How to develop an event driven app in NodeJS
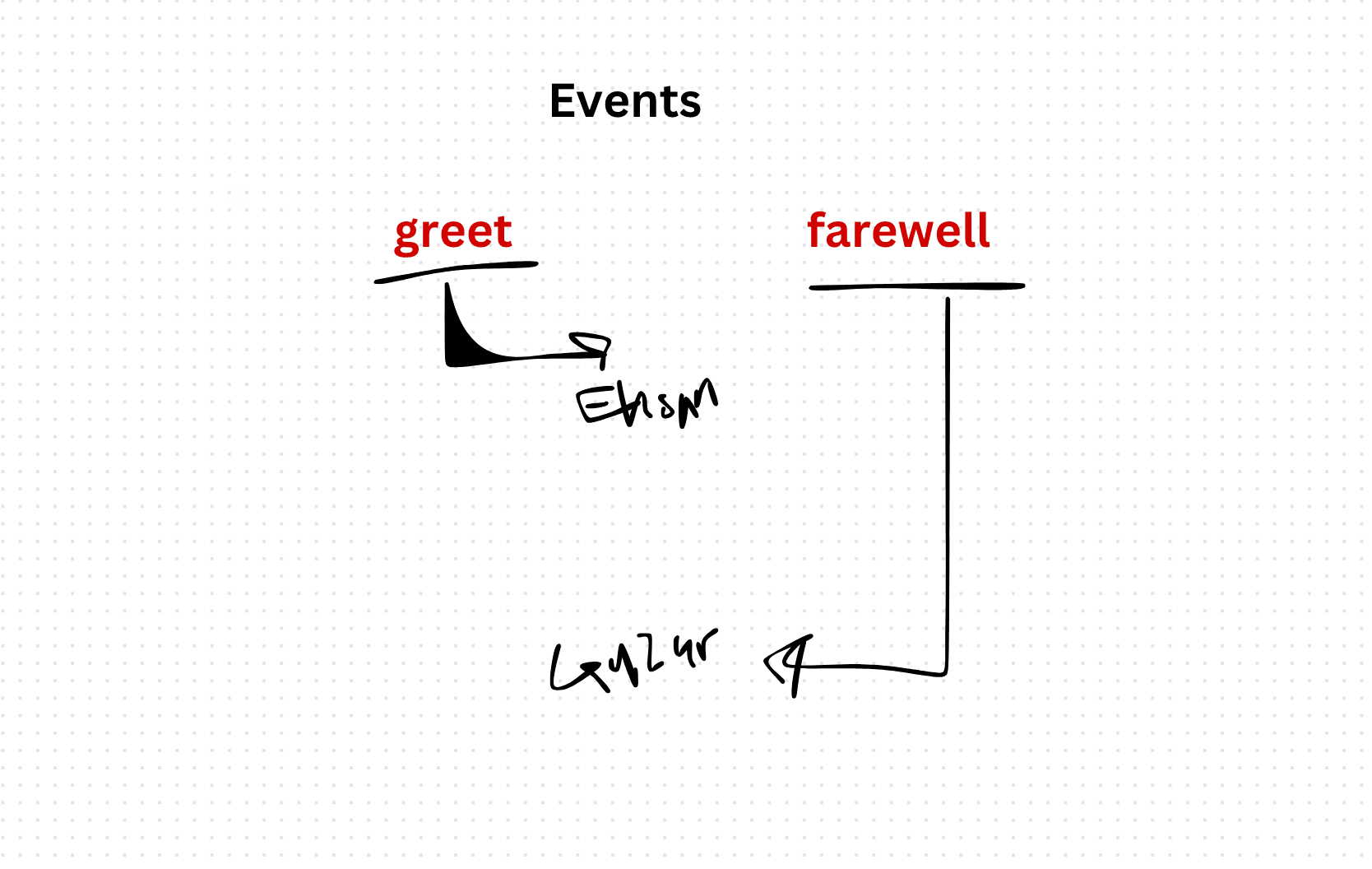
Event-driven programming is a programming paradigm where the flow of the program is determined by events such as user actions, system notifications, or messages from other parts of the application. In an event-driven architecture, components of the application communicate by emitting and listening for events. When an event occurs, the appropriate event handler is invoked to handle the event.
const EventEmitter = require("events");
const eventEmitter = new EventEmitter();
eventEmitter.on("greet", (name) => {
console.log(`Hello, ${name}!`);
});
eventEmitter.on("farewell", (name) => {
console.log(`Goodbye, ${name}!`);
});
// Emit events
eventEmitter.emit("greet", "Ehsan");
eventEmitter.emit("farewell", "Gazar");
In this tutorial, we've explored how to develop an event-driven application using Node.js. We've covered the fundamentals of event-driven programming and demonstrated how to build a simple event-driven app using Node.js and the EventEmitter module. Event-driven architecture allows applications to respond to events asynchronously, making them highly scalable and responsive. By mastering event-driven programming, you can create robust and efficient applications that meet the demands of modern software development. Happy coding!