Exploring Tree Data Structure in TypeScript
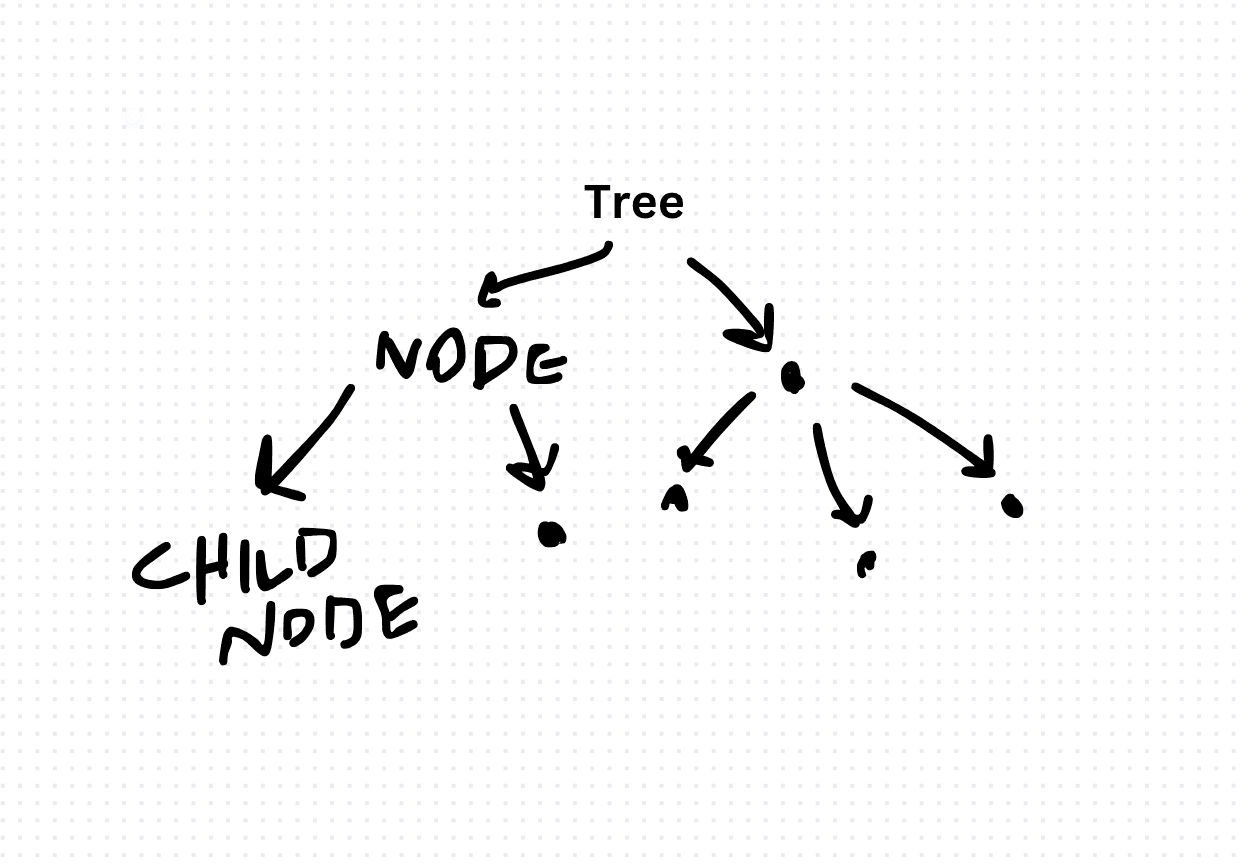
Trees are hierarchical data structures that consist of nodes connected by edges. They are widely used in computer science for organizing and representing hierarchical relationships between data. In TypeScript, we can implement tree data structures to store and manipulate hierarchical data efficiently. In this article, we'll delve into understanding tree data structures in TypeScript, exploring their characteristics, operations, and implementation with code examples.
Node Class Implementation:
To represent nodes in a tree, we'll create a Node class that contains data and references to its child nodes.
class Node<T> {
data: T;
children: Node<T>[];
constructor(data: T) {
this.data = data;
this.children = [];
}
addChild(child: Node<T>): void {
this.children.push(child);
}
}
Creating a Tree:
With the Node class in place, we can construct a tree by adding nodes and defining relationships between them.
// Create nodes
let rootNode = new Node<string>('Root');
let child1 = new Node<string>('Child 1');
let child2 = new Node<string>('Child 2');
let subChild = new Node<string>('Sub Child');
// Add child nodes
rootNode.addChild(child1);
rootNode.addChild(child2);
child1.addChild(subChild);
Tree traversal is the process of visiting all the nodes in a tree in a specific order. We can implement depth-first and breadth-first traversal algorithms recursively or iteratively.
function preOrderTraversal(node: Node<string>): void {
console.log(node.data);
node.children.forEach(child => preOrderTraversal(child));
}
preOrderTraversal(rootNode);
OR
function breadthFirstTraversal(root: Node<string>): void {
let queue: Node<string>[] = [root];
while (queue.length > 0) {
let node = queue.shift()!;
console.log(node.data);
node.children.forEach(child => queue.push(child));
}
}
breadthFirstTraversal(rootNode);
(Source: https://gazar.dev/algorithm/tree-traversal-algorithms)
Trees support various operations such as searching for a node, removing a node, finding the height of the tree, and more. These operations can be implemented recursively or iteratively depending on the requirement.
When to use it?
- File Systems: Trees are commonly used to represent file systems, where directories (folders) can contain subdirectories and files. Each directory node can have multiple children, representing its subdirectories and files.
- Organization Charts: Trees can represent hierarchical structures in organizations, where each node represents an employee or a department, and the edges represent the reporting relationships between them.
- HTML DOM: The Document Object Model (DOM) in web development is represented as a tree structure, where each HTML element is a node, and elements can have child elements (nested structure).
- Database Indexing: Trees, such as Binary Search Trees (BSTs) or B-Trees, are used in database indexing to efficiently search, insert, and delete records. They provide fast retrieval of data in sorted order. (Source: https://gazar.dev/algorithm/binary-search-trees-algorithm)
- Routing Algorithms: Trees are used in network routing algorithms to determine the best path for data packets to travel through a network. Routing tables are often represented as trees to efficiently route traffic.
- Decision Trees: In machine learning and data mining, decision trees are used to model decisions based on a set of conditions. Each node represents a decision based on a feature, and the branches represent possible outcomes.
Tree data structures play a vital role in organizing hierarchical data and are widely used in various applications ranging from file systems to database indexing. In TypeScript, implementing tree data structures allows us to efficiently manage hierarchical relationships between data elements. By understanding the characteristics, operations, and implementation of tree data structures, developers can leverage trees effectively in their TypeScript projects, enabling efficient data management and processing.