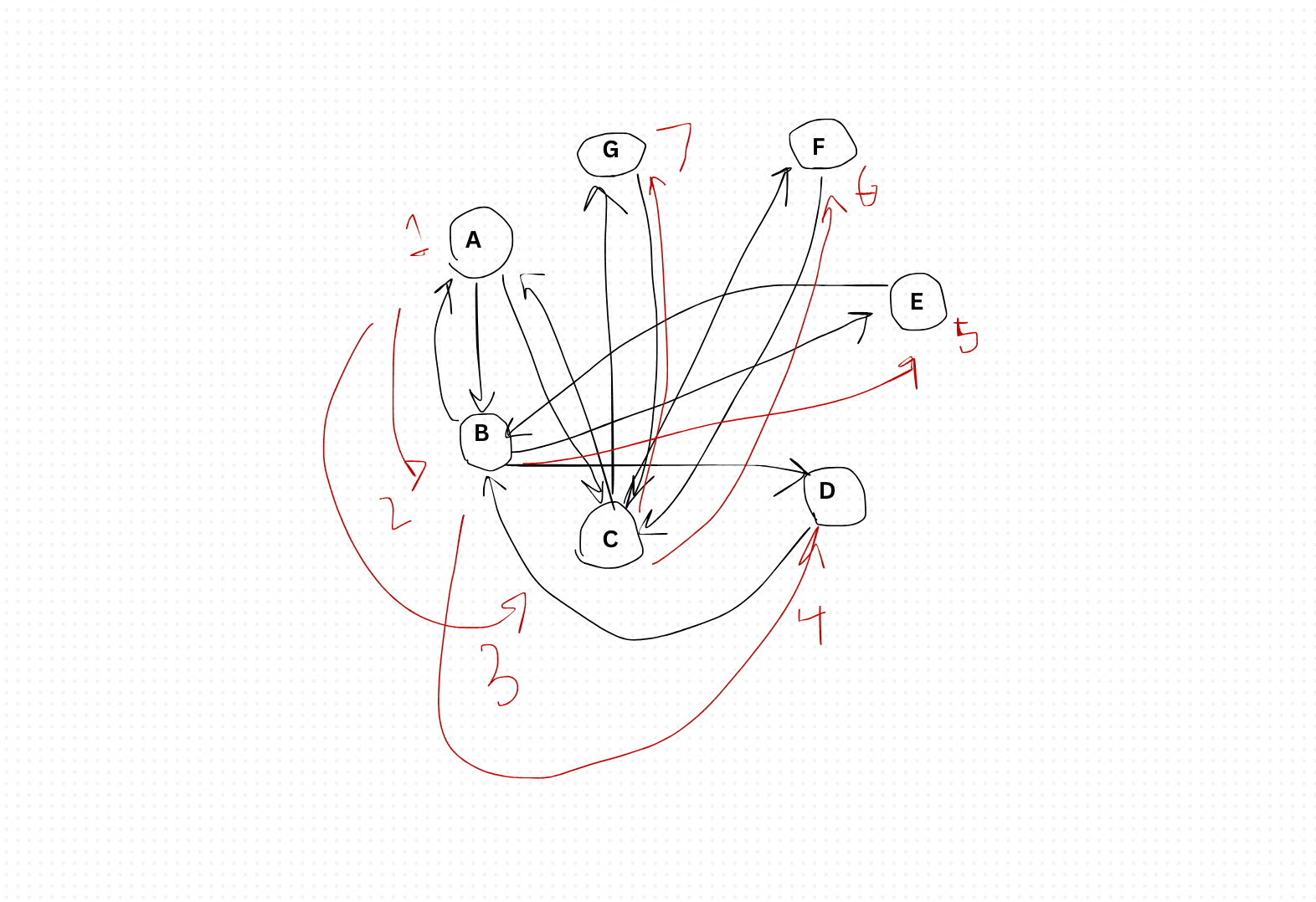
Breadth-First Search (BFS) is a fundamental algorithm used for traversing or searching tree or graph data structures level by level. It explores all the neighbor nodes at the present depth before moving on to the nodes at the next depth level. In this article, we'll undertake on a journey through the intricacies of BFS, understand its workings, and explore its applications in various domains.
Understanding Breadth-First Search:
BFS operates by starting at a designated node (usually called the "source" node) and systematically explores its neighbors before moving on to their neighbors. It continues this process until it has visited all reachable nodes or until a specific condition is met. BFS ensures that all nodes at the current depth level are visited before proceeding to nodes at deeper levels.
Implementation in JavaScript:
Below is the implementation of the Breadth-First Search algorithm in JavaScript:
const graph = {
A: ['B', 'C'],
B: ['A', 'D', 'E'],
C: ['A', 'F', 'G'],
D: ['B'],
E: ['B'],
F: ['C'],
G: ['C']
};
const bfs = (graph, start) => {
const visited = {};
const queue = [start];
visited[start] = true;
while (queue.length > 0) {
const current = queue.shift();
console.log(current);
for (const neighbor of graph[current]) {
if (!visited[neighbor]) {
queue.push(neighbor);
visited[neighbor] = true;
}
}
}
}
bfs(graph, 'A');
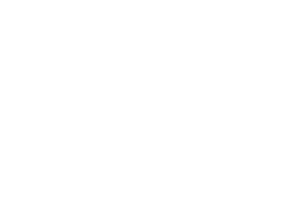
In this example, the bfs function explores the graph starting from node 'A' and systematically traverses neighboring nodes level by level.
Applications of Breadth-First Search:
- Shortest Path Finding: BFS is adept at finding the shortest path between two nodes in an unweighted graph.
- Minimum Spanning Tree: It's instrumental in finding the minimum spanning tree of a graph.
- Web Crawling: BFS powers web crawlers to methodically explore web pages starting from a given URL.
- Social Network Analysis: It facilitates the discovery of connections or relationships between individuals in social networks.
Conclusion:
Breadth-First Search emerges as a versatile algorithm with myriad applications across diverse fields. Its systematic approach to traversing nodes level by level makes it indispensable for navigating tree or graph data structures.