Naming Conventions in TypeScript: Best Practices for Classes, Functions, Types, Interfaces, Variables and Parameters
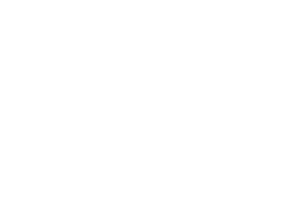
Choosing appropriate names for classes, functions, and parameters is crucial for writing clean, maintainable code in TypeScript. In this article, we'll delve into the best practices and naming conventions for each of these elements, accompanied by practical examples in TypeScript. By following these guidelines, developers can enhance code readability, facilitate collaboration, and foster consistency across projects.
Naming Classes:
Classes represent blueprints for objects in TypeScript, and naming them appropriately is essential for conveying their purpose and role in the system. Here are some best practices for naming classes:
- Use nouns or noun phrases to describe what the class represents.
- Prefer descriptive names that indicate the class's responsibility or domain.
- Use PascalCase (capitalize the first letter of each word) for class names.
class ProductService {}
Naming Functions:
Functions encapsulate specific behavior or operations within a program. Clear and descriptive function names contribute to code readability and understanding. Consider the following guidelines when naming functions:
- Use verbs or verb phrases to describe the action performed by the function.
- Choose descriptive names that convey the function's purpose and expected behavior.
- Prefer camelCase (start with a lowercase letter and capitalize subsequent words) for function names.
function calculateTotalPrice(items: Item[]): number {
}
Naming Parameters:
Parameters are inputs provided to functions or methods to perform computations or operations. Well-named parameters enhance code clarity and improve readability. Follow these principles when naming function parameters:
- Use descriptive names that convey the purpose or role of the parameter within the function.
- Avoid single-letter or ambiguous names; opt for meaningful names that provide context.
- Maintain consistency with naming conventions used for variables and identifiers.
Naming Types:
Types define custom data structures and are used to describe the shape of objects in TypeScript. Clear and descriptive type names contribute to code clarity and understanding. Consider the following principles when naming types:
- Use nouns or noun phrases to describe the entity or concept represented by the type.
- Prefer descriptive names that accurately convey the purpose and semantics of the type.
- Use PascalCase for type names to distinguish them from variables and interfaces.
- There are two approaches to use IProduct (I+Name) for interfaces and ProductType if you want to define a type
interface IProduct = {
id: number;
name: string;
price: number;
};
type ProductCategroyType = "Digital" | "Non-Digital"
Naming Variables:
Variables represent storage locations for data values and are integral to writing expressive and understandable code. Meaningful variable names improve code readability and maintainability. Consider the following principles for naming variables:
- Use descriptive names that convey the purpose or meaning of the stored value.
- Avoid single-letter or cryptic names; opt for meaningful names that provide context.
- Follow camelCase for variable names to maintain consistency with JavaScript and TypeScript conventions.
const totalPrice: number = calculateTotalPrice(items);
Conclusion:
Adhering to consistent and meaningful naming conventions for classes, functions, and parameters is vital for producing clean, understandable code in TypeScript. By following the best practices outlined in this article, developers can create codebases that are easier to comprehend, maintain, and extend. Remember, clarity and consistency in naming contribute significantly to the overall quality of software projects.