Classes in TypeScript: A Comprehensive Guide
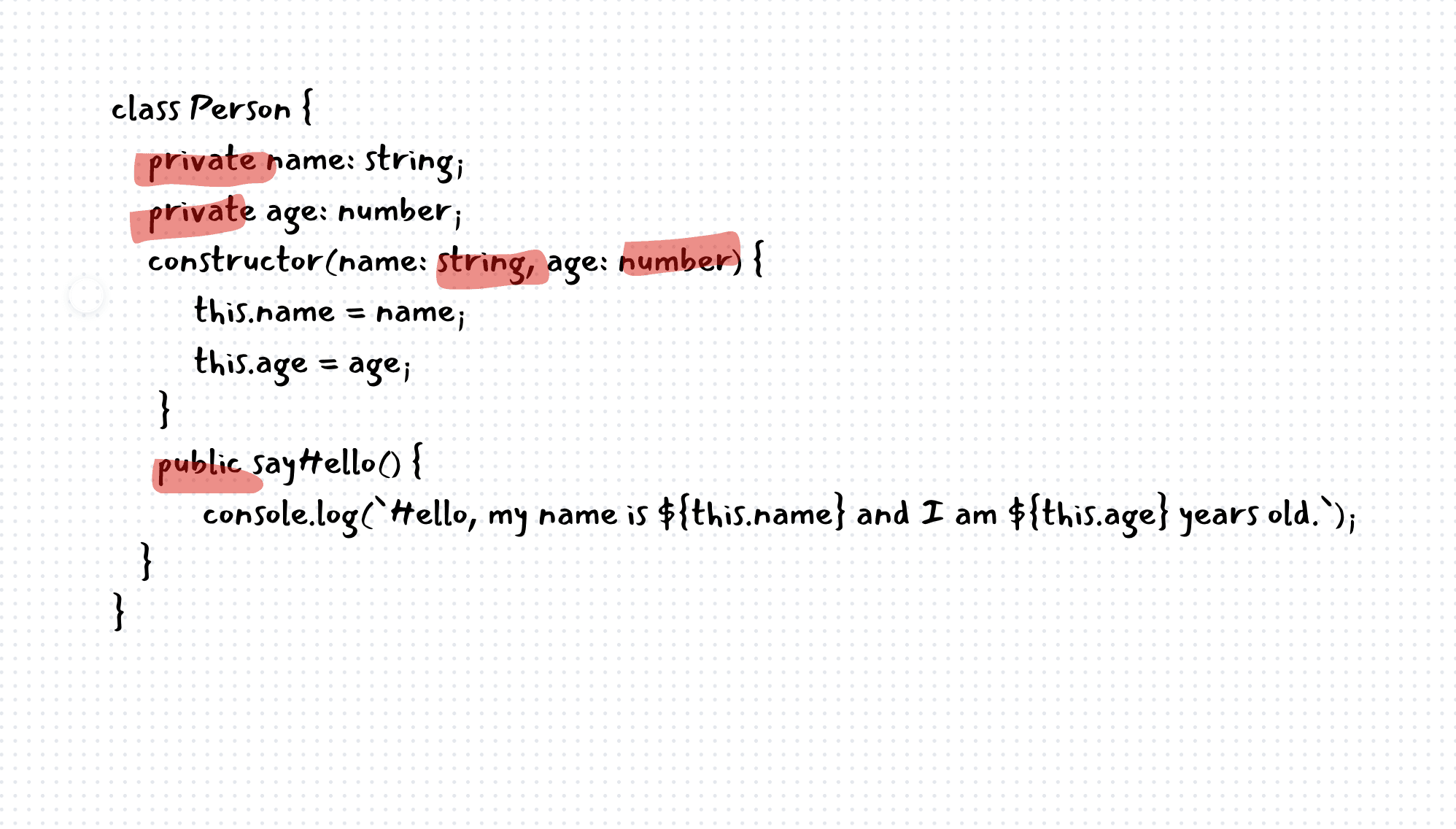
A class is a blueprint for creating objects. It defines the properties and methods that an object can have. Classes are a fundamental concept in object-oriented programming (OOP) and are used to create objects that have their own set of attributes and methods.
class Person {
private name: string;
private age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
public sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
In this example, we have defined a class called Person that has two properties: name and age. The name property is a string, and the age property is a number. The class also has a constructor that takes a name and age parameter, and a sayHello method that logs a message to the console.
Inheritance
One of the key features of classes in TypeScript is inheritance. Inheritance allows you to create a new class that inherits the properties and methods of an existing class. For example:
class Employee extends Person {
private department: string;
constructor(name: string, age: number, department: string) {
super(name, age);
this.department = department;
}
public sayHello() {
super.sayHello();
console.log(`I work in the ${this.department} department.`);
}
}
In this article, we have explored the concept of classes in TypeScript and provided examples to illustrate how they work. Classes are a powerful tool that can be used to create custom objects with their own set of attributes and methods. By using classes, you can write more robust and maintainable code that is easier to debug and maintain.