Interfaces in TypeScript: A Comprehensive Guide
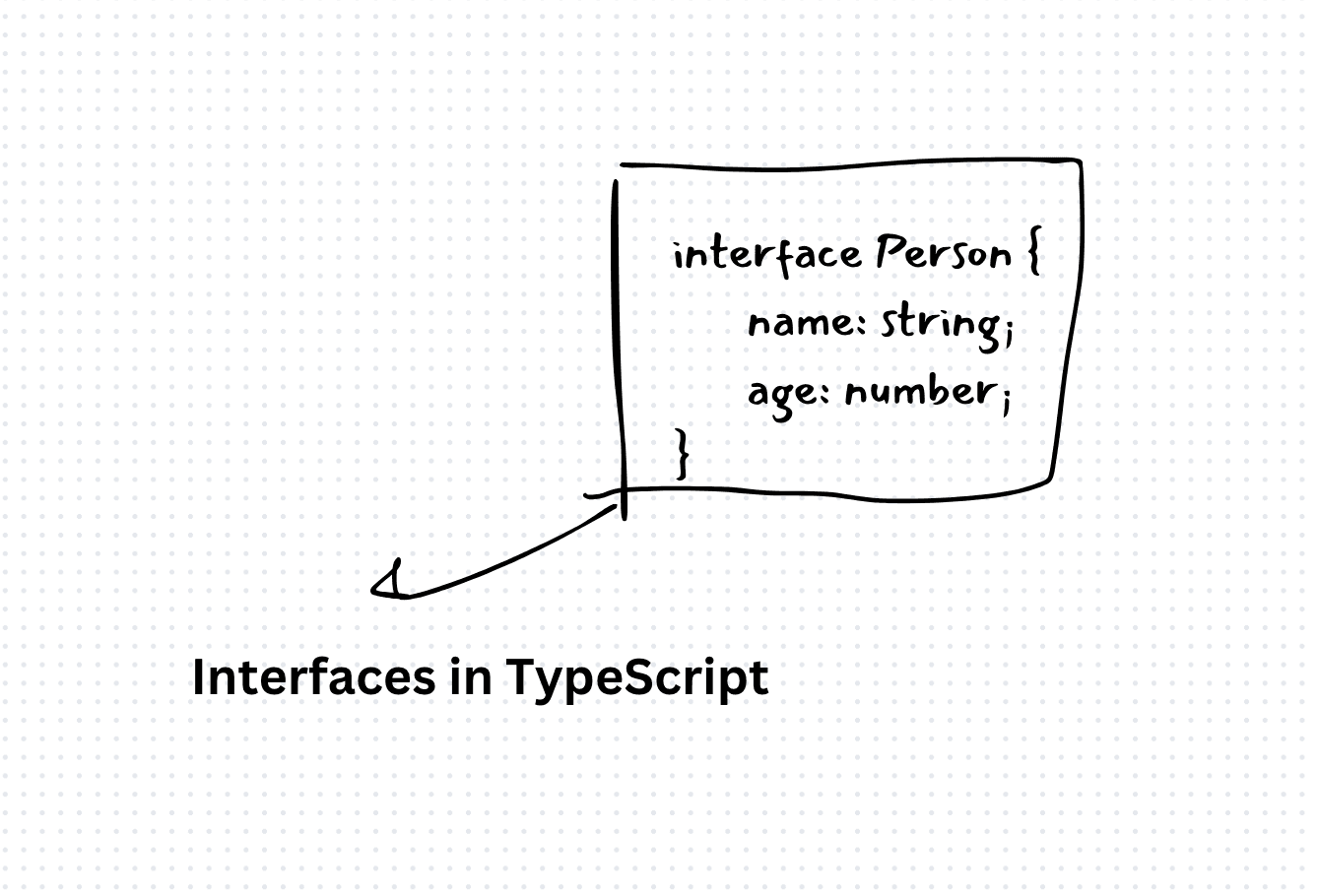
An interface is a way to define the structure of an object. It is a blueprint that specifies the properties and methods that an object must have. Interfaces are used to define the shape of an object, including its properties and methods, and can be used to ensure that an object conforms to a certain structure.
interface Person {
name: string;
age: number;
}
To implement an interface in TypeScript, you can use the implements keyword followed by the name of the interface. For example:
class Person implements Person {
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
public sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
Interfaces can be used to define the structure of an object, and can be used to ensure that an object conforms to a certain structure. For example:
let person: Person = {
name: 'John',
age: 30
};
console.log(person.name); // Output: John
console.log(person.age); // Output: 30
Benefits of Interfaces
Interfaces have several benefits, including:
- Type Safety: Interfaces ensure that an object conforms to a certain structure, which helps to prevent type-related errors.
- Code Reusability: Interfaces can be used to define the structure of an object, which can be reused throughout an application.
- Improved Code Readability: Interfaces can be used to define the structure of an object, which can improve the readability of code.
Conclusion
In this article, we have explored the concept of interfaces in TypeScript and provided examples to illustrate how they work. Interfaces are a powerful tool that can be used to define the structure of an object, and can be used to ensure that an object conforms to a certain structure. By using interfaces, you can write more robust and maintainable code that is easier to debug and maintain.