Solving the Height of a Binary Tree Puzzle
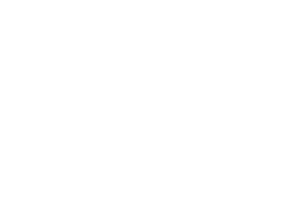
Given an array representation of a binary tree, where each element corresponds to a node in the tree, and -1 denotes a null node, the task is to determine the height of the binary tree.
Consider the array [1, 2, 3, 4, -1, -1, -1]. This array represents a binary tree.
The null nodes are denoted by -1. In this example, the height of the binary tree is 3.
class Node {
value;
left;
right;
constructor(value){
this.value = value;
}
}
class Tree {
root;
constructor(tree) {
if (tree.length === 0) {
return null;
}
const root = new Node(tree[0])
this.root = root;
const queue = [root]
let index = 1
while(index < tree.length) {
const currentNode = queue.shift();
if (tree[index] !== -1){
currentNode.left = new Node(tree[index])
queue.push(currentNode.left)
}
index++;
if (tree[index] !== -1 && !currentNode.right){
currentNode.right = new Node(tree[index])
queue.push(currentNode.right)
}
index++;
}
}
}
const getHeight = (rootNode) => {
if (!rootNode) return 0;
const leftMax = getHeight(rootNode.left)
const rightMax = getHeight(rootNode.right)
return Math.max(leftMax,rightMax) + 1
}
const solution = (tree) => {
if (!tree) tree
const treeObj = new Tree(tree)
return getHeight(treeObj.root)
}
console.log(solution([1,2,3,4,-1,-1,-1]))
By applying a recursive approach and leveraging the array representation of the binary tree, we successfully determined the height of the binary tree. This solution provides a valuable insight into solving similar problems in algorithmic programming, showcasing the power of recursive techniques and data structure manipulation.