Session Storage: Limitations and TypeScript Usage
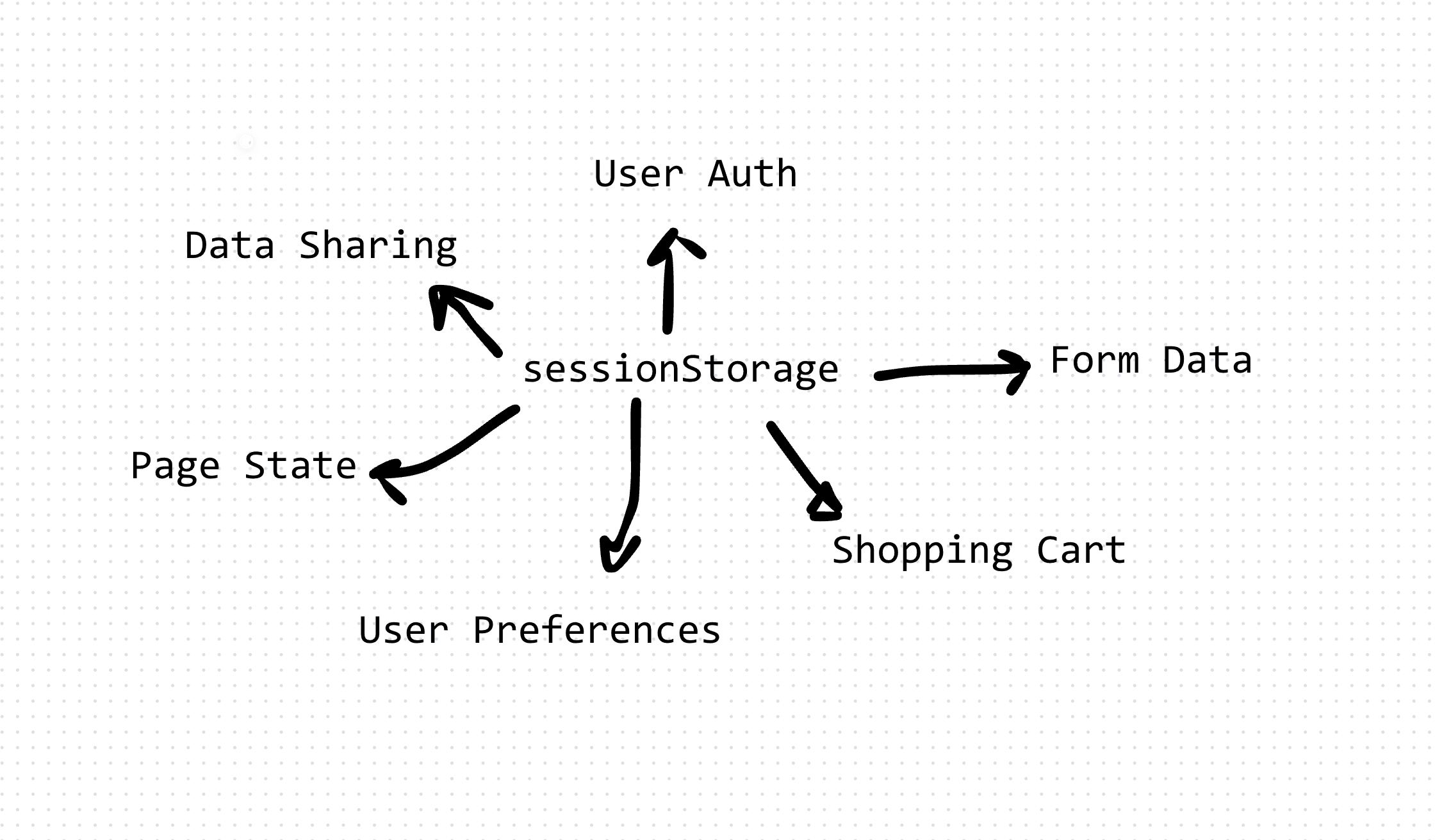
Session storage is a valuable tool for web developers, allowing them to store data locally within the user's browser session. While it offers convenience and simplicity, it's essential to understand its limitations to use it effectively. In this article, we'll explore the constraints of session storage and demonstrate how to leverage it in TypeScript applications with practical examples.
Understanding Session Storage Limitations:
Session storage is limited in several ways:
- Session Scope: Data stored in session storage is only available for the duration of the browser session. Once the session ends (e.g., when the user closes the tab or browser), the data is lost.
- Storage Capacity: Session storage has a limited storage capacity compared to other storage mechanisms like local storage. Browsers typically allocate around 5-10 MB of storage per origin for session storage.
- String-only Data: Session storage can only store data in string format. Complex data types like objects or arrays need to be serialized into strings before storage and deserialized upon retrieval.
- Security Restrictions: Session storage is subject to the same-origin policy, limiting access to data stored by other origins.
Using Session Storage in TypeScript:
Here are a few examples demonstrating how to use session storage in TypeScript:
sessionStorage.setItem('username', 'JohnDoe');
const username = sessionStorage.getItem('username');
sessionStorage.removeItem('username');
sessionStorage.clear();
const user = { name: 'JaneDoe', age: 30 };
sessionStorage.setItem('user', JSON.stringify(user));
const storedUser = JSON.parse(sessionStorage.getItem('user') || '{}');
Session storage provides a convenient way to store data locally within the user's browser session, but it comes with limitations such as session scope, storage capacity, and string-only data. By understanding these constraints and leveraging session storage effectively in TypeScript applications, developers can create more responsive and interactive web experiences while respecting user privacy and security.