Exploring Queue Data Structure in TypeScript: Implementation and Applications
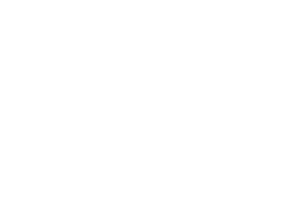
A queue is a linear data structure that stores a collection of elements with two primary operations: enqueue and dequeue. The enqueue operation adds an element to the rear of the queue, while the dequeue operation removes and returns the front element of the queue. Additionally, queues typically support other operations such as peek (retrieve the front element without removing it) and isEmpty (check if the queue is empty).
class Queue<T> {
private items: T[];
constructor() {
this.items = [];
}
enqueue(item: T): void {
this.items.push(item);
}
dequeue(): T | undefined {
return this.items.shift();
}
peek(): T | undefined {
return this.items[0];
}
isEmpty(): boolean {
return this.items.length === 0;
}
size(): number {
return this.items.length;
}
clear(): void {
this.items = [];
}
}
Applications of Queues:
- Task Scheduling: Queues are commonly used to manage tasks and processes in computer systems. For example, an operating system's task scheduler may use a queue to prioritize and execute tasks based on their arrival time or priority level.
- Breadth-First Search (BFS): Queues play a crucial role in graph traversal algorithms such as BFS. In BFS, nodes are explored level by level, with each level represented by a queue of nodes. This approach ensures that nodes are visited in the order of their distance from the starting node.
- Message Queues: Queues are fundamental components of message-oriented middleware systems used in distributed computing environments. Message queues enable asynchronous communication between different parts of a system, allowing for reliable and scalable message processing.
- Print Queue: In computer systems, print queues are used to manage print jobs sent to printers. Print requests are added to the queue and processed in the order they were received, ensuring fair access to the printing resources.
Conclusion:
Queues are versatile data structures with a wide range of applications in computer science, software engineering, and everyday life. By understanding how queues work and leveraging their FIFO nature, developers can design efficient and elegant solutions to various problems.