Exploring Matrix Data Structure in TypeScript
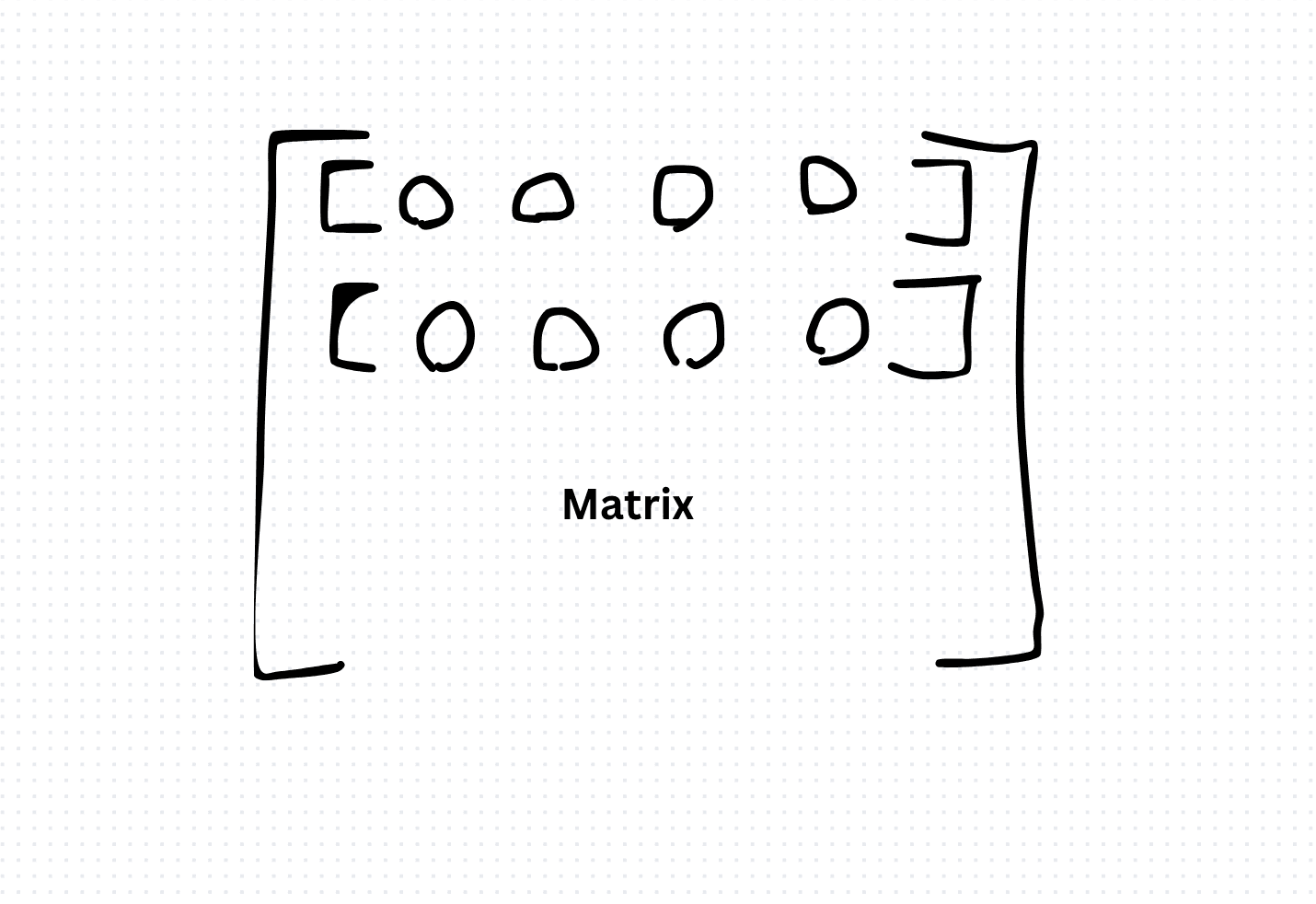
A matrix is a two-dimensional array of numbers arranged in rows and columns. In TypeScript, we can represent a matrix using a nested array, where each inner array represents a row of the matrix.
type Matrix = number[][];
const matrix: Matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
Matrix Addition:
To add two matrices, we add corresponding elements from each matrix.
function addMatrices(matrix1: Matrix, matrix2: Matrix): Matrix {
const result: Matrix = [];
for (let i = 0; i < matrix1.length; i++) {
const row: number[] = [];
for (let j = 0; j < matrix1[i].length; j++) {
row.push(matrix1[i][j] + matrix2[i][j]);
}
result.push(row);
}
return result;
}
When to use Matrices?
Matrices are commonly used in various scenarios where data is organized in a two-dimensional format, and operations such as addition, multiplication, and transformation are required. Here are some specific situations where matrices are commonly used:
- Computer Graphics: Matrices play a crucial role in computer graphics for representing transformations such as translation, rotation, scaling, and projection. They are used to manipulate the positions of vertices in 2D and 3D space to render images.
- Image Processing: In image processing applications, matrices are used to represent images, where each element corresponds to a pixel value. Matrices are employed for operations such as convolution, filtering, and transformations to enhance or manipulate images.
- Machine Learning: Matrices are fundamental to machine learning algorithms, especially in tasks like linear regression, classification, and dimensionality reduction. Features of data samples are often represented as rows in matrices, and operations such as dot products and matrix multiplication are used extensively.
- Numerical Analysis: Matrices are used in numerical analysis for solving systems of linear equations, eigenvalue problems, and differential equations. Techniques like Gaussian elimination and LU decomposition rely on matrix manipulation.
- Physics and Engineering: Matrices are used to represent physical quantities and equations in fields like mechanics, electromagnetics, and signal processing. They enable the modeling and simulation of complex systems and phenomena.
- Optimization and Operations Research: Matrices are used to model optimization problems, such as linear programming, network flow, and resource allocation. They help in representing constraints and objective functions in a concise format.
The matrix data structure is a powerful tool used in various computational tasks, from computer graphics to scientific computing. By understanding its representation and common operations, developers can leverage matrices to solve a wide range of problems efficiently in TypeScript applications. Whether it's performing geometric transformations or solving complex equations, matrices provide a versatile and flexible framework for mathematical computations.