Exploring Primitive Data Structures, Number, String, Boolean, Null and Undefined in TypeScript
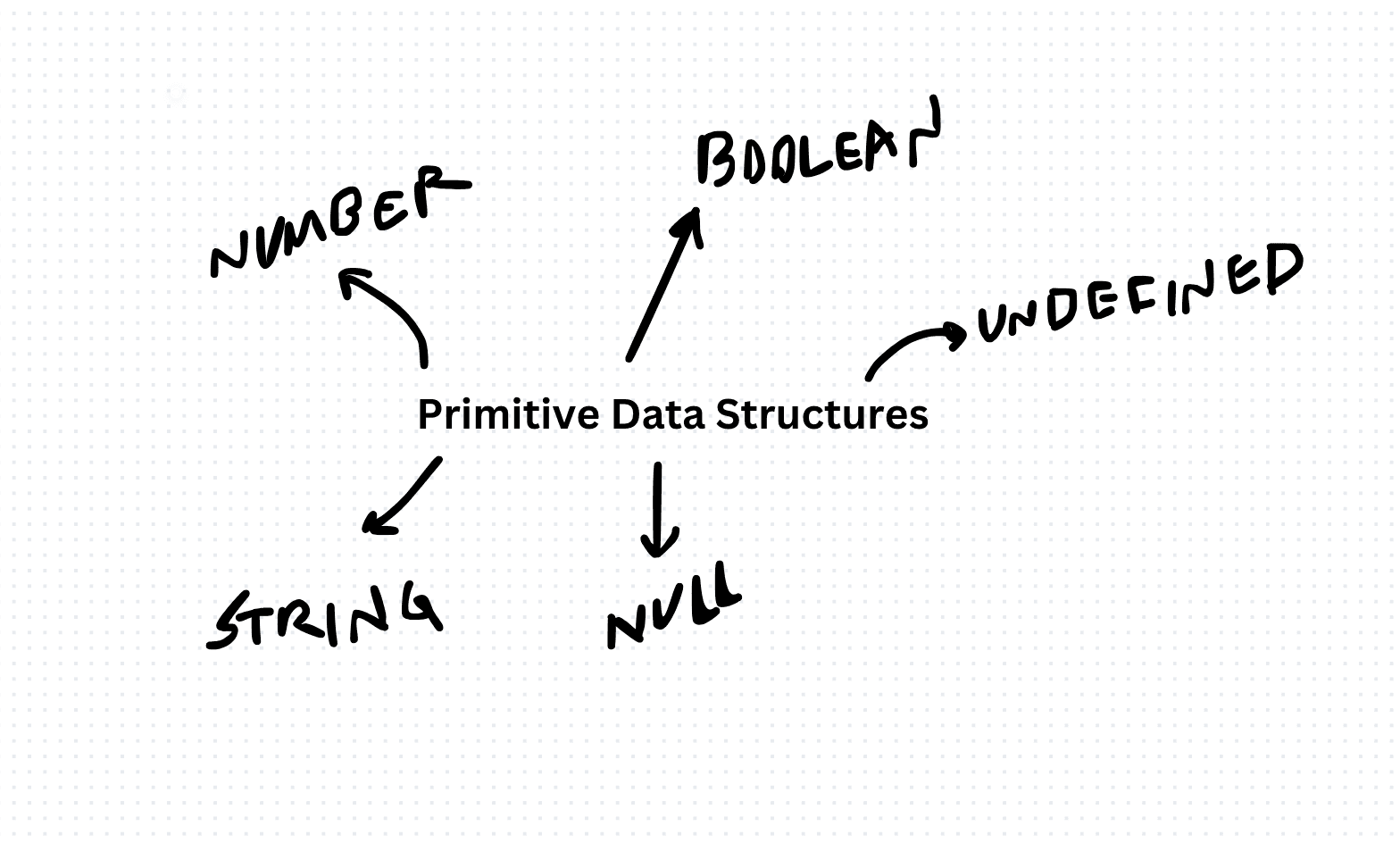
Primitive data structures form the foundation of any programming language, providing fundamental building blocks for storing and manipulating data. In TypeScript, primitive data types include numbers, strings, booleans, null, and undefined. Understanding these primitive data structures and how to work with them is essential for every TypeScript developer.
Number:
The number data type in TypeScript represents both integer and floating-point numbers. Here's how you can use numbers in TypeScript:
const age: number = 30;
const height: number = 5.9;
String:
Strings are used to represent textual data in TypeScript. They can be declared using single or double quotes:
const message: string = "Hello, TypeScript!";
const name: string = 'John Doe';
Boolean:
Booleans represent true or false values. They are commonly used for conditional statements and logical operations:
const isActive: boolean = true;
const isLoggedOut: boolean = false;
Null and Undefined:
Null and undefined are used to represent the absence of value. Null is explicitly assigned to a variable, while undefined typically indicates that a variable has not been initialized:
let firstName: string | null = "John";
let lastName: string | undefined;
Understanding primitive data structures is fundamental to writing efficient and bug-free TypeScript code. By mastering the usage of numbers, strings, booleans, null, and undefined, developers can build robust and reliable applications. With the code examples provided in this article, you're now equipped to leverage primitive data structures effectively in your TypeScript projects!