Testing SetInterval in React Components
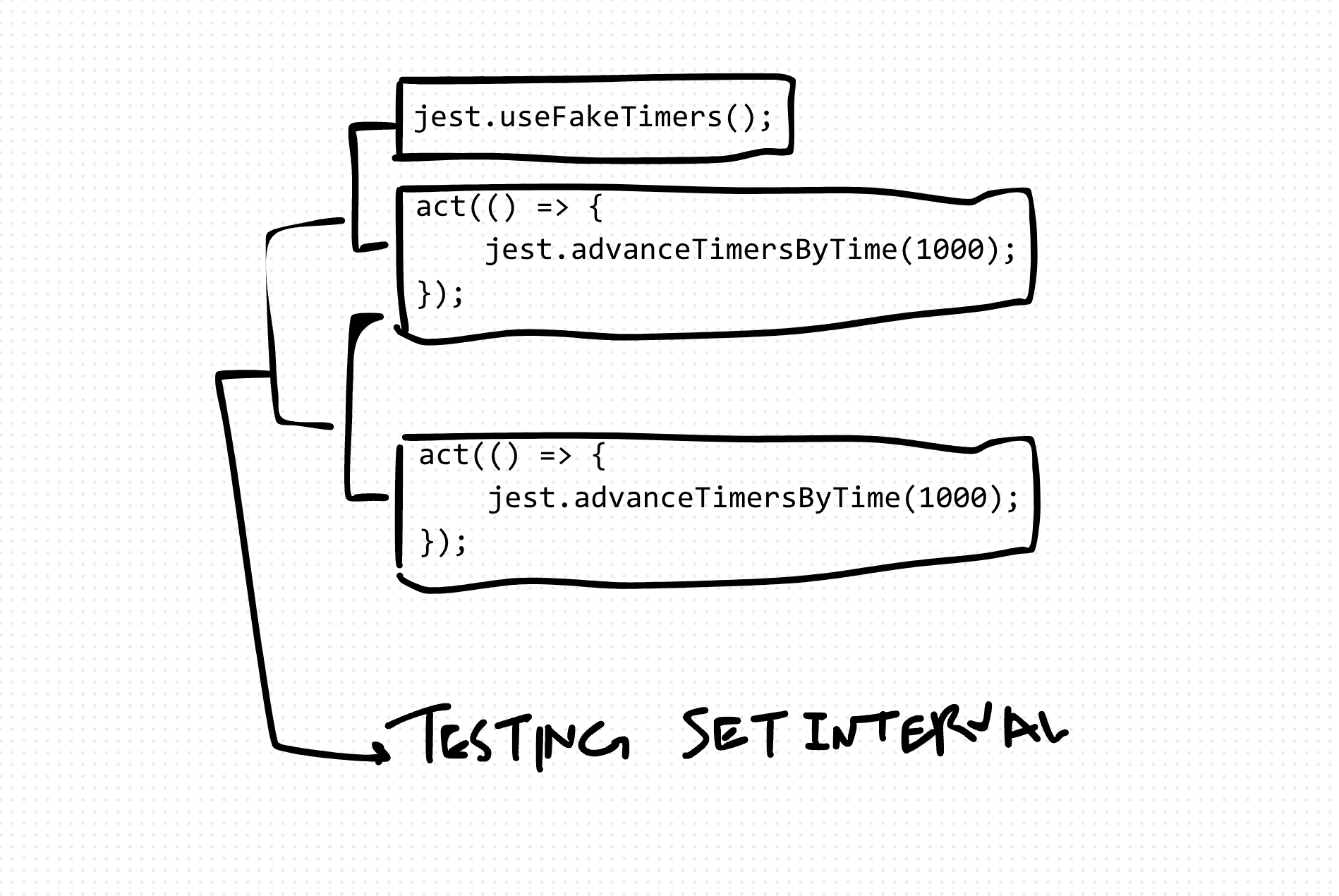
SetInterval is a built-in JavaScript function that repeatedly calls a function or executes a code snippet at specified time intervals. In React components, setInterval is often used for tasks like fetching data from an API periodically, updating UI components, or implementing real-time features.
Testing SetInterval in React Components:
- Mocking setInterval: When testing setInterval in React components, it's essential to mock the timer to control its behavior during tests. Jest, a popular testing framework, provides utilities for mocking timers.
- Using Jest's Fake Timers: Jest offers a feature called "fake timers," which allows you to control the passage of time in your tests. By using fake timers, you can simulate the behavior of setInterval without actually waiting for the intervals to elapse.
- Example Test Case: Let's consider a simple React component that uses setInterval to update a counter every second. Here's how you can test it:
import React from 'react';
import { render, act } from '@testing-library/react';
import CounterComponent from './CounterComponent';
describe('CounterComponent', () => {
it('updates counter every second', () => {
jest.useFakeTimers();
const { getByText } = render(<CounterComponent />);
// Initial counter value
expect(getByText('Counter: 0')).toBeInTheDocument();
// Fast-forward time by 1 second
act(() => {
jest.advanceTimersByTime(1000);
});
// Counter should be updated
expect(getByText('Counter: 1')).toBeInTheDocument();
// Fast-forward time by another 1 second
act(() => {
jest.advanceTimersByTime(1000);
});
// Counter should be updated again
expect(getByText('Counter: 2')).toBeInTheDocument();
});
});
In this test case, we use Jest's fake timers to simulate the passage of time. We render the CounterComponent, fast-forward time by 1 second using jest.advanceTimersByTime, and then assert that the counter is updated accordingly.
Conclusion:
Testing setInterval functionality in React components is essential to ensure the correctness and reliability of your application. By leveraging Jest's fake timers and employing proper testing techniques, you can thoroughly test components that use setInterval for various purposes.
By following the guidelines and examples outlined in this guide, you'll be able to write robust tests for setInterval functionality in your React components, enhancing the overall quality of your application.