The New Map() Feature in TypeScript: A Game-Changer for Your Code
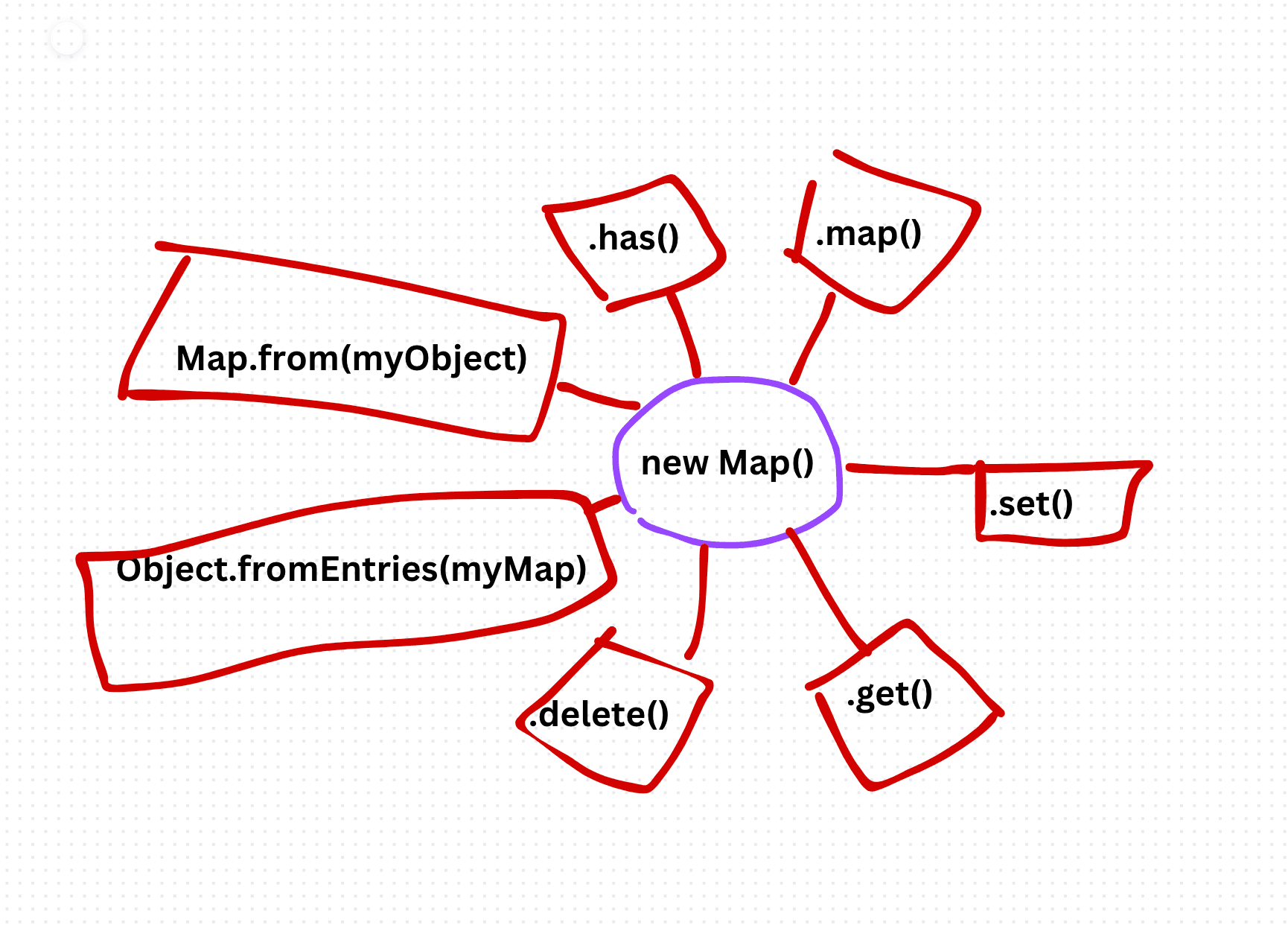
Are you tired of using Object to store and retrieve data in your TypeScript code? Well, say goodbye to those days with the new Map() feature! In this article, we'll dive into the world of Map() and explore its benefits, syntax, and code examples.
What's the big deal about Map()?
Map() is a built-in data structure that allows you to create a collection of key-value pairs. It's like a super-powered Object.
const myMap = new Map();
const myMap = new Map([
['key1', 'value1'],
['key2', 'value2'],
['key3', 'value3']
]);
const myMap = new Map();
myMap.set('key1', 'value1');
myMap.set('key2', 'value2');
console.log(myMap.get('key1')); // Output: "value1"
console.log(myMap.get('key2')); // Output: "value2"
console.log(myMap.has('key1')); // Output: true
myMap.delete('key1');
myMap.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
const obj = Object.fromEntries(myMap);
console.log(obj); // Output: { key1: "value1", key2: "value2", key3: "value3" }
const obj = { key1: "value1", key2: "value2", key3: "value3" };
const myMap = new Map(Object.entries(obj))
console.log(myMap);
The new Map() feature in TypeScript is a game-changer for your code. With its efficiency, flexibility, and ease of use, Map() is a must-have in your toolkit. So, what are you waiting for? Give Map() a try and take your code to the next level!