Exploring Trie Data Structure in TypeScript: Implementation and Applications
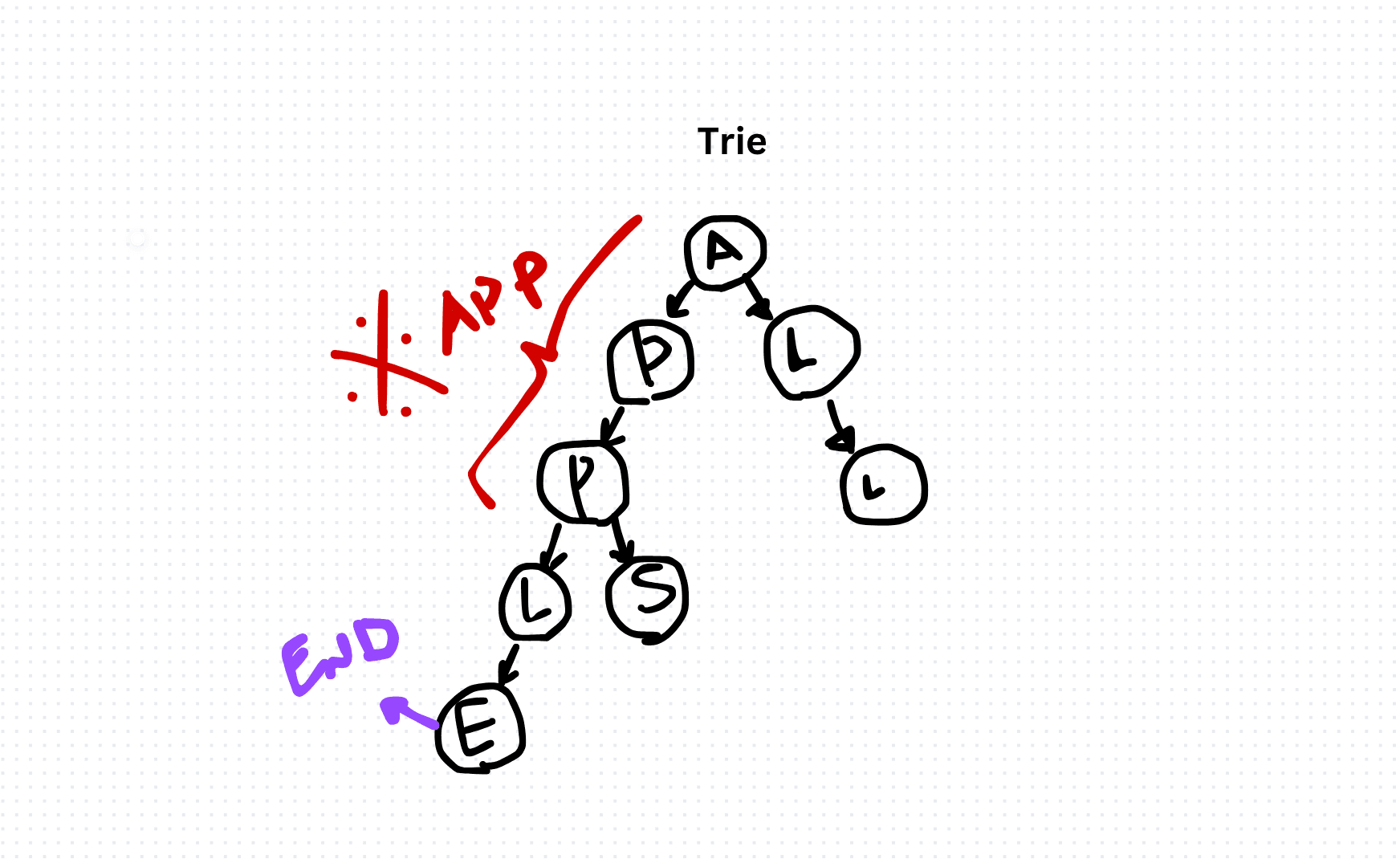
Trie is a tree-like data structure that stores a dynamic set of strings where the keys can be traversed in a prefix search manner. Each node of the Trie represents a single character of the string, and the path from the root to a node spells out a particular string. Trie enables fast searching, insertion, and deletion of strings, making it suitable for tasks like autocomplete, spell checking, and dictionary implementations.
Implementation in TypeScript:
Below is a TypeScript implementation of a basic Trie data structure:
class TrieNode {
children: Map<string, TrieNode>;
isEndOfWord: boolean;
constructor() {
this.children = new Map();
this.isEndOfWord = false;
}
}
class Trie {
root: TrieNode;
constructor() {
this.root = new TrieNode();
}
insert(word: string): void {
let node = this.root;
for (let char of word) {
if (!node.children.has(char)) {
node.children.set(char, new TrieNode());
}
node = node.children.get(char)!;
}
node.isEndOfWord = true;
}
search(word: string): boolean {
let node = this.root;
for (let char of word) {
if (!node.children.has(char)) {
return false;
}
node = node.children.get(char)!;
}
return node.isEndOfWord;
}
}
// Example usage
const trie = new Trie();
trie.insert("apple");
console.log(trie.search("apple")); // Output: true
console.log(trie.search("app")); // Output: false
(Source: https://gazar.dev/system-design/how-to-implement-auto-suggestion-system-design)
Applications of Trie:
- Autocomplete: Trie data structure is commonly used in autocomplete systems to suggest possible word completions as users type.
- Spell Checking: Trie can efficiently check whether a given word exists in a dictionary or not, making it suitable for spell-checking applications.
- IP Routing: Trie can be used in networking algorithms like IP routing to store and search for IP addresses efficiently.
- Text Indexing: Trie is used in text indexing applications to quickly search for strings in large documents or datasets.
Conclusion:
Trie data structure offers efficient string search, insertion, and deletion operations, making it a valuable tool for various applications such as autocomplete, spell checking, and text indexing. By understanding the principles of Trie and its implementation in TypeScript, developers can leverage its capabilities to build fast and scalable solutions for string-related tasks.