Explain SQL Injection and how to prevent it in software applications.
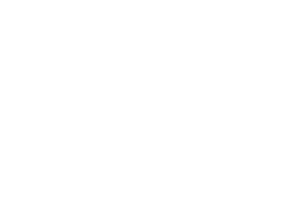
This nasty bug can wreak havoc on your software applications if you're not careful. But fear not! We'll delve into what SQL Injection is all about and arm ourselves with JavaScript examples to fend off this menace.
Understanding SQL Injection:
SQL Injection is like a Trojan horse sneaking into your application's database through user input. Attackers inject malicious SQL queries into input fields, exploiting vulnerabilities to steal, modify, or delete sensitive data.
Example Scenario:
Imagine a login form where users enter their credentials. Now, if the application isn't properly secured, a crafty attacker could input something like this:
const username = "' OR 1=1;--";
const password = "password123";
const query = `SELECT * FROM users WHERE username='${username}' AND password='${password}'`;
If the application blindly concatenates user inputs into SQL queries without proper validation, the attacker's input effectively bypasses authentication and gains unauthorized access.
Preventing SQL Injection with JavaScript:
Now, let's armor up our applications with some JavaScript defenses against SQL Injection.
- Parameterized Queries: Use parameterized queries to separate SQL logic from user data, preventing injection attacks.
const query = 'SELECT * FROM users WHERE username = ? AND password = ?';
connection.query(query, [username, password], (error, results) => {
// Handle query results
});
- Input Sanitization: Sanitize user input by removing or escaping special characters that could be interpreted as SQL code.
const username = sanitizeInput(req.body.username);
const password = sanitizeInput(req.body.password);
function sanitizeInput(input) {
return input.replace(/['";\\]/g, '');
}
- Object Relational Mapping (ORM): Use ORM libraries like Sequelize or TypeORM to abstract database interactions and automatically handle input sanitization.
const user = await User.findOne({
where: {
username: req.body.username,
password: req.body.password
}
});
SQL Injection is a serious threat to software applications, but with the right defenses in place, we can keep our data safe and sound. By adopting practices like parameterized queries, input sanitization, and ORM usage, we can fortify our applications against SQL Injection attacks.