Selection Sort Algorithm
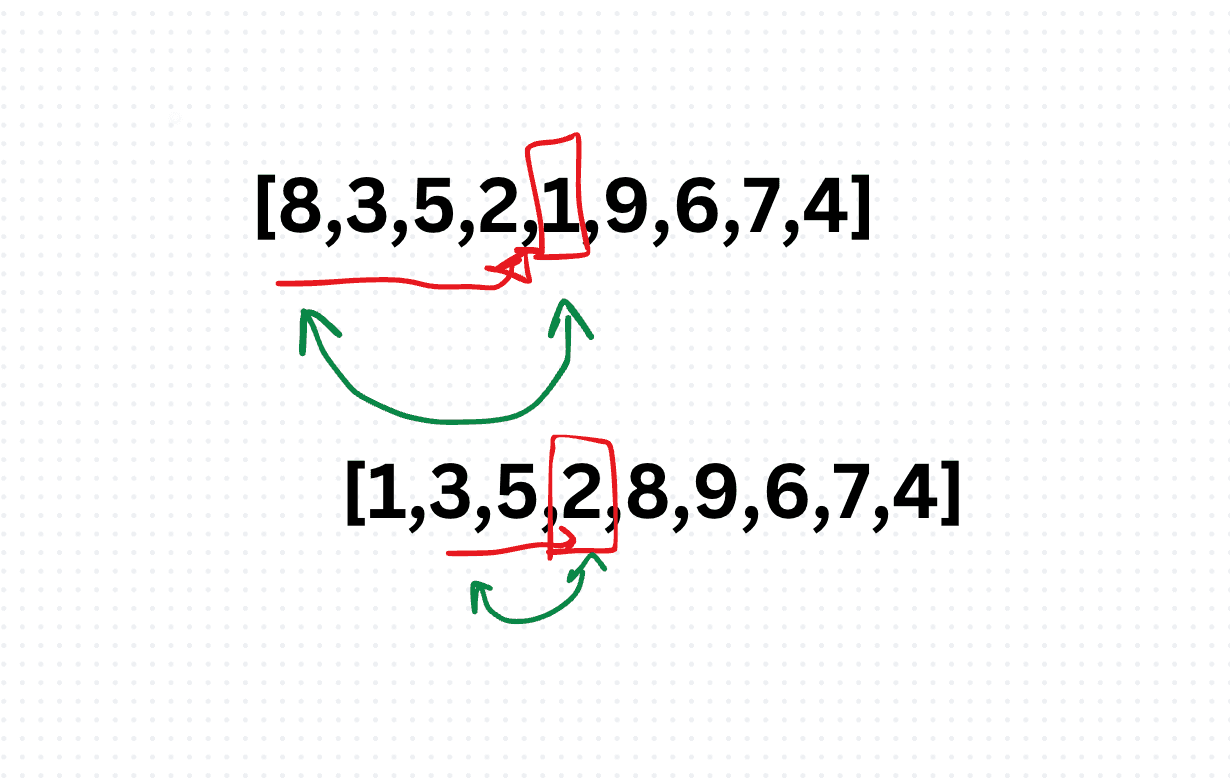
Sorting algorithms are fundamental in computer science and are used in various applications ranging from databases to algorithms. Selection sort is one of the simplest sorting algorithms, and understanding its implementation and performance characteristics can provide valuable insights into algorithmic design and analysis.
function selectionSort(arr) {
const n = arr.length;
for (let i = 0; i < n - 1; i++) {
let minIndex = i;
for (let j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex !== i) {
let temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
return arr;
}
The time complexity of selection sort is O(n^2), where n is the number of elements in the array. This is because the algorithm involves nested loops, where the outer loop runs n - 1 times and the inner loop runs n - i times on average, resulting in a quadratic time complexity.
While selection sort may not be the most efficient sorting algorithm for large datasets, it is a valuable learning tool for understanding sorting algorithms and algorithmic analysis.