Adapter Design Pattern
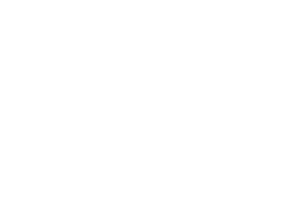
Often, we encounter scenarios where existing classes or components have interfaces that are not compatible with the interface expected by clients. Instead of modifying existing code or interfaces, the Adapter Design Pattern provides a solution by creating an adapter that acts as an intermediary, allowing the incompatible interfaces to work together seamlessly.
// Adaptee (Legacy Component)
class LegacyLogger {
log(message) {
console.log(`Legacy Logger: ${message}`);
}
}
class Logger {
log(message) {
throw new Error("log method must be implemented.");
}
}
class LegacyLoggerAdapter extends Logger {
constructor() {
super();
this.legacyLogger = new LegacyLogger();
}
log(message) {
this.legacyLogger.log(message);
}
}
// Usage
const logger = new LegacyLoggerAdapter();
logger.log("This is a message.");
Logger is the target interface expected by clients, defining the log method.
The Adapter Design Pattern provides a practical solution for integrating incompatible interfaces, allowing components with different interfaces to work together harmoniously. By creating adapters that bridge the gap between interfaces, developers can achieve greater flexibility and maintainability in their codebases.