KISS Principle: Keep It Simple, Stupid
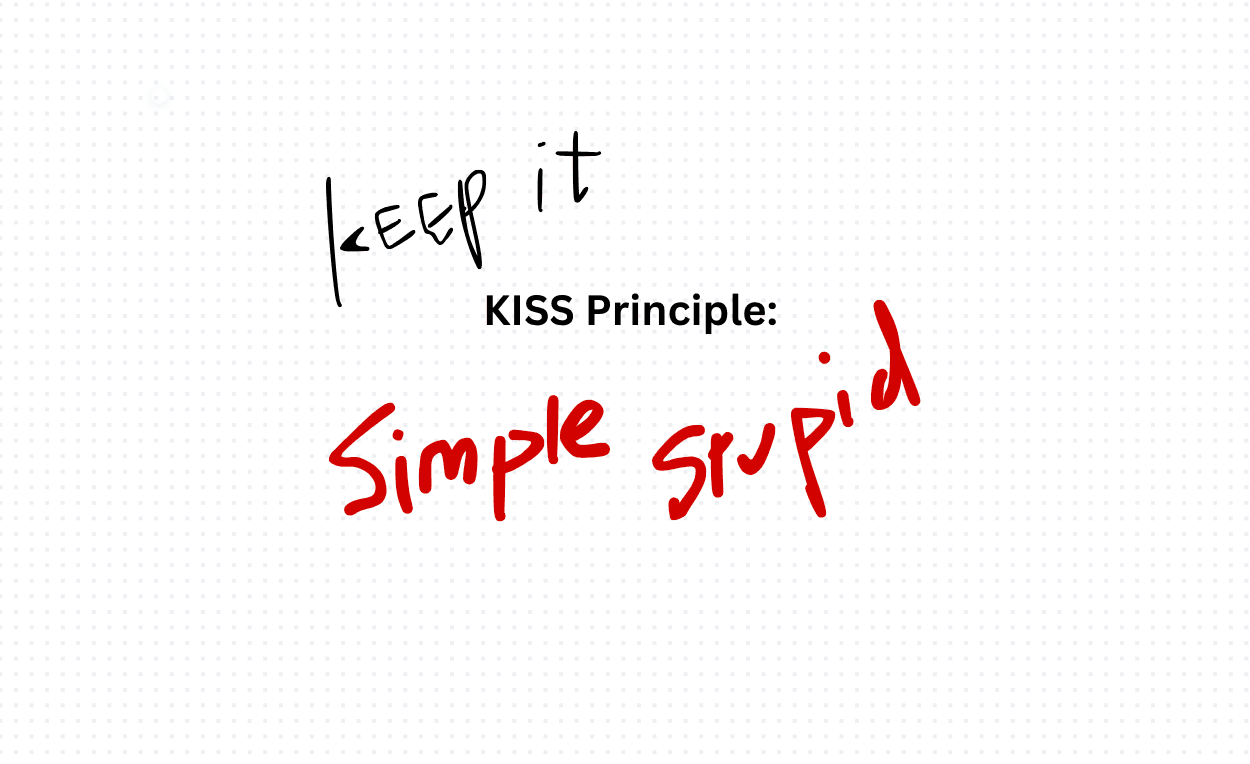
The KISS principle encourages developers to favor simple solutions over complex ones. It emphasizes the importance of clarity, readability, and maintainability in code by discouraging unnecessary complexity, abstraction, or over-engineering. The essence of the KISS principle is to strive for simplicity in design without sacrificing functionality or robustness.
Importance of the KISS Principle:
- Readability: Simple code is easier to understand and reason about, making it more accessible to other developers and facilitating collaboration.
- Maintainability: Simple solutions are typically easier to maintain and debug compared to complex ones. They have fewer moving parts and dependencies, reducing the risk of unintended consequences or bugs.
- Scalability: Simple designs are more adaptable to change and evolution over time. They provide a solid foundation for future enhancements and modifications without introducing unnecessary complexity.
- Reduced Cognitive Load: Complex code can overwhelm developers and hinder productivity. By keeping solutions simple, developers can focus on solving the problem at hand rather than grappling with unnecessary complexity.
- Faster Development: Simple designs often lead to faster development cycles, as developers spend less time grappling with complex architectures or intricate implementations.
// Complex solution
function calculateFibonacci(n: number): number[] {
if (n <= 0) return [];
if (n === 1) return [0];
if (n === 2) return [0, 1];
const fibonacciSequence: number[] = [0, 1];
while (fibonacciSequence.length < n) {
const nextFibonacciNumber =
fibonacciSequence[fibonacciSequence.length - 1] +
fibonacciSequence[fibonacciSequence.length - 2];
fibonacciSequence.push(nextFibonacciNumber);
}
return fibonacciSequence;
}
// Simple solution
function calculateFibonacci(n: number): number[] {
const fibonacciSequence: number[] = [0, 1];
while (fibonacciSequence.length < n) {
fibonacciSequence.push(
fibonacciSequence[fibonacciSequence.length - 1] +
fibonacciSequence[fibonacciSequence.length - 2]
);
}
return fibonacciSequence.slice(0, n);
}
OR Even better
function fibonacci(n: number): number {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
The KISS principle is a guiding philosophy for TypeScript developers seeking to write clean, maintainable, and scalable code. By prioritizing simplicity and clarity, TypeScript developers can create codebases that are easier to understand, maintain, and extend over time. Incorporate the KISS principle into your TypeScript development practices to streamline your codebase, enhance collaboration, and accelerate development cycles.