Observer Design Pattern
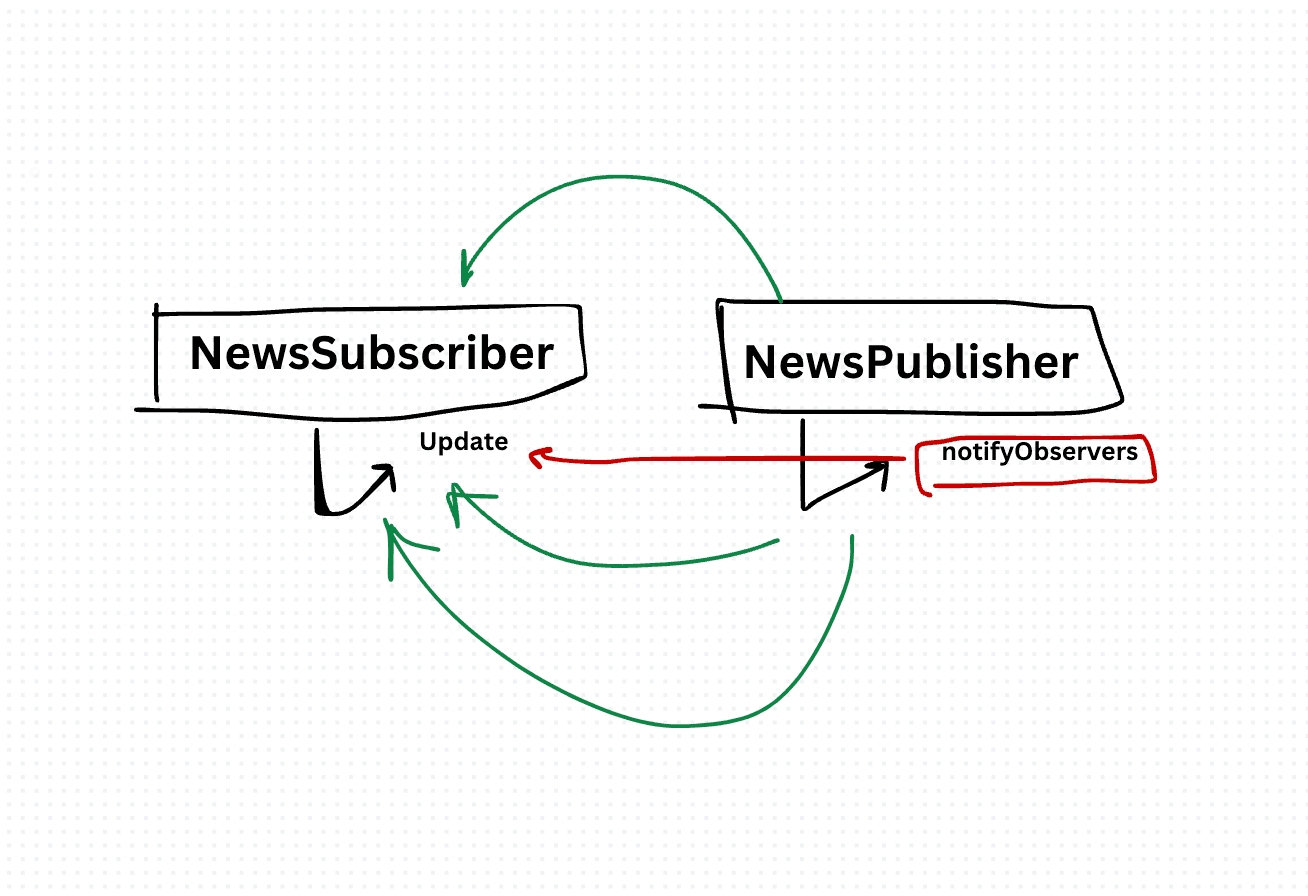
At its core, the Observer Design Pattern consists of two key components: the Subject and the Observers. The Subject is the object being observed, while the Observers are the objects interested in its state changes. Whenever the state of the Subject changes, it notifies all registered Observers, triggering them to update themselves accordingly. This pattern is widely used in event handling systems, UI frameworks, and reactive programming.
class NewsPublisher {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
removeObserver(observer) {
this.observers = this.observers.filter(obs => obs !== observer);
}
notifyObservers(news) {
this.observers.forEach(observer => observer.update(news));
}
}
Then:
// Observer (Subscriber)
class NewsSubscriber {
constructor(name) {
this.name = name;
}
update(news) {
console.log(`${this.name} received news: ${news}`);
}
}
And use it like this:
const publisher = new NewsPublisher();
const subscriber1 = new NewsSubscriber("Subscriber 1");
const subscriber2 = new NewsSubscriber("Subscriber 2");
publisher.addObserver(subscriber1);
publisher.addObserver(subscriber2);
publisher.notifyObservers("Breaking news: JavaScript wins Language of the Year!");
publisher.removeObserver(subscriber1);
publisher.notifyObservers("Latest update: ES12 features announced!");
The Observer Design Pattern provides an elegant solution for implementing communication between objects in a loosely coupled manner. By decoupling the Subject from its Observers, this pattern promotes modularity, reusability, and maintainability in software systems. In JavaScript, the Observer pattern is commonly used in various scenarios such as event handling, pub/sub systems, and reactive programming libraries.