Implementing Queues with TypeScript and Bull.js: A Comprehensive Guide
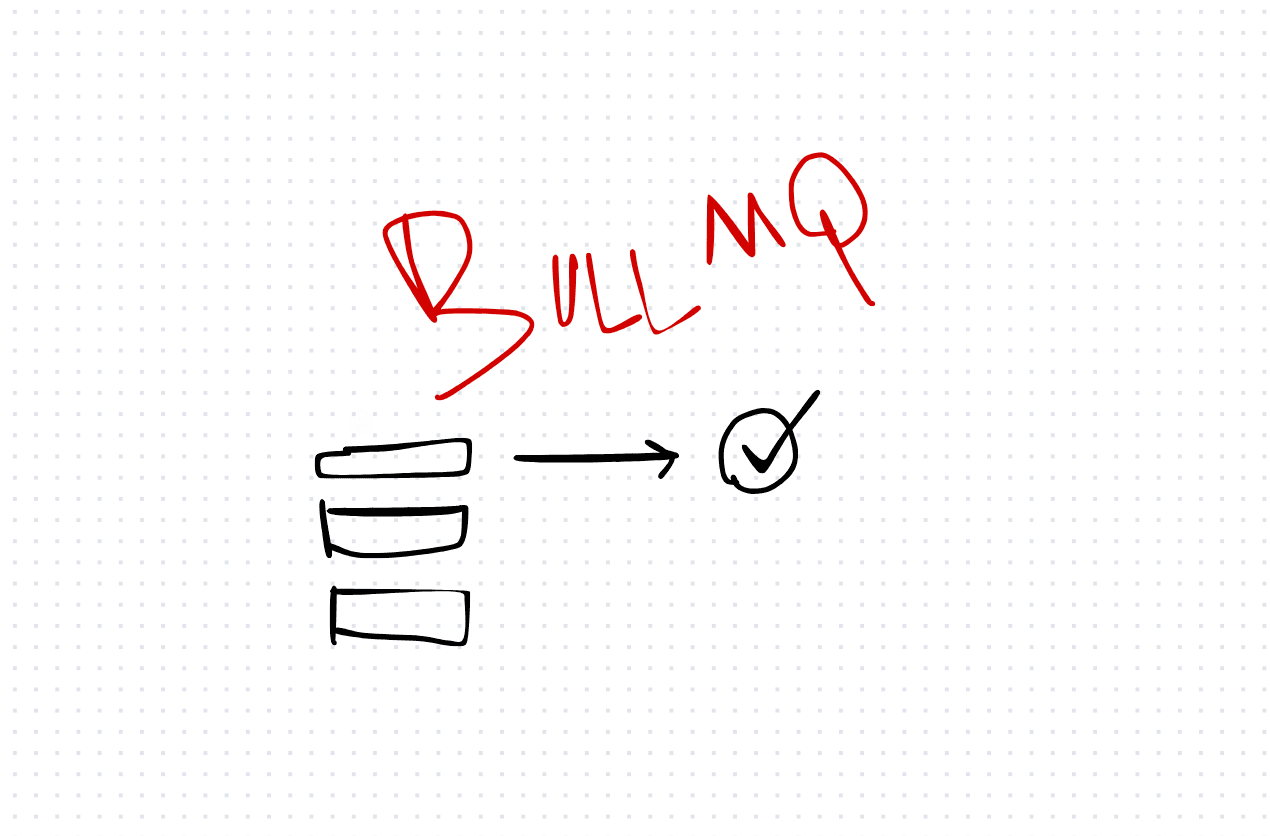
In this technical article, we'll explore how to use Bull.js, a powerful Node.js library for handling queues and background jobs, with TypeScript. We'll cover everything you need to know to implement queues effectively, from installation and basic usage to more advanced features. Throughout the article, we'll provide TypeScript examples to demonstrate each concept and ensure type safety in your codebase.
Bull.js is a Redis-based queue library for Node.js applications. It provides a simple yet robust API for managing queues and processing background jobs asynchronously. With Bull.js, you can easily create, monitor, and process queues, making it ideal for implementing features like job scheduling, task processing, and message queuing in your applications.
import { Queue, Worker } from "bullmq";
const myQueue = new Queue("my-queue", {
connection: {
host: "localhost",
port: 6379,
},
});
const myWorker = new Worker(
"my-queue",
async (job) => {
console.log("data", job.data);
},
{
connection: {
host: "localhost",
port: 6379,
},
}
);
myWorker.on("completed", (job) => {
console.log(`${job.id} has completed!`);
});
myWorker.on("failed", (job, err) => {
if (!job) return;
console.log(`${job.id} has failed with ${err.message}`);
});
const addJobs = async (indexNumber: number) => {
if (indexNumber === 10) {
return;
}
await new Promise((resolve) => {
setTimeout(() => {
myQueue.add(`Job ${indexNumber}`, {
data: `Data for job ${indexNumber}`,
});
addJobs(indexNumber + 1);
resolve("DONE");
}, 1000);
});
};
addJobs(1);
export { myQueue, myWorker };
In this article, we've learned how to use Bull.js to implement queues in a TypeScript project. We covered creating queues, enqueuing jobs, and processing jobs using Bull.js. By following these examples, you can leverage the power of Bull.js to build robust and scalable applications with background job processing capabilities.