How Do You Implement a React Portal in a React Application?
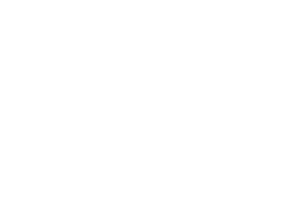
React portals enable rendering children components into a DOM node that is outside the parent component's DOM hierarchy. This allows developers to render content outside the usual flow of the DOM, making it useful for scenarios like modals, tooltips, and popovers.
To implement a React portal in a TypeScript React application, follow these steps:
- Create a Portal Component: First, create a component that will serve as the portal. This component will render its children into a separate DOM node.
- Render Portal Using ReactDOM.createPortal(): Use the ReactDOM.createPortal() method to render the portal component's children into a specific DOM node.
import React from 'react';
import ReactDOM from 'react-dom';
interface ModalProps {
onClose: () => void;
}
const Modal: React.FC<ModalProps> = ({ children, onClose }) => {
return ReactDOM.createPortal(
<div className="modal-overlay">
<div className="modal">
<button className="close-btn" onClick={onClose}>Close</button>
{children}
</div>
</div>,
document.getElementById('modal-root')!
);
};
export default Modal;
And then you can use it like this:
import React, { useState } from 'react';
import Modal from './Modal';
const App: React.FC = () => {
const [showModal, setShowModal] = useState(false);
const toggleModal = () => {
setShowModal(!showModal);
};
return (
<div>
<button onClick={toggleModal}>Open Modal</button>
{showModal && (
<Modal onClose={toggleModal}>
<h2>Modal Content</h2>
<p>This is the content of the modal.</p>
</Modal>
)}
</div>
);
};
export default App;
In TypeScript React applications, implementing portals provides a powerful mechanism for rendering content outside the usual DOM hierarchy. By using ReactDOM.createPortal(), developers can create flexible and reusable components like modals, tooltips, and overlays. Understanding and leveraging React portals enable developers to build more dynamic and interactive user interfaces with ease.