Builder Design Pattern
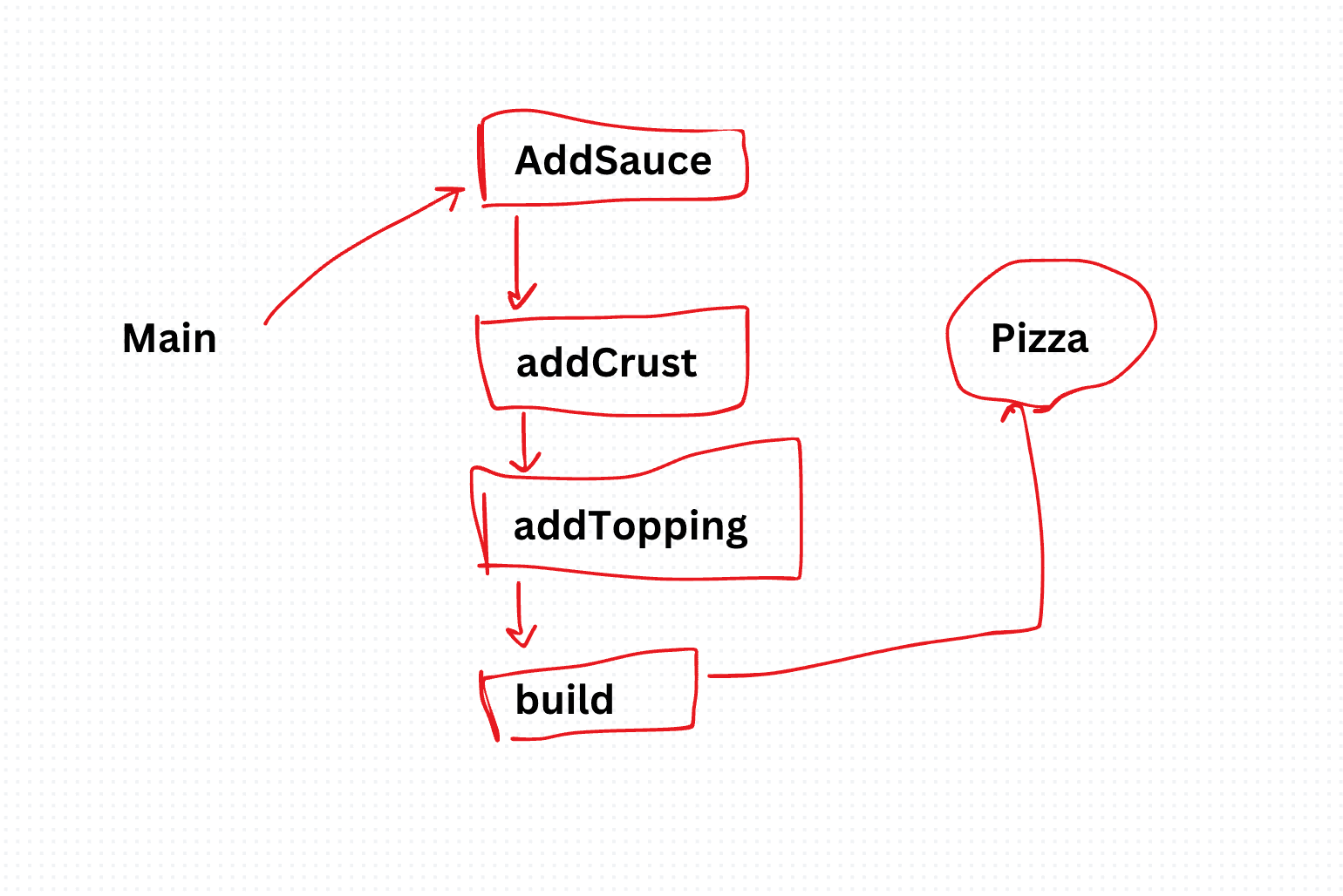
The Builder Design Pattern is particularly useful when an object's construction process involves multiple steps or configurations. It provides a clear and fluent interface for constructing objects, allowing clients to specify different configurations without needing to understand the complex construction logic.
class Pizza {
constructor() {
this.crust = "";
this.sauce = "";
this.toppings = [];
}
addCrust(crust) {
this.crust = crust;
}
addSauce(sauce) {
this.sauce = sauce;
}
addTopping(topping) {
this.toppings.push(topping);
}
describe() {
console.log(`Pizza with ${this.crust} crust, ${this.sauce} sauce, and toppings: ${this.toppings.join(", ")}.`);
}
}
// Builder class
class PizzaBuilder {
constructor() {
this.pizza = new Pizza();
}
addCrust(crust) {
this.pizza.addCrust(crust);
return this;
}
addSauce(sauce) {
this.pizza.addSauce(sauce);
return this;
}
addTopping(topping) {
this.pizza.addTopping(topping);
return this;
}
build() {
return this.pizza;
}
}
// Usage
const pizza = new PizzaBuilder()
.addCrust("thin")
.addSauce("tomato")
.addTopping("cheese")
.addTopping("mushrooms")
.build();
pizza.describe();
PizzaBuilder serves as the builder class, providing methods for adding crust, sauce, and toppings to the pizza.
The Builder Design Pattern offers a flexible and fluent way to construct complex objects with multiple configurations. By encapsulating the construction logic within a builder class, developers can create objects with different representations while keeping the client code clean and easy to understand.