Quick Sort Algorithm
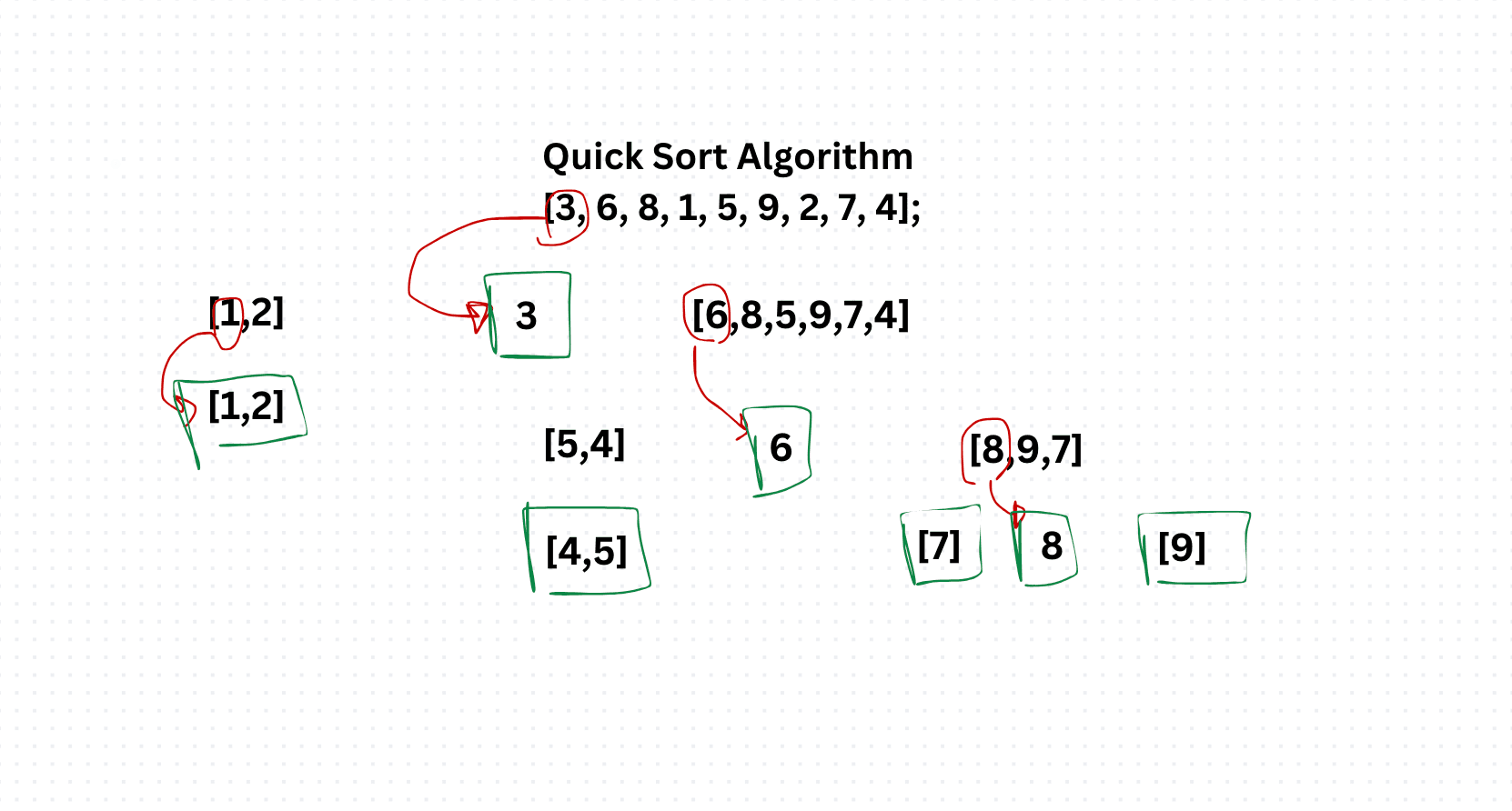
Quick Sort operates on the principle of partitioning. It selects a pivot element from the array and rearranges the elements such that all elements less than the pivot are placed before it, and all elements greater than the pivot are placed after it. This process partitions the array into two subarrays, which are then recursively sorted using the same technique. Eventually, the entire array becomes sorted through a sequence of partitioning steps.
Algorithm Implementation:
Below is the implementation of the Quick Sort algorithm in JavaScript:
const unsortedArray = [3, 6, 8, 1, 5, 9, 2, 7, 4];
const quickSort = (myArray) => {
if (myArray.length <= 1) {
return myArray;
}
let pivot = myArray[0];
let left = [];
let right = [];
for (let i = 1; i < myArray.length; i++) {
if (myArray[i] < pivot) {
left.push(myArray[i]);
} else {
right.push(myArray[i]);
}
}
return [...quickSort(leftArr), pivot, ...quickSort(rightArr)];
};
console.log(quickSort(unsortedArray));
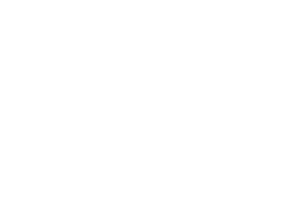
Time Complexity Analysis:
Quick Sort exhibits an average-case time complexity of O(n log n), making it highly efficient for large datasets. However, in the worst-case scenario, particularly when the pivot selection is poor, Quick Sort's time complexity can degrade to O(n^2). Despite this, its average-case performance makes it a preferred choice for many applications.
Strengths and Weaknesses:
Strengths:
- Excellent average-case performance for large datasets.
- In-place sorting, requiring minimal additional memory.
Weaknesses:
- Vulnerable to worst-case scenarios if pivot selection is suboptimal.
- Not stable, meaning the relative order of equal elements may not be preserved.
Conclusion:
Quick Sort stands as a beacon of efficiency in the world of sorting algorithms, admired for its speed and elegance. By leveraging the divide-and-conquer strategy and intelligent pivot selection, Quick Sort efficiently sorts large datasets with ease.