Word Pattern Algorithm
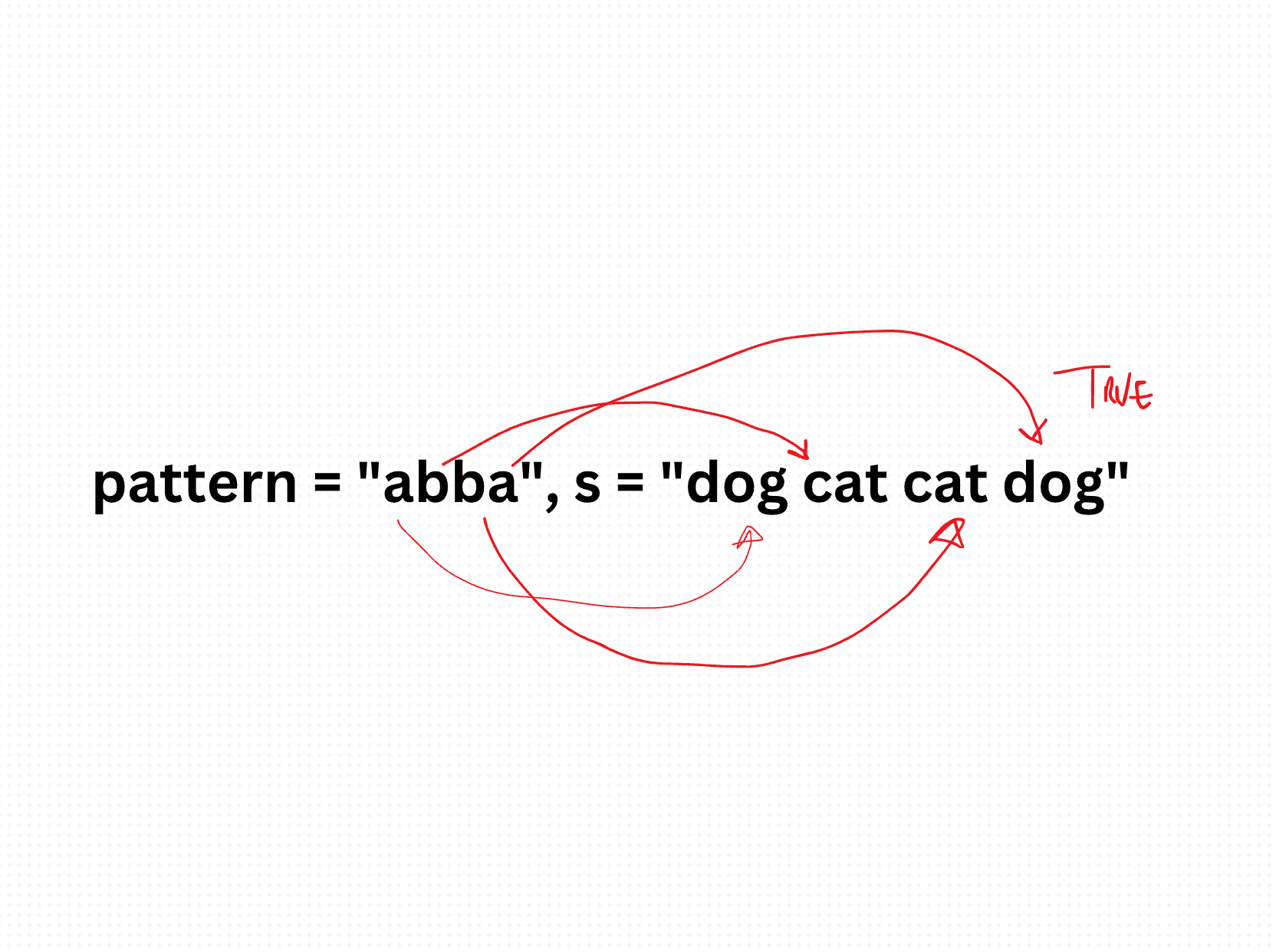
Given a pattern and a string s, find if s follows the same pattern.
Here follow means a full match, such that there is a bijection between a letter in pattern and a non-empty word in s.
Input: pattern = "abba", s = "dog cat cat dog"
Output: true
var wordPattern = function(pattern, word) {
const wordArray = word.trim().split(" ")
if (pattern.length !== wordArray.length) return false;
if (new Set(pattern).size !== new Set(wordArray).size) return false;
const mapObj = new Map()
for (let index = 0 ; index < pattern.length ; index++){
if (!mapObj.has(pattern[index])) {
mapObj.set(pattern[index],wordArray[index])
}
if (wordArray[index] !== mapObj.get(pattern[index])) return false
}
return true
};