Count Down
0
Count Up Automatically
0
Counter and Count Up App, Working with SetInterval and Refs
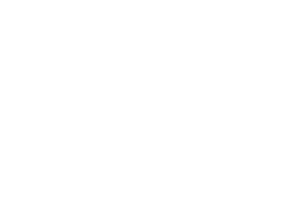
In modern web development, creating dynamic and interactive user interfaces is essential. In this technical article, we'll explore how to build a Counter and Count-Up app in React, leveraging the power of setInterval and refs. By the end of this tutorial, you'll have a solid understanding of how to implement real-time updates and manage DOM elements efficiently in React.
const ref = useRef(null);
const [counter, setCounter] = useState(0);
const [seconds, setSeconds] = useState(0);
const [start, setStart] = useState(false);
const { posts } = useLoaderData<{ posts: IPosts }>();
const post = posts?.data[0];
useEffect(() => {
if (start) {
ref.interval = setInterval(() => {
setSeconds((seconds) => seconds + 1);
}, 1000);
} else {
clearInterval(ref.interval);
}
return () => clearInterval(ref.interval);
}, [start]);
In this component, we use the useState hook to manage the count state and the useEffect hook to run a timer that increments the count every second using setInterval. We also clean up the interval using clearInterval in the cleanup function of useEffect.