Unit of Work Design Pattern
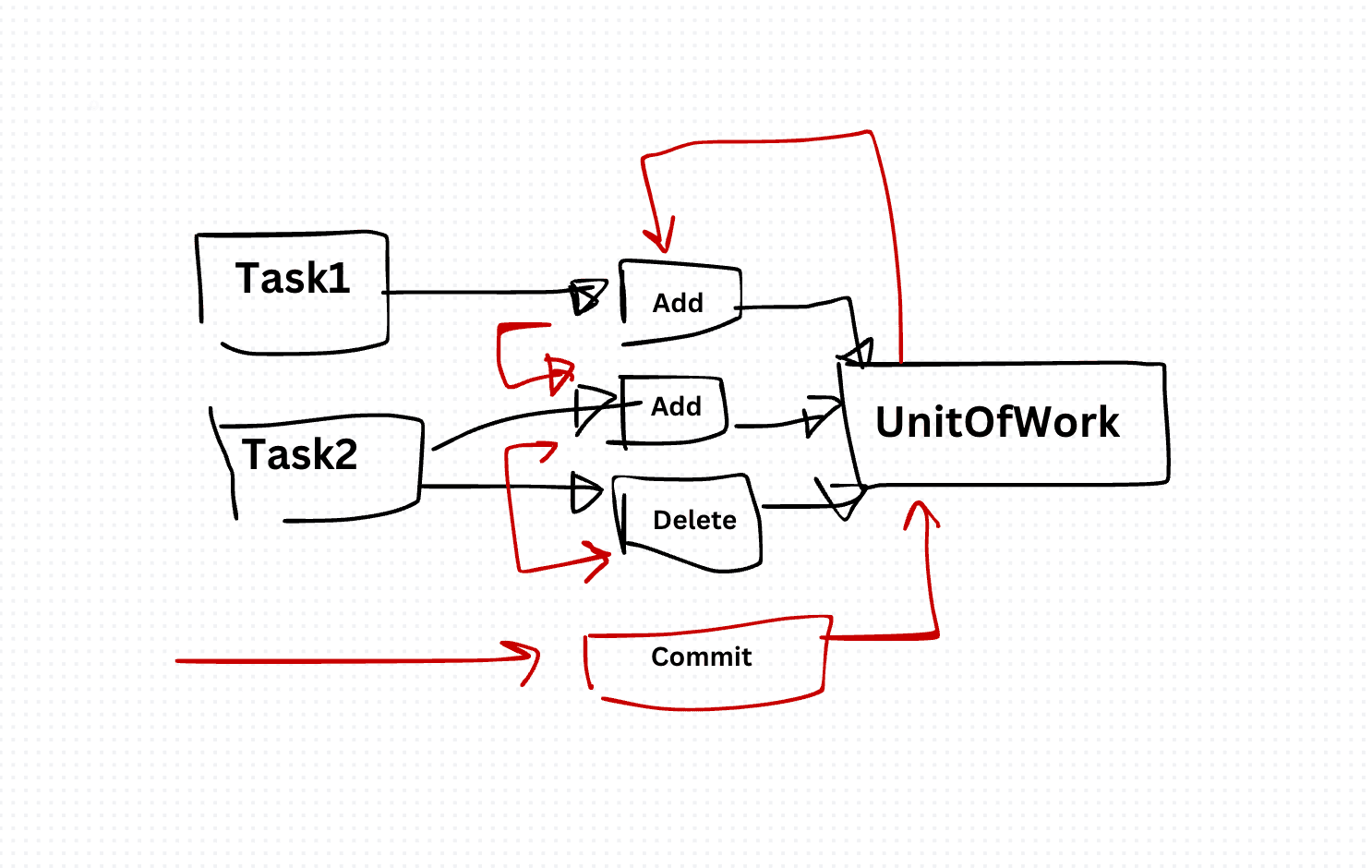
The Unit of Work pattern is based on the concept of treating a series of related data operations as a single unit of work. It provides a way to track and manage changes to entities within a transaction boundary, ensuring that all changes are committed or rolled back atomically. This pattern is commonly used in applications that involve complex data interactions, such as web applications with multiple data updates across different entities.
// Task Model
class Task {
constructor(id, title) {
this.id = id;
this.title = title;
}
}
// Unit of Work
class UnitOfWork {
constructor() {
this.tasksToAdd = [];
this.tasksToDelete = [];
}
registerNew(task) {
this.tasksToAdd.push(task);
}
registerDeleted(task) {
this.tasksToDelete.push(task);
}
commit() {
// Logic to commit changes to the database
console.log('Committing changes to the database...');
this.tasksToAdd.forEach(task => {
console.log('Adding task:', task);
// Perform database insertion
});
this.tasksToDelete.forEach(task => {
console.log('Deleting task:', task);
// Perform database deletion
});
console.log('Changes committed successfully.');
}
}
// Example usage
const unitOfWork = new UnitOfWork();
// Register new tasks
unitOfWork.registerNew(new Task(null, 'Task 1'));
unitOfWork.registerNew(new Task(null, 'Task 2'));
// Delete tasks (assuming tasks with IDs 1 and 2 exist)
unitOfWork.registerDeleted(new Task(1, ''));
unitOfWork.registerDeleted(new Task(2, ''));
// Commit changes
unitOfWork.commit();
The Unit of Work Design Pattern provides a structured approach to managing data persistence operations within a transaction boundary. By encapsulating changes to entities and coordinating their interactions, developers can ensure data integrity and consistency. In JavaScript applications, the Unit of Work pattern can be used to handle complex data operations efficiently, leading to more robust and maintainable code.