Active Object Design Pattern
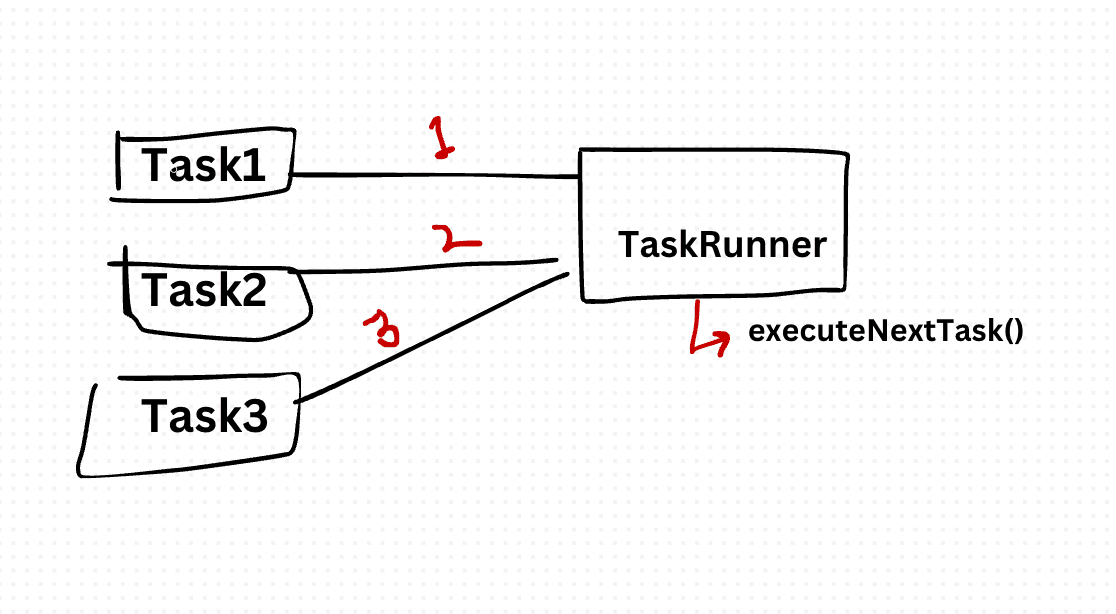
The Active Object Pattern involves separating method invocation from method execution by encapsulating each method call into an object called an "active object." These active objects maintain a queue of method requests and execute them asynchronously in the background. This pattern promotes concurrency, responsiveness, and modularity in software systems by allowing tasks to be performed concurrently without blocking the calling thread.
class TaskRunner {
constructor() {
this.taskQueue = [];
this.active = false;
}
enqueueTask(task) {
this.taskQueue.push(task);
if (!this.active) {
this.executeNextTask();
}
}
executeNextTask() {
if (this.taskQueue.length > 0) {
this.active = true;
const task = this.taskQueue.shift();
task().then(() => {
this.active = false;
this.executeNextTask();
});
}
}
}
// Example Task
function sampleTask() {
return new Promise(resolve => {
setTimeout(() => {
console.log("Task executed");
resolve();
}, 1000);
});
}
// Usage
const taskRunner = new TaskRunner();
taskRunner.enqueueTask(sampleTask);
taskRunner.enqueueTask(sampleTask);
The Active Object Pattern provides an effective solution for handling concurrency and asynchronous operations in JavaScript applications. By decoupling method invocation from method execution and executing tasks asynchronously in the background, this pattern enhances responsiveness, scalability, and modularity. In JavaScript, the Active Object pattern is commonly used in scenarios such as event handling, message passing, and asynchronous task processing.