Web3.js: A Casual Developer's Guide
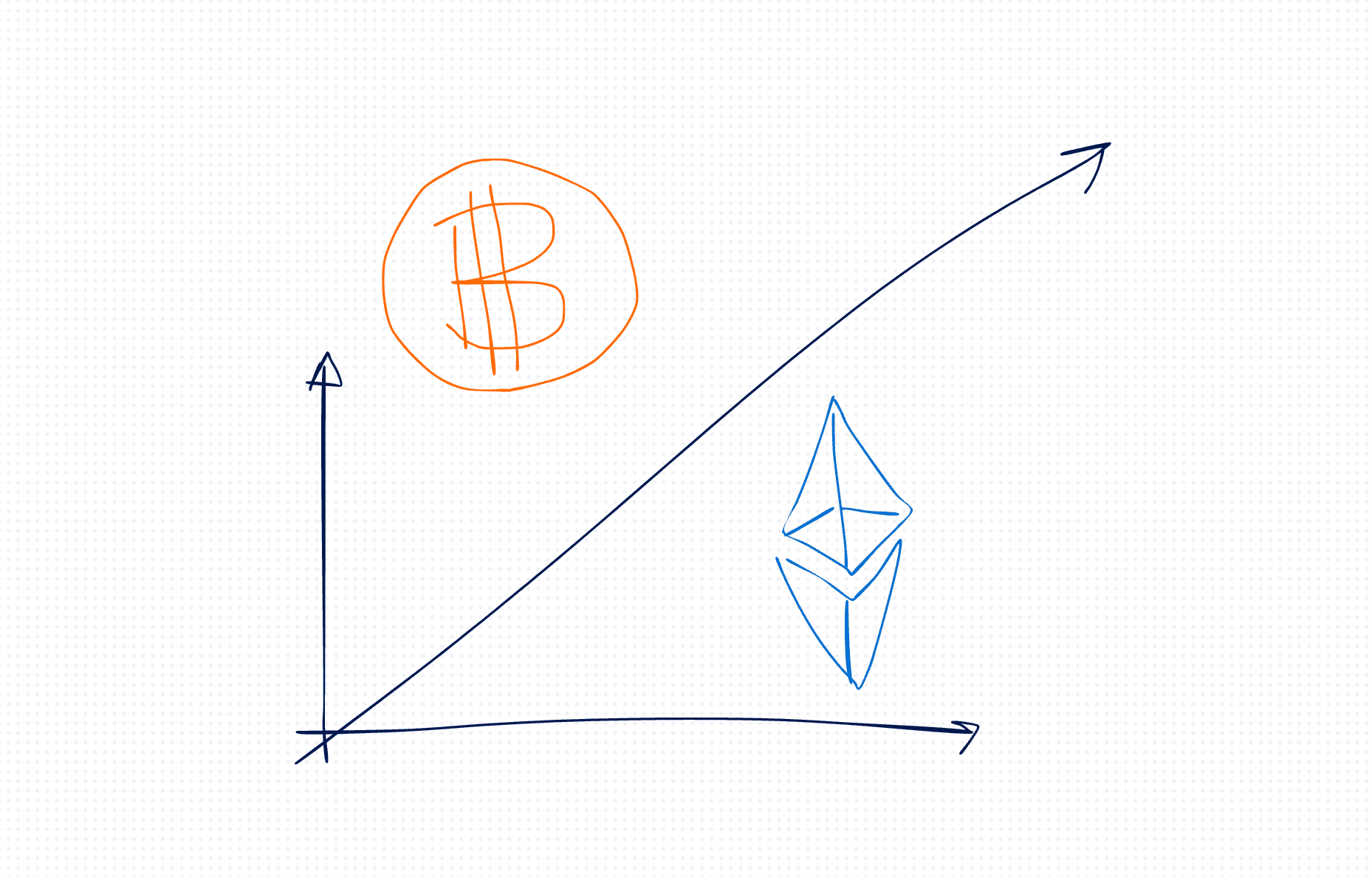
Are you curious about building decentralized applications (dApps) on the Ethereum blockchain? Look no further! In this guide, we'll take a relaxed approach to understanding Web3.js, the JavaScript library that makes interacting with Ethereum smart contracts a breeze. With detailed examples and a casual tone, you'll be well on your way to becoming a blockchain developer extraordinaire!
Now that bitcoin and crypto are back up again, 67,412.40USD!
Understanding Web3.js:
Think of Web3.js as your trusty sidekick in the world of Ethereum development. It's like having a magic wand that lets you communicate with the blockchain from your JavaScript applications. With Web3.js, you can send transactions, deploy smart contracts, and retrieve data from the Ethereum network, all without breaking a sweat.
Setting Up Web3.js:
First things first, let's get Web3.js up and running in our project. If you're using Node.js, simply install it via npm:
npm install web3
Connecting to Ethereum:
Now that we have Web3.js installed, it's time to connect to the Ethereum network. If you're just testing things out, you can use Infura, a popular Ethereum node provider. Grab your Infura project ID and initialize Web3.js like below.
Note that, there are many node providers out there, like Infura, Alchemy, QuickNode and so on
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
Sending Transactions:
Sending transactions on Ethereum is as easy as pie with Web3.js. Let's say you want to send some ether from one account to another:
const senderAddress = '0xYourAddress';
const receiverAddress = '0xReceiverAddress';
web3.eth.sendTransaction({
from: senderAddress,
to: receiverAddress,
value: web3.utils.toWei('1', 'ether')
})
.then(receipt => console.log('Transaction successful! Receipt:', receipt))
.catch(error => console.error('Transaction failed:', error));
Deploying Smart Contracts:
Ready to deploy your first smart contract? Web3.js has got your back. Here's a simple example of deploying a contract:
const contractData = require('./SimpleContract.json');
const contract = new web3.eth.Contract(contractData.abi);
contract.deploy({
data: contractData.bytecode,
arguments: [123] // constructor arguments
})
.send({
from: '0xYourAddress',
gas: 2000000
})
.then(newContractInstance => console.log('Contract deployed at:', newContractInstance.options.address))
.catch(error => console.error('Contract deployment failed:', error));
Interacting with Smart Contracts:
Once your contract is deployed, you can interact with it using Web3.js. Let's call a method on our deployed contract:
const contractAddress = '0xContractAddress';
const contractInstance = new web3.eth.Contract(contractData.abi, contractAddress);
contractInstance.methods.get().call()
.then(value => console.log('Current value:', value))
.catch(error => console.error('Method call failed:', error));
What are the other Etherium Node Providers?
Here is just 3 of many:
When it comes to Ethereum node providers, there are several options available, each offering unique features and capabilities. Here are three popular Ethereum node providers:
Infura:
Infura is one of the most widely used Ethereum node providers, offering reliable and scalable infrastructure for developers building decentralized applications.
- It provides both mainnet and testnet nodes, along with WebSocket support for real-time updates.
- Infura offers a free tier with limited requests per day and paid plans for higher usage.
Alchemy:
- Alchemy is a powerful Ethereum infrastructure provider that offers high-performance API endpoints for interacting with the Ethereum network.
- It provides robust developer tools and features such as analytics, monitoring, and debugging tools.
- Alchemy offers a free tier for developers with limited requests per day, along with paid plans for higher usage and additional features.
QuickNode:
QuickNode offers Ethereum nodes and APIs for developers looking to build and deploy decentralized applications.
- It provides dedicated nodes with customizable configurations, allowing developers to tailor their infrastructure to their specific needs.
- QuickNode offers a range of plans, including free tiers for development purposes and paid plans for production deployments.
What is Mainnet?
Here are few options, however Mainnet is the real one.
Mainnet, Goerli, and Rinkeby are all different Ethereum networks, each serving a specific purpose in the Ethereum ecosystem:
Mainnet:
Mainnet is the primary Ethereum network where real transactions occur using actual Ether (ETH) and ERC-20 tokens.
- It is the live, production-ready blockchain network that processes and validates transactions involving Ether and various decentralized applications (DApps).
- Transactions on the mainnet are irreversible and have real economic value, making it the network of choice for most users and applications.
Goerli:
Goerli is one of the Ethereum test networks, specifically designed for testing and development purposes.
- It operates similarly to the mainnet but uses test Ether (GöETH), which has no real-world value.
- Developers use the Goerli network to test their smart contracts, decentralized applications, and other Ethereum-related tools without risking real funds.
- Goerli is one of several Ethereum test networks and is widely used by developers due to its stability and reliability.
Rinkeby:
Rinkeby is another Ethereum test network similar to Goerli, providing developers with a sandbox environment for testing and experimentation.
- Like Goerli, Rinkeby uses test Ether (ETH) and is primarily used for testing smart contracts, DApps, and other Ethereum-related projects.
- Rinkeby is known for its compatibility with MetaMask and other popular Ethereum tools, making it a preferred choice for many developers.
Congratulations! You've taken your first steps into the exciting world of Ethereum development with Web3.js. With its intuitive APIs and detailed examples like the ones provided in this guide, you're well-equipped to start building your own decentralized applications. So grab your favorite beverage, fire up your code editor, and let your imagination run wild in the world of blockchain!