Composite Design Pattern
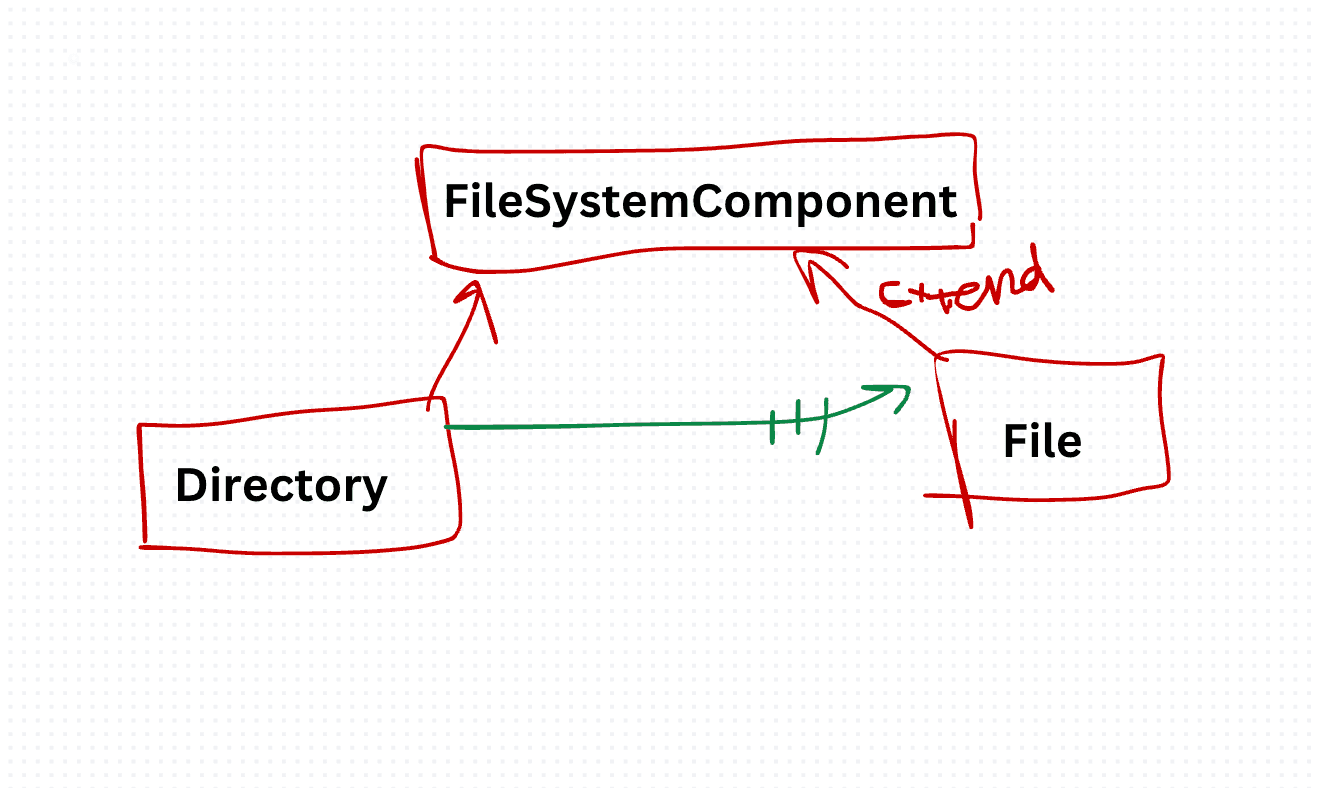
The Composite Design Pattern consists of three main components: Component, Leaf, and Composite.
- Component: This defines the common interface for all objects in the composition, providing default behavior for all objects, including leaf nodes and composite nodes.
- Leaf: This represents the individual objects in the composition. Leaf nodes have no children and implement the operations defined by the Component interface.
- Composite: This represents the composite objects in the composition, which are composed of leaf nodes and/or other composite nodes. Composite nodes delegate operations to their children.
/ Component interface
class FileSystemComponent {
constructor(name) {
this.name = name;
}
display() {
throw new Error("display method must be implemented.");
}
}
// Leaf
class File extends FileSystemComponent {
display() {
console.log(`File: ${this.name}`);
}
}
// Composite
class Directory extends FileSystemComponent {
constructor(name) {
super(name);
this.children = [];
}
add(component) {
this.children.push(component);
}
remove(component) {
const index = this.children.indexOf(component);
if (index !== -1) {
this.children.splice(index, 1);
}
}
display() {
console.log(`Directory: ${this.name}`);
this.children.forEach(child => child.display());
}
}
// Usage
const root = new Directory("Root");
const folder1 = new Directory("Folder 1");
const folder2 = new Directory("Folder 2");
const file1 = new File("File 1");
const file2 = new File("File 2");
root.add(folder1);
root.add(folder2);
folder1.add(file1);
folder2.add(file2);
root.display();
The Composite Design Pattern is a powerful tool for building tree-like structures of objects where individual objects and compositions of objects are treated uniformly. By understanding and implementing this pattern, developers can create flexible and scalable systems capable of representing complex hierarchies.