Describe the difference between symmetric and asymmetric encryption, and when each is appropriate.
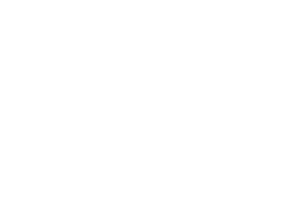
Symmetric encryption, as the name suggests, relies on a shared secret between parties involved in communication. In this method, a single key is used both for encryption and decryption of data. This key must be kept secret and shared securely between authorized parties.
const crypto = require('crypto');
// Generate a random symmetric key
const key = crypto.randomBytes(32);
// Data to be encrypted
const data = 'Sensitive information';
// Encrypt the data using the symmetric key
const cipher = crypto.createCipher('aes-256-cbc', key);
let encryptedData = cipher.update(data, 'utf8', 'hex');
encryptedData += cipher.final('hex');
console.log('Encrypted Data:', encryptedData);
// Decrypt the data using the same symmetric key
const decipher = crypto.createDecipher('aes-256-cbc', key);
let decryptedData = decipher.update(encryptedData, 'hex', 'utf8');
decryptedData += decipher.final('utf8');
console.log('Decrypted Data:', decryptedData);
In symmetric encryption, due to its simplicity and efficiency, it is suitable for encrypting large amounts of data, such as files or database records. However, the primary challenge lies in securely sharing the secret key between communicating parties.
Asymmetric encryption employs a pair of keys: a public key for encryption and a private key for decryption. Unlike symmetric encryption, the public key can be freely distributed, while the private key is kept secret.
const crypto = require('crypto');
// Generate a pair of asymmetric keys
const { publicKey, privateKey } = crypto.generateKeyPairSync('rsa', {
modulusLength: 2048,
});
// Data to be encrypted
const data = 'Sensitive information';
// Encrypt the data using the public key
const encryptedData = crypto.publicEncrypt(publicKey, Buffer.from(data, 'utf8')).toString('base64');
console.log('Encrypted Data:', encryptedData);
// Decrypt the data using the private key
const decryptedData = crypto.privateDecrypt(privateKey, Buffer.from(encryptedData, 'base64')).toString('utf8');
console.log('Decrypted Data:', decryptedData);
Asymmetric encryption excels in scenarios where secure communication channels for sharing secret keys are impractical or impossible. It facilitates secure key exchange, digital signatures, and user authentication.
Choosing the Right Encryption Method
- Symmetric Encryption: Suitable for encrypting large volumes of data efficiently. Ideal when secure sharing of a secret key is feasible and practical.
- Asymmetric Encryption: Appropriate for scenarios requiring secure communication over untrusted channels. Ideal for key exchange, digital signatures, and user authentication.
In conclusion, both symmetric and asymmetric encryption play critical roles in securing digital communication and data storage.