Factory Method Design Pattern
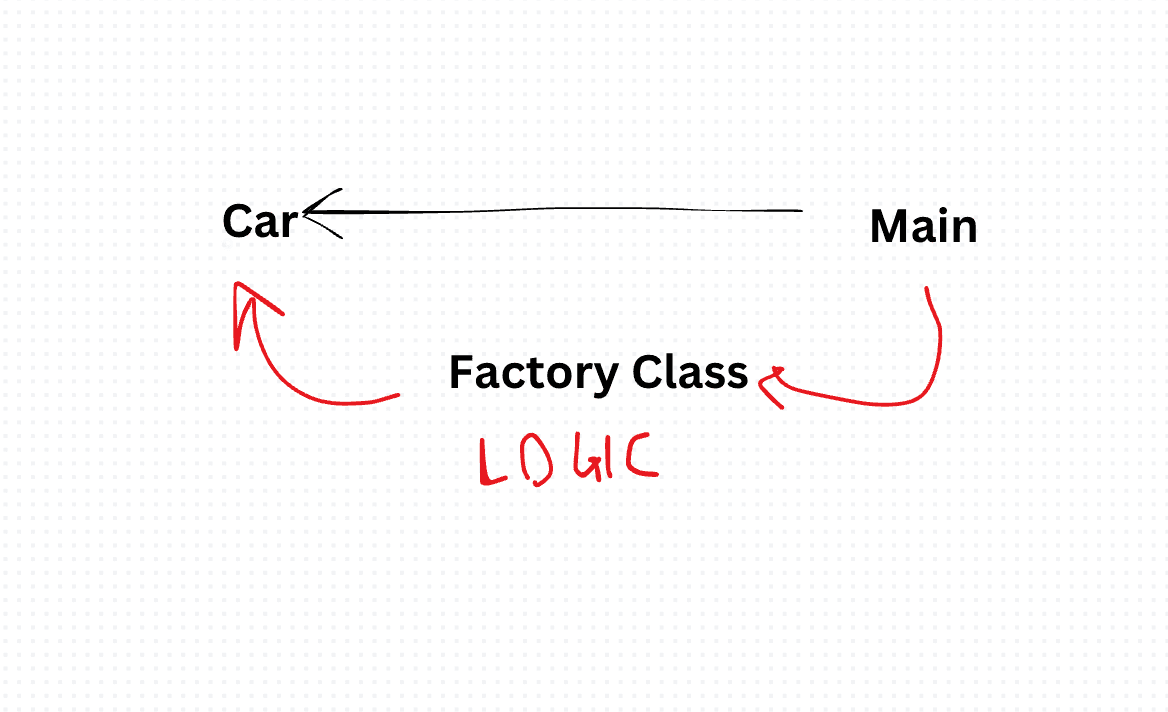
The Factory Method Pattern promotes loose coupling by encapsulating object creation logic in a separate method or class. Instead of directly instantiating objects using a constructor, clients rely on factory methods to create instances of objects.
class Vehicle {
constructor() {
if (this.constructor === Vehicle) {
throw new Error("Vehicle cannot be instantiated directly.");
}
}
display() {
console.log("This is a generic vehicle.");
}
}
and
// Concrete Products
class Car extends Vehicle {
display() {
console.log("This is a car.");
}
}
class Motorcycle extends Vehicle {
display() {
console.log("This is a motorcycle.");
}
}
here is the Factory
class VehicleFactory {
createVehicle() {
throw new Error("createVehicle method must be implemented.");
}
}
// Concrete Creator classes
class CarFactory extends VehicleFactory {
createVehicle() {
return new Car();
}
}
class MotorcycleFactory extends VehicleFactory {
createVehicle() {
return new Motorcycle();
}
}
// Usage
const carFactory = new CarFactory();
const car = carFactory.createVehicle();
car.display(); // Output: This is a car.
const motorcycleFactory = new MotorcycleFactory();
const motorcycle = motorcycleFactory.createVehicle();
motorcycle.display(); // Output: This is a motorcycle.
VehicleFactory serves as the creator class with a factory method createVehicle, which is responsible for creating instances of concrete products.
The Factory Method Pattern provides a flexible and scalable way to create objects by encapsulating object creation logic