What is a Buffer Overflow vulnerability, and how can it be exploited?
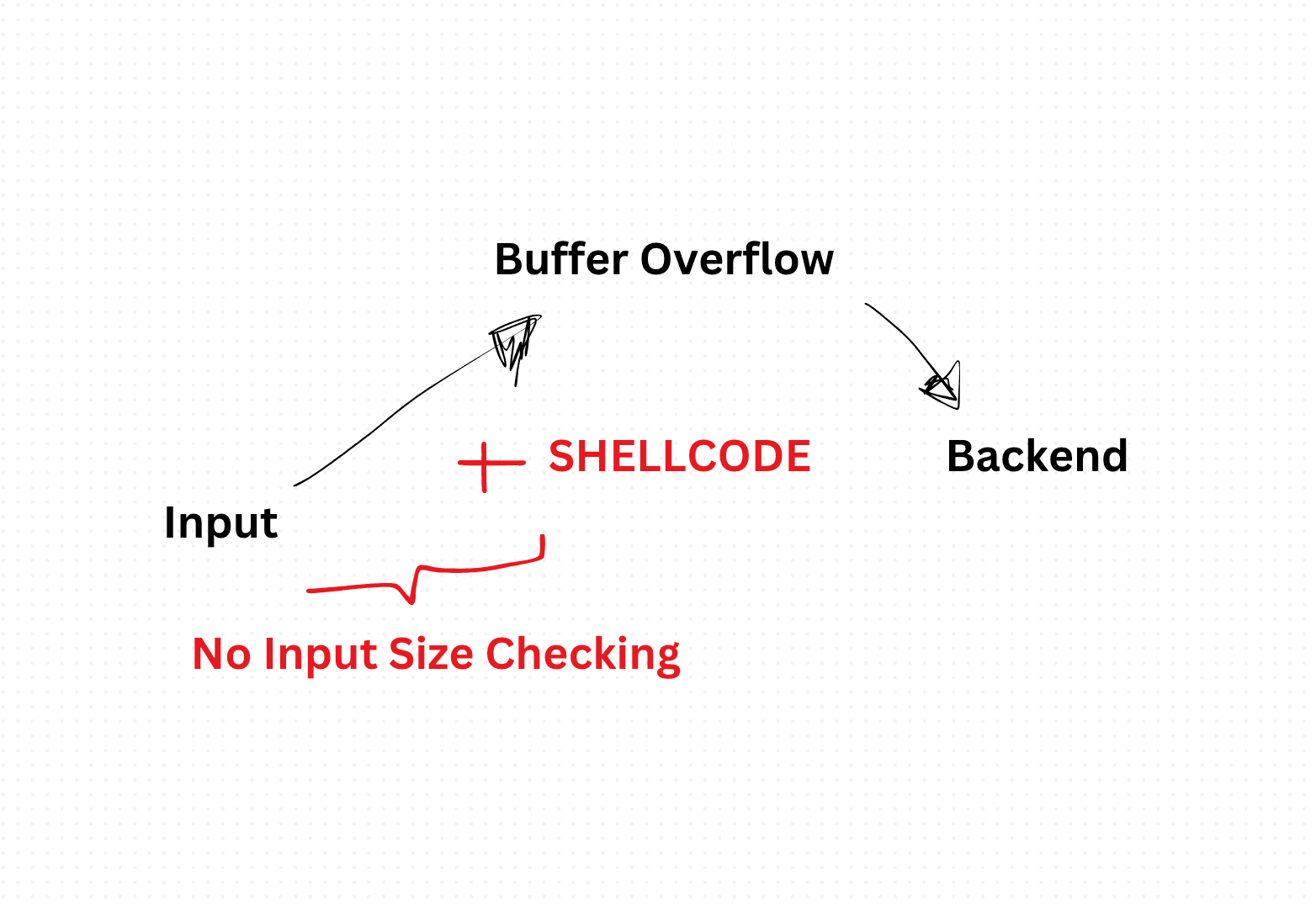
One of the oldest yet persistent threats in software development. In this article, we'll unravel the enigma surrounding Buffer Overflows, explore how attackers exploit them, and equip ourselves with JavaScript examples to combat this menace. Buffer Overflow arises when a program attempts to write data beyond the bounds of a buffer, leading to memory corruption. This vulnerability enables attackers to inject malicious code into the program's memory, potentially compromising system security.
Imagine a JavaScript function that reads user input into a buffer without performing bounds checking:
function vulnerableFunction(input) {
let buffer = new Array(10);
buffer.fill(0);
buffer = input; // Vulnerability: No bounds checking
}
If an attacker provides input larger than the buffer size, it overflows into adjacent memory, creating an opportunity for exploitation.
const maliciousInput = 'A'.repeat(20) + '\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90\x90';
vulnerableFunction(maliciousInput);
Here, the attacker sends a string longer than the buffer size, followed by shellcode represented in hexadecimal format. Upon overflow, the injected shellcode is executed, granting the attacker control over the program.
To counter Buffer Overflow vulnerabilities, adhere to these mitigation strategies:
- Bounds Checking: Always validate input sizes to ensure they do not exceed buffer capacities.
function safeFunction(input) {
if (input.length <= buffer.length) {
buffer = input;
} else {
// Handle error or truncate input
}
}
- Utilize Safe APIs: Opt for safer alternatives like Buffer.alloc() in Node.js or ArrayBuffer in browsers for buffer allocation.
let buffer = Buffer.alloc(10); // Safe buffer allocation
Buffer Overflow vulnerabilities pose significant risks to software security, enabling attackers to compromise system integrity. By understanding the intricacies of Buffer Overflows and implementing robust countermeasures, developers can fortify their applications against this persistent threat.