00:00:00
MilliSeconds: 0
Milliseconds Counter App - Interview Question
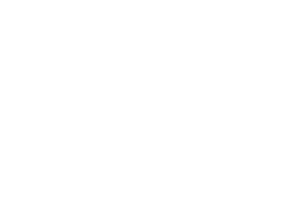
In this tutorial, we'll create a Milliseconds Counter App using React and TypeScript. This project will demonstrate how to build a simple web application to display a continuously updating count of milliseconds since the app was loaded. We'll leverage the power of React's component-based architecture along with TypeScript's type safety to create a clean and maintainable codebase.
Next, let's create a new TypeScript file named MillisecondsCounter.tsx in the src directory of your project. This file will contain the React component for our app:
const MilliSecondsCounter = () => {
const ref = useRef<{ interval: NodeJS.Timeout }>({ interval: null });
const [milliseconds, setMilliseconds] = useState(0);
const [started, setStarted] = useState(false);
const [startedAt, setStartedAt] = useState(0);
const start = () => {
setStarted(true);
setStartedAt(new Date().getTime());
};
const pause = () => {
setStarted(false);
};
useEffect(() => {
if (!started) {
clearInterval(ref.current.interval);
return;
}
ref.current.interval = setInterval(() => {
const elapsedTime = Date.now() - startedAt + milliseconds;
setMilliseconds(elapsedTime);
}, 1);
return () => clearInterval(ref.current.interval);
// eslint-disable-next-line react-hooks/exhaustive-deps
}, [started]);
return (
<Box>
<Button onClick={start}>Start</Button>
<Box>
<Heading as="h1" size="xl" textAlign={"center"}>
{new Date(milliseconds).toISOString().substr(11, 8)}
</Heading>
<Text
fontSize={"sm"}
textAlign={"center"}
color={"gray.500"}
py={4}
>
MilliSeconds:{" "}
</Text>
<Text as={"span"} id="milliseconds">
{milliseconds}
</Text>
</Box>
<Button onClick={pause}>Pause</Button>
</Box>
In this tutorial, we've built a simple Milliseconds Counter App using React and TypeScript. We've leveraged the power of React's component-based architecture along with TypeScript's type safety to create a clean and maintainable codebase. This project serves as a great starting point for further exploration and experimentation with React and TypeScript web development. Happy coding!