Director Design Pattern
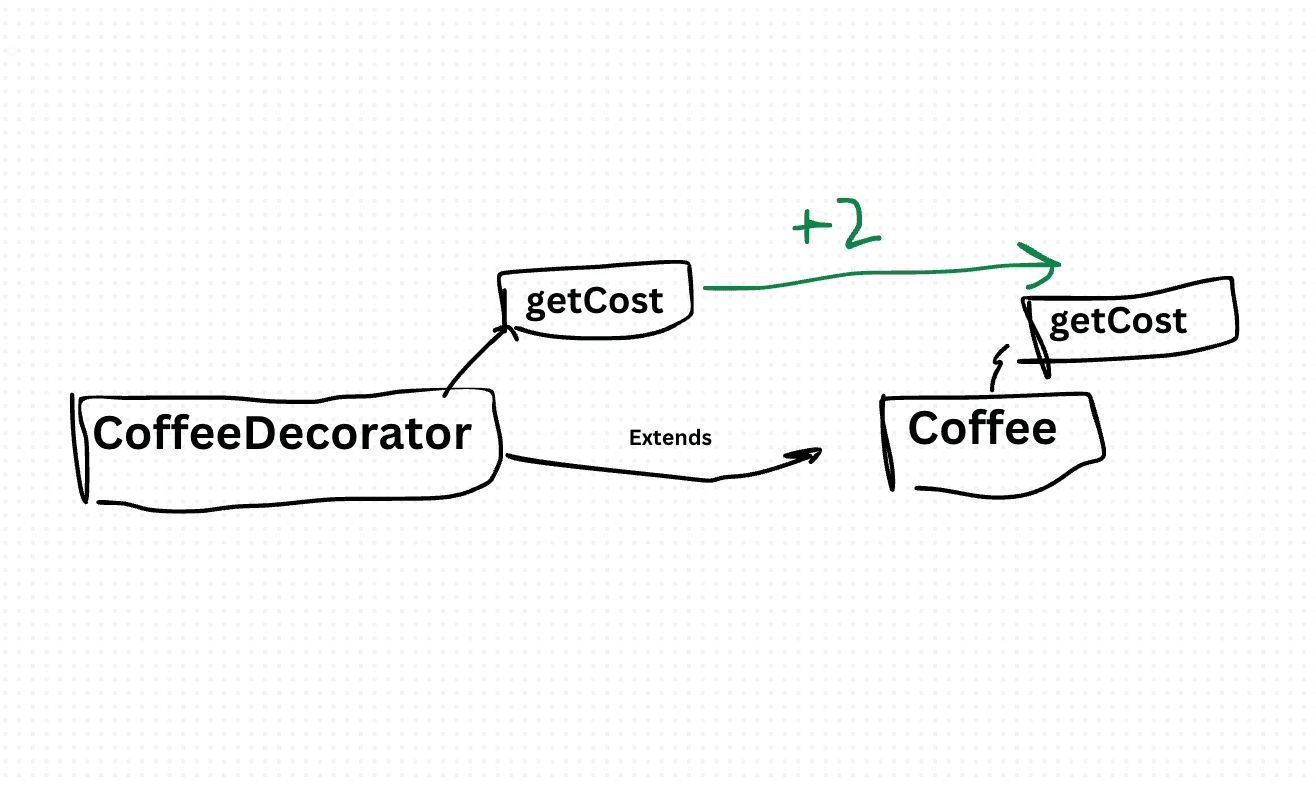
At its core, the Decorator Design Pattern aims to enhance the behavior of individual objects by wrapping them with one or more decorator objects. These decorator objects add new features or modify existing behavior without changing the original object's structure. This pattern adheres to the Open/Closed Principle, allowing classes to be easily extended with new functionality without modifying their source code.
class Coffee {
getCost() {
return 5; // Base cost of a coffee
}
getDescription() {
return "Coffee"; // Base description
}
}
// Decorator
class CoffeeDecorator extends Coffee {
constructor(coffee) {
super();
this.coffee = coffee;
}
getCost() {
return this.coffee.getCost();
}
getDescription() {
return this.coffee.getDescription();
}
}
// Concrete Decorators
class MilkDecorator extends CoffeeDecorator {
getCost() {
return this.coffee.getCost() + 2; // Additional cost for milk
}
getDescription() {
return this.coffee.getDescription() + ", Milk";
}
}
The Decorator Design Pattern offers a flexible and elegant solution for dynamically enhancing the behavior of individual objects.