Decoupling Functions and Methods: Best Practices in TypeScript
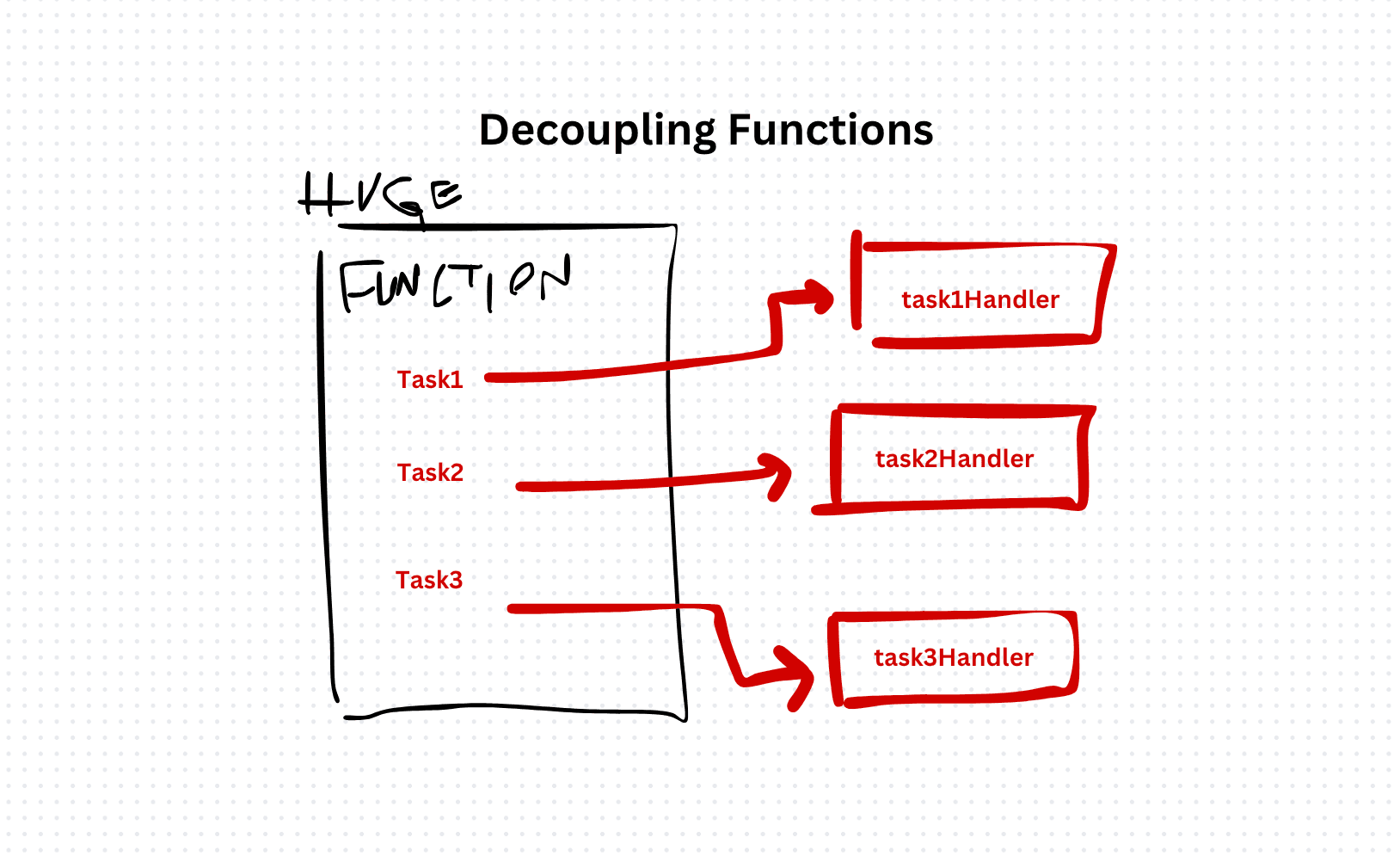
In software development, decoupling larger functions and methods is essential for improving code maintainability, readability, and testability. Decoupling allows breaking down complex logic into smaller, reusable units, making it easier to understand and modify. In this article, we'll explore the importance of decoupling, best practices for achieving it in TypeScript, and provide practical examples to demonstrate these concepts.
Decoupling is the process of reducing interdependencies between different parts of a system. It promotes modularity, flexibility, and easier code maintenance. By decoupling functions and methods, developers can isolate concerns, making it easier to change one part of the code without affecting others. This leads to cleaner, more maintainable codebases.
Example: Decoupling with TypeScript
function calculateOrderTotal(items: Item[], taxRate: number): number {
let subtotal = 0;
for (const item of items) {
subtotal += item.price * item.quantity;
}
const tax = subtotal * taxRate;
return subtotal + tax;
}
Decoupled Function with Dependency Injection
function calculateSubtotal(items: Item[]): number {
let subtotal = 0;
for (const item of items) {
subtotal += item.price * item.quantity;
}
return subtotal;
}
function calculateTax(subtotal: number, taxRate: number): number {
return subtotal * taxRate;
}
function calculateOrderTotal(items: Item[], taxRate: number): number {
const subtotal = calculateSubtotal(items);
const tax = calculateTax(subtotal, taxRate);
return subtotal + tax;
}
Decoupling functions and methods is crucial for building maintainable, flexible software systems. By following best practices such as the Single Responsibility Principle, Dependency Injection, and embracing functional programming principles, developers can create modular, reusable code that is easier to understand, test, and maintain. In TypeScript, these principles can be applied effectively to improve code quality and promote codebase longevity.