React Refs and Callback Refs
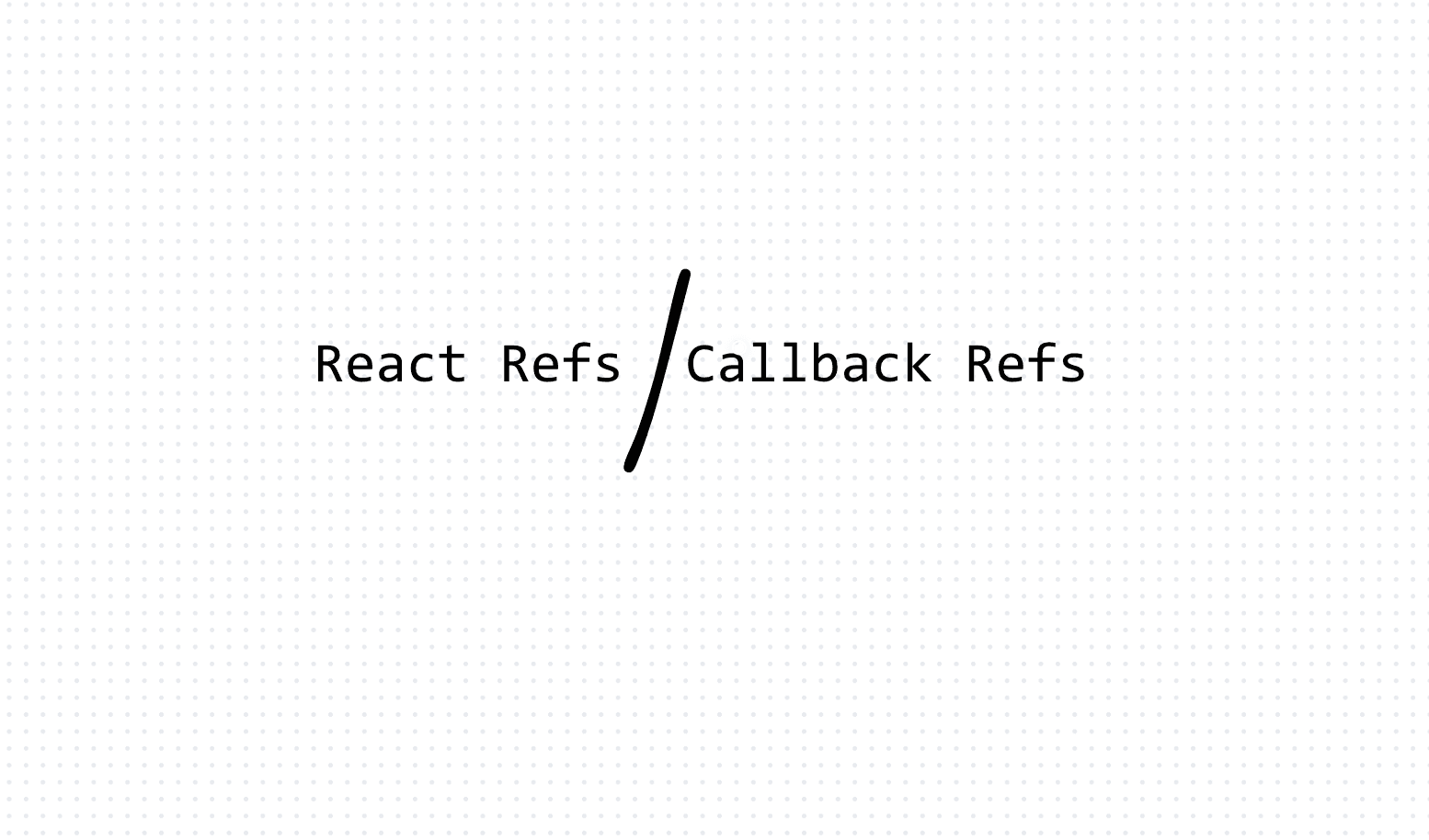
React refs are objects created using the React.createRef() method, allowing developers to reference DOM elements or React components. Refs provide a way to access and manipulate DOM elements directly, such as focusing an input or measuring its dimensions.
import React, { useRef } from 'react';
const MyComponent: React.FC = () => {
const inputRef = useRef<HTMLInputElement>(null);
const focusInput = () => {
if (inputRef.current) {
inputRef.current.focus();
}
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={focusInput}>Focus Input</button>
</div>
);
};
Callback refs are a function-based approach for managing refs in React. Instead of creating refs using React.createRef(), developers pass a callback function to the ref attribute of a JSX element. This callback function receives the DOM element or component instance as an argument, allowing for custom logic to be executed.
To implement a callback ref in a TypeScript React application, define a callback function and pass it to the ref attribute of a JSX element. Here's an example:
import React, { useRef } from 'react';
const MyComponent: React.FC = () => {
const inputRef = useRef<HTMLInputElement>(null);
const setInputRef = (element: HTMLInputElement | null) => {
if (element) {
element.focus();
}
};
return (
<div>
<input ref={setInputRef} type="text" />
</div>
);
};
Difference between React Refs and Callback Refs
The main difference between React refs and callback refs lies in their implementation and usage:
- React Refs: React refs are objects created using React.createRef(), providing a direct reference to DOM elements or React components.
- Callback Refs: Callback refs are function-based approaches where a callback function is passed to the ref attribute, allowing for custom logic to be executed when the ref is set.
In TypeScript React applications, managing refs is essential for interacting with DOM elements and React components. While React refs provide a straightforward approach for referencing elements, callback refs offer flexibility for executing custom logic when setting refs. By understanding and utilizing both techniques effectively, developers can enhance the interactivity and functionality of their React applications.