Understanding LocalStorage Limitations in TypeScript
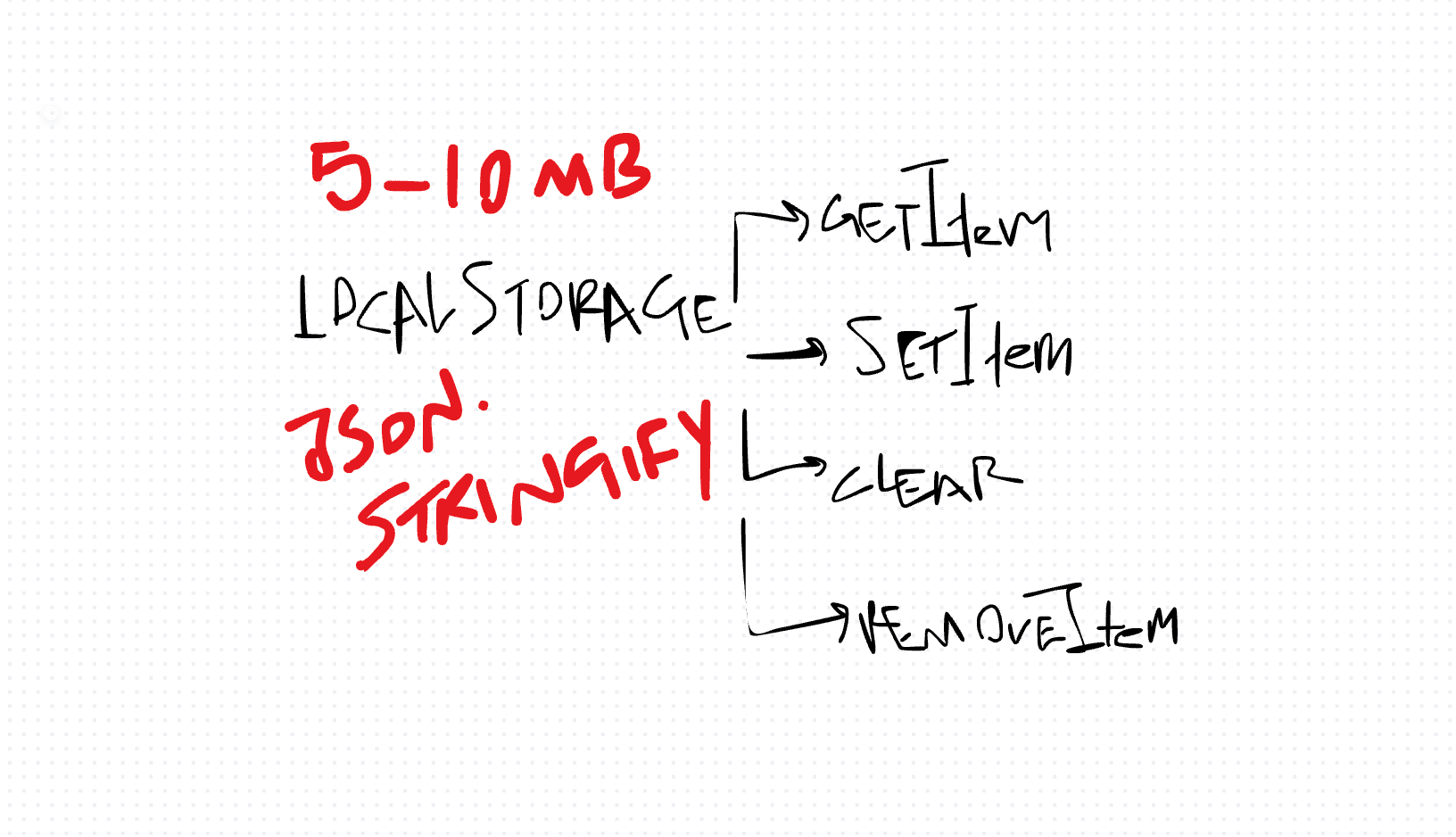
One of the primary constraints of LocalStorage is its storage capacity. Each origin, determined by the combination of protocol, hostname, and port number, typically has a maximum storage limit enforced by browsers, usually ranging from 5 to 10 MB. Here's how TypeScript developers can handle this limitation:
const dataToStore = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.';
try {
localStorage.setItem('exampleKey', dataToStore);
} catch (error) {
console.error('LocalStorage capacity exceeded:', error);
}
LocalStorage exclusively stores data as strings. Consequently, when working with TypeScript, developers must serialize complex data structures like objects or arrays into strings before storing them. Below is an example of serializing and storing data in LocalStorage using TypeScript:
const complexData = { key: 'value', array: [1, 2, 3] };
const serializedData = JSON.stringify(complexData);
localStorage.setItem('complexData', serializedData);
Reading from and writing to LocalStorage are blocking operations, potentially impacting the performance of TypeScript applications, especially when dealing with large datasets or frequent data operations. TypeScript developers can mitigate this limitation by implementing asynchronous operations where applicable:
async function storeData(key: string, value: any): Promise<void> {
return new Promise<void>((resolve, reject) => {
try {
localStorage.setItem(key, JSON.stringify(value));
resolve();
} catch (error) {
reject(error);
}
});
}
// Usage example:
storeData('exampleKey', { value: 'data' })
.then(() => console.log('Data stored successfully.'))
.catch(error => console.error('Error storing data:', error));
While LocalStorage is generally considered safe for storing non-sensitive data, TypeScript developers must exercise caution when storing sensitive information, as data stored in LocalStorage is accessible via JavaScript and susceptible to cross-site scripting (XSS) attacks. It's advisable to avoid storing sensitive data in LocalStorage or implement encryption mechanisms when necessary.
LocalStorage serves as a valuable tool for storing data locally within web applications, including those built with TypeScript. By understanding and addressing its limitations effectively, TypeScript developers can leverage LocalStorage to enhance user experiences while ensuring optimal performance and security in their applications. With careful consideration and appropriate usage, LocalStorage remains a powerful asset in the TypeScript developer's arsenal.