Retrieving Employee Information with Department Name
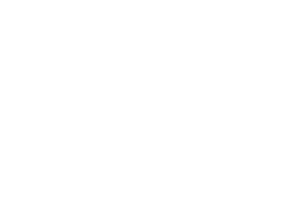
To begin, let's consider a TypeScript function that executes a SQL query to retrieve employee information along with their department names. We'll utilize TypeORM, a popular TypeScript ORM library, for database interactions.
import { getConnection } from 'typeorm';
async function getEmployeeDetailsWithDepartment(): Promise<any[]> {
const connection = getConnection();
const queryResult = await connection.query(`
SELECT e.*, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.department_id
`);
return queryResult;
}
Retrieving employee information along with their department names is a common task in database applications. By leveraging the JOIN clause in SQL, we can efficiently combine data from multiple tables to fulfill such requirements. In TypeScript, we can use libraries like TypeORM to execute SQL queries and fetch the desired results seamlessly. Understanding how to utilize TypeScript with pure SQL empowers developers to build robust and efficient database-driven applications.