A Guide to Creating AI Assistants with OpenAI: Unleashing Intelligent Task-Specific Models
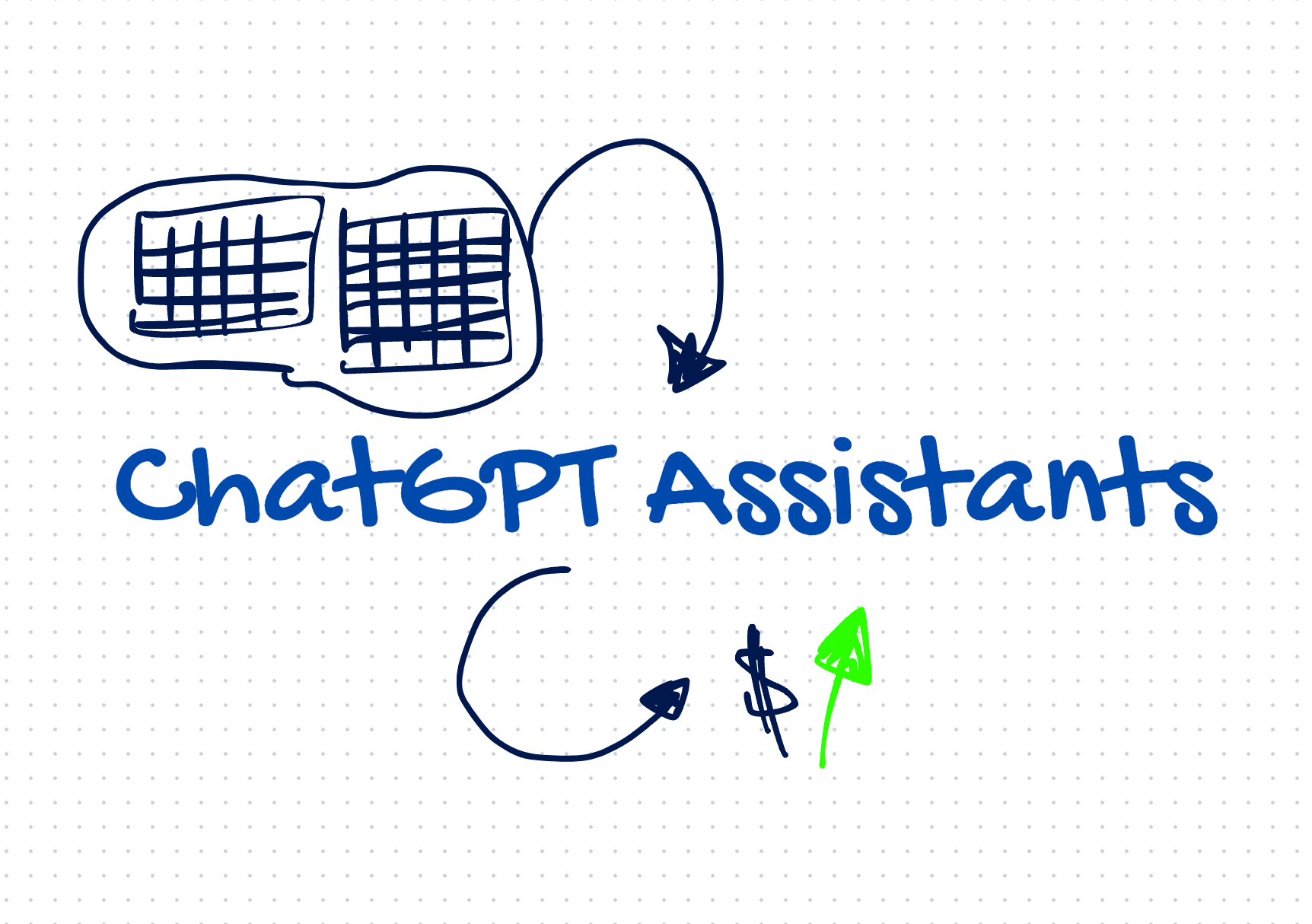
OpenAI has introduced a promising beta feature known as Assistants, which revolves around the concept of creating an assistant with specific characteristics to perform certain tasks. The idea is compelling, but how exactly can one create an AI Assistant? Allow me to guide you through the process based on my exploration of OpenAI's documentation.
First and foremost, OpenAI Assistants are designed to respond to your queries regarding files or any type of media you wish to train the model on. To illustrate this, I decided to leverage the feature for analyzing my bank transactions from CommBank and ING. The goal was to visually map the data and pose inquiries. To achieve this, I opted for Node.js and initiated the process with a succinct syntax.
import OpenAI from "openai";
const openai = new OpenAI();
This snippet served as my starting point, allowing me to interact with OpenAI's Assistants using Node.js.
Afterward, I exported my data in .csv format and utilized the following syntax to import them:
const commbankData = await openai.files.create({
file: fs.createReadStream("./combank.csv"),
purpose: "assistants",
});
const ingData = await openai.files.create({
file: fs.createReadStream("./ing.csv"),
purpose: "assistants",
});
Keep in mind that you only need to perform this step once. Once you obtain the unique ID, store it in a persistent state, such as a JSON file. Subsequently, you can refer to this ID whenever you need to create an assistant.
const assistant = await openai.beta.assistants.create({
name: "Data visualizer",
description:
"You are great at creating beautiful data visualizations. You analyze data present in .csv files, understand trends, and come up with data visualizations relevant to those trends. You also share a brief text summary of the trends observed.",
model: "gpt-4-1106-preview",
tools: [{ type: "code_interpreter" }],
file_ids: [combankData.id, ingData.id],
});
Easy, and now I have my assistant. If you visit their panel at https://platform.openai.com/assistants, you can view the list of assistants you've created. Similarly, in the 'Files' section at https://platform.openai.com/files, you can see the number of files in the system. Don't fret if you lose track during development; you can always rely on the stored IDs.
The subsequent step involves creating a thread
const thread = await openai.beta.threads.create({
messages: [
{
role: "user",
content: "Create data visualizations based on the my expenses in these file.",
file_ids: [combankData.id, ingData.id],
},
],
});
This syntax initiates a thread, encompassing our inaugural message. The ChatGPT model will process this upon executing the command
const run = await openai.beta.threads.runs.create(threadData.id, {
assistant_id: assistantData.id,
});
Subsequently, you have your initial run within the system. However, keep in mind that a run undergoes various states. To monitor the progression and ensure the status transitions to 'completed,' it's essential to retrieve the state periodically.
const run = await openai.beta.threads.runs.retrieve(threadData.id, runData.id);
After this, your initial run is complete. Now, proceed by adding a message to your thread through the following command
const message = await openai.beta.threads.messages.create(threadData.id, {
role: "user",
content: "how much money did I spend last year?",
file_ids: [combankData.id, ingData.id],
});
Once more, execute the command to progress further. This step is crucial to obtain the new command. Additionally, you can review all messages in the thread with the following command:
const messages = await openai.beta.threads.messages.list(threadData.id);
If your run is completed, the final data will encompass the system's response to your message
console.log("messages", messages.data[0].content);
Here's a typical example:
[
{
type: 'text',
text: {
value: 'The total amount of money spent in the year 2023, based on the data from the first file, is approximately \\( \\$89,899.31 \\). Please note that this figure includes all debits from the account and assumes that all negative transactions represent spending. If there are specific types of transactions that should not be counted as spending, this figure would need to be adjusted accordingly.',
annotations: []
}
}
]
Now, with the insights gained, I've realized that I've spent a considerable amount, which wasn't the initial intent of this exercise. A bit regretful! 😅 Nonetheless, it's intriguing how straightforward it is to train and inquire.
Regarding the pricing, the cost incurred for numerous thread retrievals and message exchanges was approximately 0.41 USD, which is reasonable for smaller-scale applications. However, for more extensive use cases, it's advisable to carefully evaluate costs and consider implementing limits.
In conclusion, OpenAI's Assistants beta feature offers a compelling avenue for creating AI with specific characteristics. The journey involves leveraging Node.js, interacting with Assistants, and utilizing unique IDs for assistant creation. The process continues with threading, runs, and messages, allowing seamless interaction with the ChatGPT model.
As demonstrated, the system's responses can provide valuable insights, even if the discoveries might lead to a bit of regret. The ease with which one can train the model and pose inquiries is indeed fascinating.
However, it's crucial to be mindful of costs. While the pricing for smaller-scale applications, including thread retrievals and message exchanges, remains reasonable at around 0.41 USD, thorough evaluation is recommended for more extensive use cases. Implementing limits may also be a prudent strategy.
In essence, exploring OpenAI Assistants opens up a realm of possibilities for creating intelligent, task-specific AI, bringing both simplicity and depth to the development process.