Distinction: Node.js Child Process vs. Node.js Worker
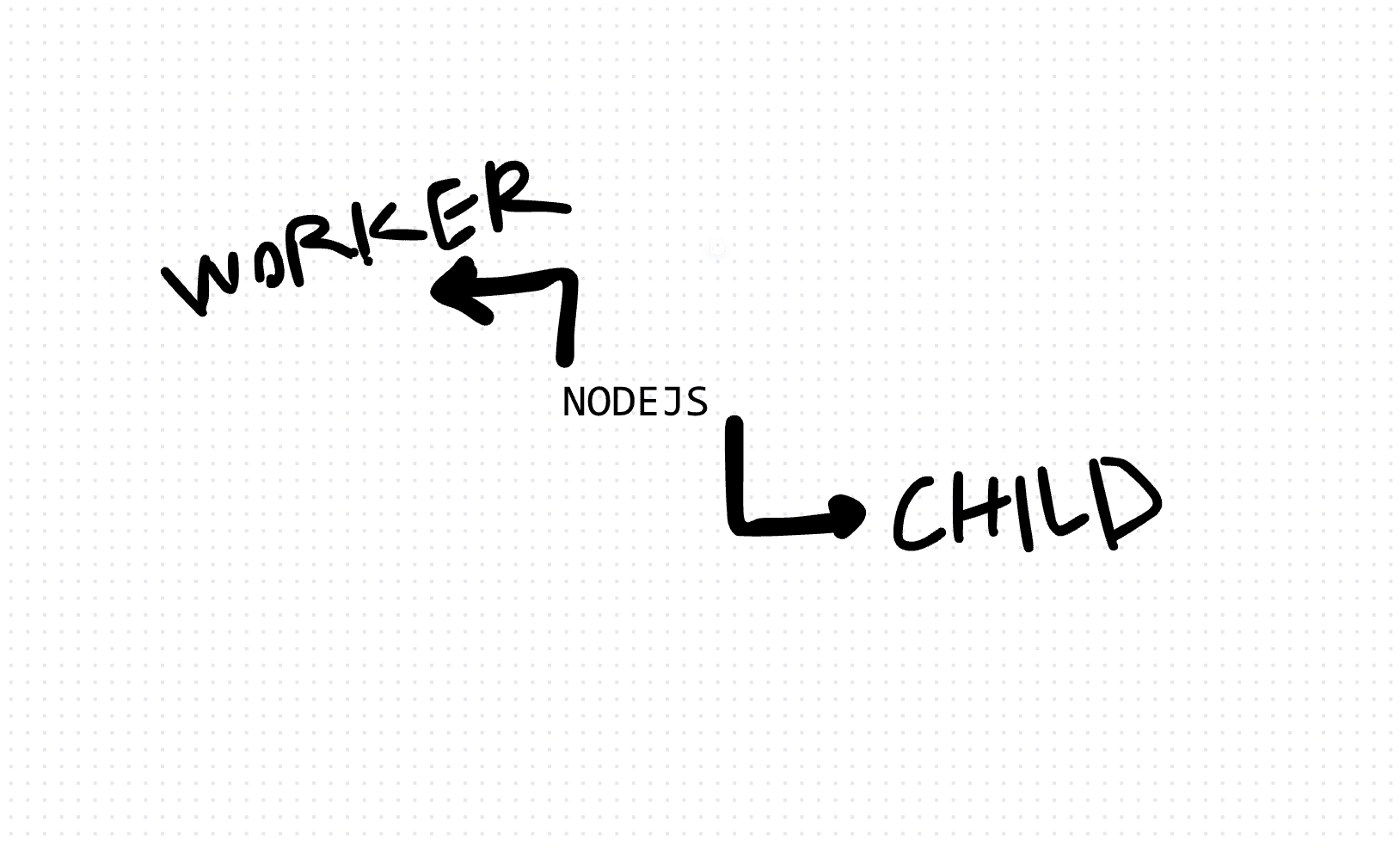
Have you ever found yourself tangled in the realm of Node.js concurrency, wondering what sets a child process apart from a worker thread? If so, you're not alone. In this article, we'll delve into the nuances between Node.js child processes and worker threads, exploring their differences, use cases, and how to implement them in TypeScript.
Node.js Child Process:
Node.js child processes offer a way to execute separate instances of the Node.js runtime, enabling parallelism by running tasks concurrently outside the main event loop. These processes are ideal for handling CPU-intensive operations, such as heavy computation or executing blocking tasks like file I/O.
Let's dive into a TypeScript example showcasing the use of a child process to compute the sum of an array of numbers:
import { fork } from 'child_process';
const childProcess = fork('./childProcess.ts');
// Listen for messages from the child process
childProcess.on('message', (message) => {
console.log('Sum computed by child process:', message);
});
// Send data to the child process for computation
childProcess.send({ numbers: [1, 2, 3, 4, 5] });
And childProcess.ts
// childProcess.ts
process.on('message', (data) => {
const sum = data.numbers.reduce((acc, num) => acc + num, 0);
// Send the computed sum back to the parent process
process.send(sum);
});
Node.js Worker:
On the other hand, Node.js workers, introduced in Node.js version 10.5.0, provide native support for creating and managing threads within a single Node.js process. These lightweight threads are designed for parallelizing I/O-bound tasks, such as handling network requests or processing asynchronous operations.
Let's explore a TypeScript example illustrating the use of a worker thread to perform an asynchronous task:
import { Worker, isMainThread } from 'worker_threads';
if (isMainThread) {
// Create a new worker thread
const worker = new Worker('./workerThread.ts');
// Listen for messages from the worker thread
worker.on('message', (message) => {
console.log('Result received from worker thread:', message);
});
// Send data to the worker thread for processing
worker.postMessage({ data: 'example data' });
}
And workerThread.ts
import { parentPort } from 'worker_threads';
// Perform asynchronous task and send result back to the main thread
const performTask = () => {
// Simulating an asynchronous operation
setTimeout(() => {
const result = 'Processed data';
// Send the result back to the main thread
parentPort.postMessage(result);
}, 2000);
};
// Invoke the performTask function
performTask();
- Tasks that involve synchronous or blocking operations, like file I/O or executing external commands, are well-suited for child processes. By offloading these operations to separate processes
- When dealing with asynchronous tasks that primarily involve I/O operations, such as network requests, database queries, or file system operations, worker threads excel at parallelizing these tasks within the same Node.js process
- Unlike child processes, which incur additional overhead due to the creation of separate instances of the Node.js runtime, worker threads are lightweight and share memory within the same process. As a result, they offer greater resource efficiency and reduced latency for tasks that don't require isolation.
In summary, Node.js child processes and worker threads serve distinct purposes in handling concurrency and parallelism within Node.js applications. While child processes excel at executing CPU-intensive tasks in separate instances of the Node.js runtime, worker threads are optimized for parallelizing I/O-bound operations within the same Node.js process. By understanding their differences and use cases, developers can leverage these features effectively to build scalable and efficient Node.js applications.