Momento Design Pattern
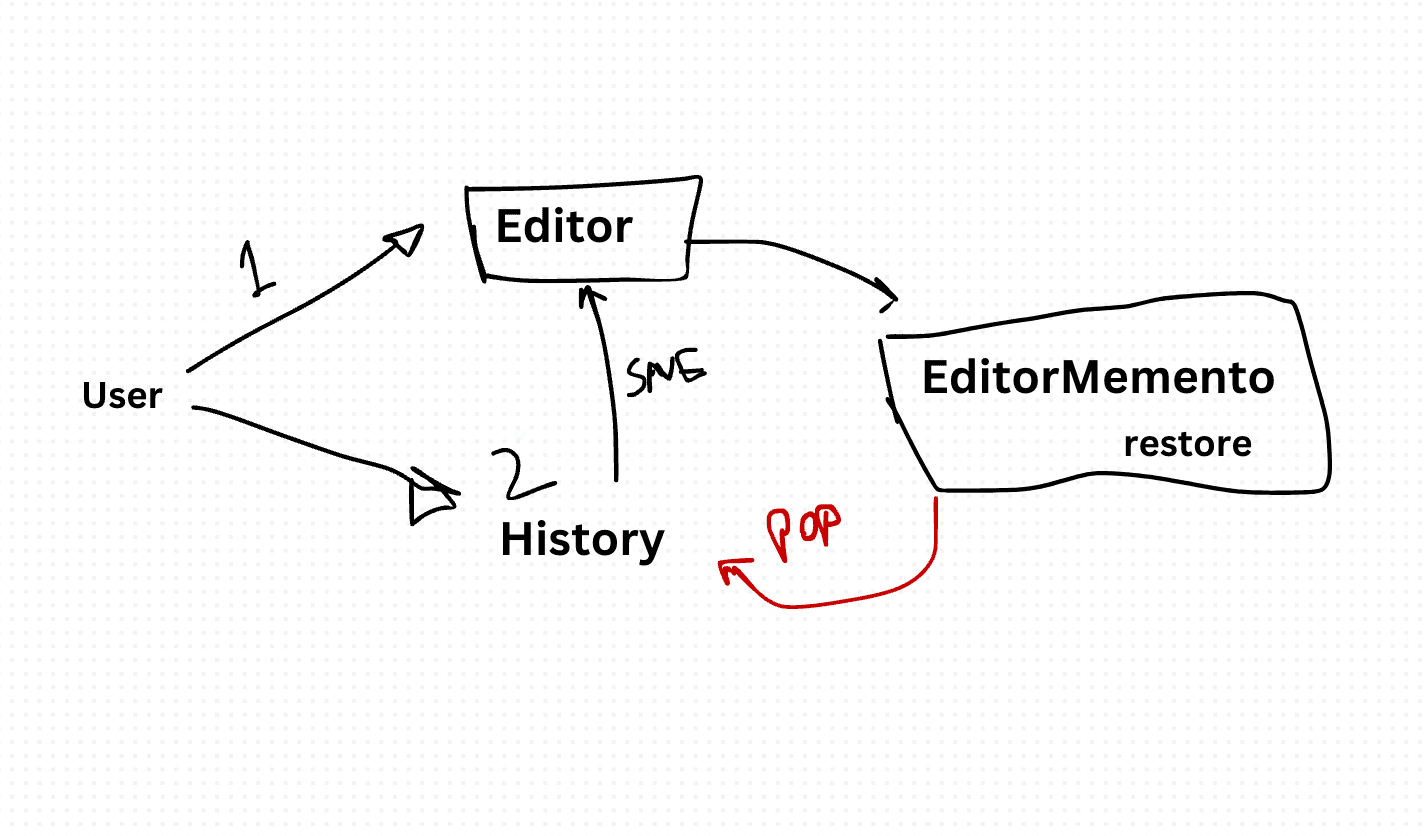
At its core, the Memento Design Pattern consists of three main components: the Originator, the Memento, and the Caretaker. The Originator is the object whose state needs to be saved, the Memento is an object that stores the state of the Originator, and the Caretaker is responsible for storing and managing Mementos. This pattern allows for the capture and restoration of an object's state without violating encapsulation, promoting flexibility and undo/redo functionalities.
// Memento
class EditorMemento {
constructor(content) {
this.content = content;
}
getContent() {
return this.content;
}
}
// Originator
class Editor {
constructor() {
this.content = "";
}
type(text) {
this.content += text;
}
save() {
return new EditorMemento(this.content);
}
restore(memento) {
this.content = memento.getContent();
}
}
// Caretaker
class History {
constructor() {
this.states = [];
}
push(memento) {
this.states.push(memento);
}
pop() {
return this.states.pop();
}
}
// Usage
const editor = new Editor();
const history = new History();
editor.type("This is the first sentence. ");
history.push(editor.save());
editor.type("This is the second sentence. ");
console.log(editor.content); // Output: This is the first sentence. This is the second sentence.
editor.restore(history.pop());
console.log(editor.content);
The Memento Design Pattern provides a powerful mechanism for preserving and restoring an object's state without exposing its internal structure. By encapsulating state into Memento objects and managing them through Caretakers, this pattern enables flexible undo and redo functionalities while maintaining encapsulation and modularity. In JavaScript, the Memento pattern is commonly used in scenarios such as text editors, form data management, and transactional systems.