Best Practices for Structuring Express.js Applications with Prisma Design Pattern
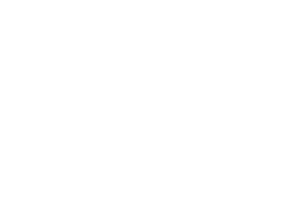
Express.js is a popular web application framework for Node.js known for its simplicity and flexibility. When combined with Prisma, a modern database toolkit, developers can build powerful and scalable backend applications with ease. In this article, we'll explore the best practices for organizing Express.js applications with Prisma to ensure clean, modular, and efficient code.
Folder Structure:
All in /src folder, This directory serves as the main source folder for our application:
- /controllers/: Contains controller modules responsible for handling HTTP requests and implementing business logic.
- models/: Includes Prisma models for defining data structures and interacting with the database.
- routes/: Houses route modules responsible for defining API endpoints and associating them with appropriate controller methods.
- services/: Contains service modules responsible for encapsulating business logic, such as authentication, data validation, or third-party integrations.
- middlewares/: Holds middleware functions that intercept and modify incoming HTTP requests or outgoing responses.
- config/: Includes configuration files for environment-specific settings, database connections, or external service configurations.
- utils/: Contains utility modules for common functions, helper methods, or reusable components across the application.
- tests/: Houses test files for unit tests, integration tests, or end-to-end tests to ensure the reliability and correctness of the application.
- app.js: The entry point of the application where middleware, routes, and other configurations are initialized.
- index.js: Initializes the Express server and listens for incoming HTTP requests.
Best Practices:
- Separation of Concerns: Follow the MVC (Model-View-Controller) architecture to separate concerns between data models, business logic (controllers/services), and presentation (routes).
- Modular Routing: Use modular routing to organize API endpoints into separate route files, promoting scalability and maintainability.
- Middleware Usage: Utilize middleware functions for common tasks like logging, error handling, authentication, or request validation to keep route handlers clean and focused.
- Service Layer: Implement a service layer to encapsulate business logic and promote code reusability across multiple controllers or routes.
- Error Handling: Centralize error handling logic using middleware to catch and respond to errors consistently across the application.
- Environment Configuration: Use environment variables and configuration files to manage environment-specific settings, such as database URLs, API keys, or logging levels.
- Dependency Injection: Employ dependency injection to inject dependencies into controllers, services, or middleware, facilitating testing and reducing tight coupling.
- Logging and Monitoring: Implement logging mechanisms to track application behavior and performance, and integrate monitoring tools for real-time insights into application health.
- Security Practices: Adhere to security best practices, such as input validation, parameterized queries, and protection against common vulnerabilities like SQL injection or cross-site scripting (XSS).
- Testing: Write comprehensive test suites covering unit tests for individual components, integration tests for modules interacting with external dependencies, and end-to-end tests to validate application behavior from the client's perspective.
Conclusion:
By following these best practices and organizing our Express.js applications with Prisma, we can create scalable, maintainable, and efficient backend systems. Leveraging Prisma's capabilities for data modeling and database interactions enhances productivity and simplifies database management, allowing developers to focus on building robust and feature-rich applications. With a clear folder structure, modular components, and separation of concerns, Express.js applications with Prisma can meet the demands of modern web development and deliver exceptional user experiences.