Embracing the DRY Principle: Best Practices for Don't Repeat Yourself in Programming
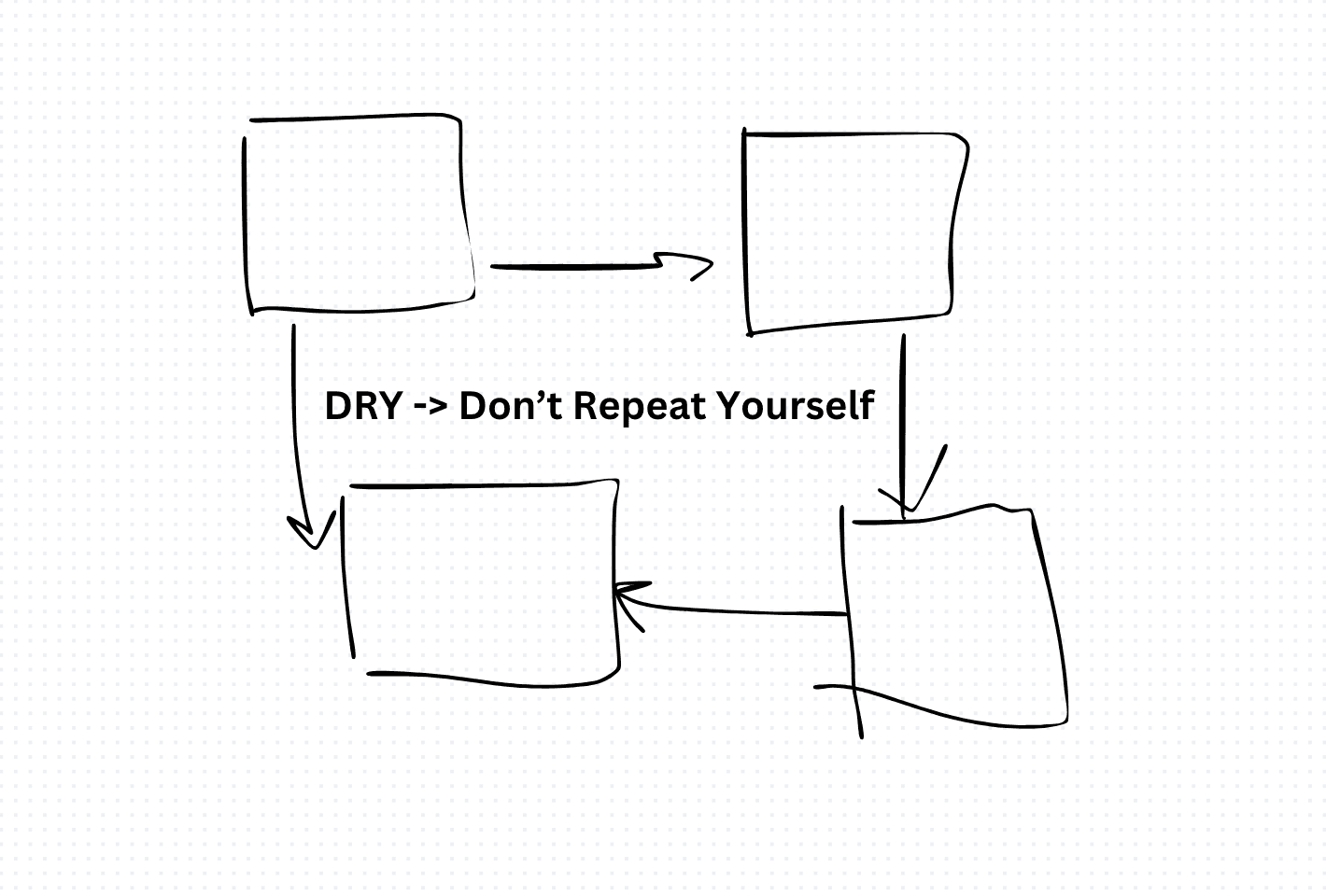
The DRY (Don't Repeat Yourself) principle is a fundamental concept in software development that emphasizes code reusability and maintainability. In this technical article, we'll delve into the DRY principle, discuss its importance in writing clean and efficient code, and provide practical strategies and examples for applying it effectively in programming.
The DRY principle states that every piece of knowledge or logic in a software system should have a single, unambiguous representation. In other words, code duplication should be avoided whenever possible, and common functionality should be abstracted into reusable components.
Best Practices for Applying the DRY Principle:
- Identify Common Patterns: Look for patterns or recurring themes in your codebase that indicate opportunities for abstraction and reuse. Common functionality such as input validation, error handling, and data manipulation are prime candidates for abstraction.
- Extract Reusable Components: Once common patterns are identified, extract them into reusable components, functions, or modules. These components should encapsulate well-defined functionality and be designed with a clear interface that promotes reusability.
- Parameterization and Generalization: Make your reusable components more flexible and adaptable by parameterizing them and making them more general-purpose. Avoid hardcoding specific values or assumptions that limit their applicability.
- Create Libraries and Utilities: Consider creating libraries or utility modules to encapsulate commonly used functionality that can be shared across multiple projects or teams. These libraries can serve as a centralized repository of reusable code.
- Refactor Existing Code: Regularly review your codebase for instances of duplication and refactor them to adhere to the DRY principle. Refactoring tools and code analysis can help identify areas where code can be consolidated or abstracted.
- Documentation and Communication: Document your reusable components and communicate their existence and purpose to other developers on your team. Clear documentation helps promote code reuse and ensures that developers are aware of existing solutions before reinventing the wheel.
function calculateAreaOfRectangle(width, height) {
return width * height;
}
function calculatePerimeterOfRectangle(width, height) {
return 2 * (width + height);
}
// Refactored code
function calculateRectangleProperties(width, height, operation) {
switch (operation) {
case 'area':
return width * height;
case 'perimeter':
return 2 * (width + height);
default:
throw new Error('Invalid operation');
}
}
The DRY principle is a cornerstone of software development that promotes code reusability, maintainability, and scalability. By identifying common patterns, extracting reusable components, and fostering a culture of code reuse, developers can create cleaner, more efficient codebases that are easier to maintain and extend over time. Embrace the DRY principle in your programming practices to write better code and become a more effective developer.