Linear Regression in Artificial Intelligence
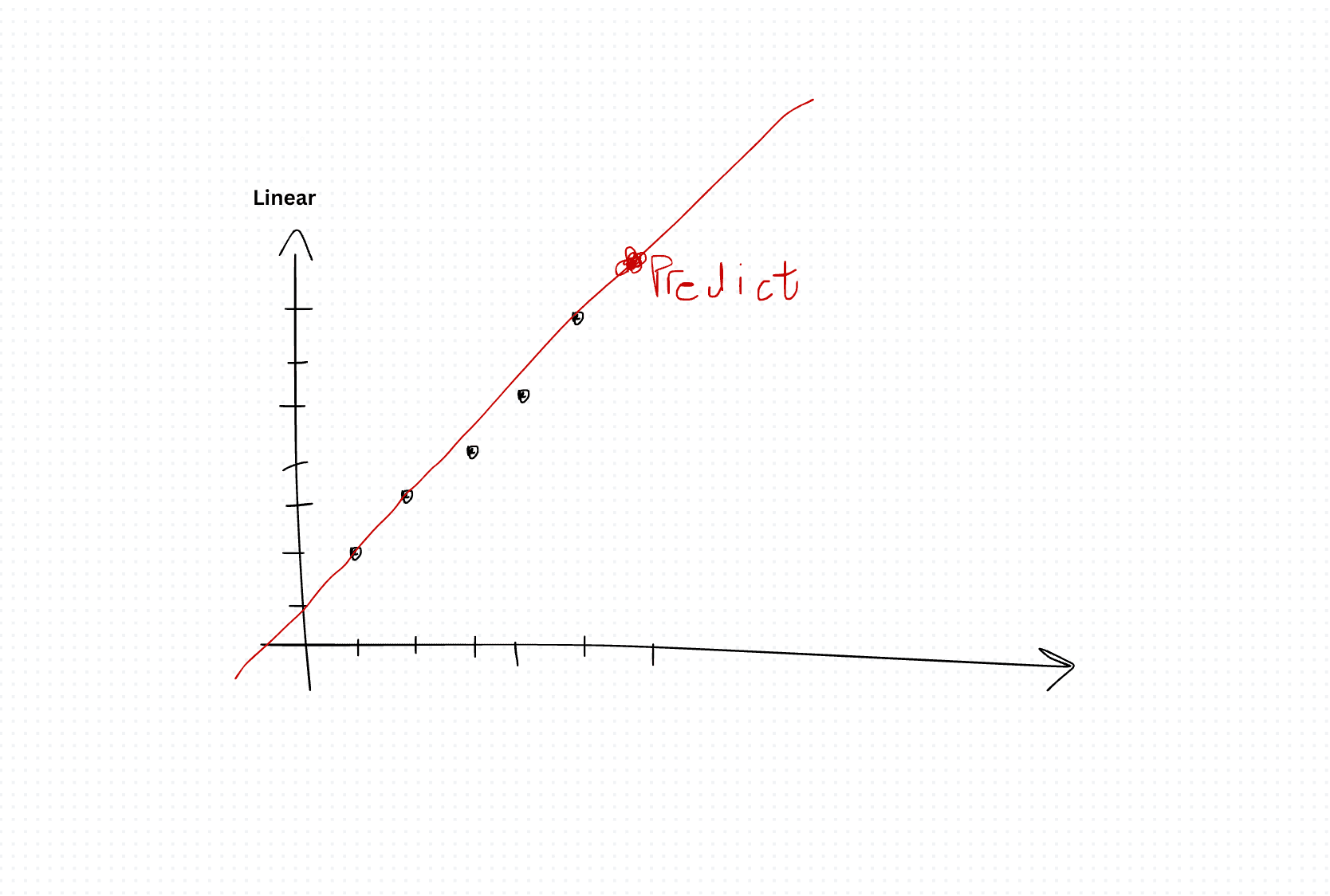
Linear regression is a foundational algorithm in machine learning, widely used for predicting continuous outcomes based on input features. In this article, we'll explore how to implement linear regression using TensorFlow.js, a JavaScript library for training and deploying machine learning models in the browser and on Node.js.
Linear regression aims to model the relationship between a dependent variable (target) and one or more independent variables (features) by fitting a linear equation to observed data. The equation for a simple linear regression model can be represented as:
y = mx + b
- y is the dependent variable (target)
- x is the independent variable (feature)
- m is the slope of the line (coefficient)
- b is the y-intercept
The goal of linear regression is to find the values of m and b that minimize the difference between the predicted values and the actual values in the training data.
// Import TensorFlow.js
const tf = require('@tensorflow/tfjs');
// Define sample training data (input features and corresponding target values)
const X = tf.tensor2d([[1], [2], [3], [4], [5]]);
const Y = tf.tensor2d([[2], [3], [4], [5], [6]]);
// Define the linear regression model
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Compile the model
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
// Train the model
async function trainModel() {
await model.fit(X, Y, {epochs: 100});
console.log('Model training complete.');
// Get the trained slope (m) and y-intercept (b)
const weights = model.getWeights();
const slope = weights[0].dataSync()[0];
const yIntercept = weights[1].dataSync()[0];
console.log(`Slope: ${slope}, Y-intercept: ${yIntercept}`);
// Predict the target value for a new input feature
const newX = tf.tensor2d([[6]]);
const predictedY = model.predict(newX);
predictedY.print();
}
trainModel();
// Model training complete.
// Slope: 1.1759456396102905, Y-intercept: 0.36478060483932495
// Tensor
// [[7.420454],]
TensorFlow.js provides a powerful platform for implementing machine learning algorithms in JavaScript, including linear regression. In this article, we demonstrated how to implement linear regression using TensorFlow.js to predict continuous outcomes based on input features. Linear regression is a fundamental technique in machine learning, with applications in various domains such as finance, healthcare, and engineering.