Object Properties and Methods in JavaScript and TypeScript - Interview Question
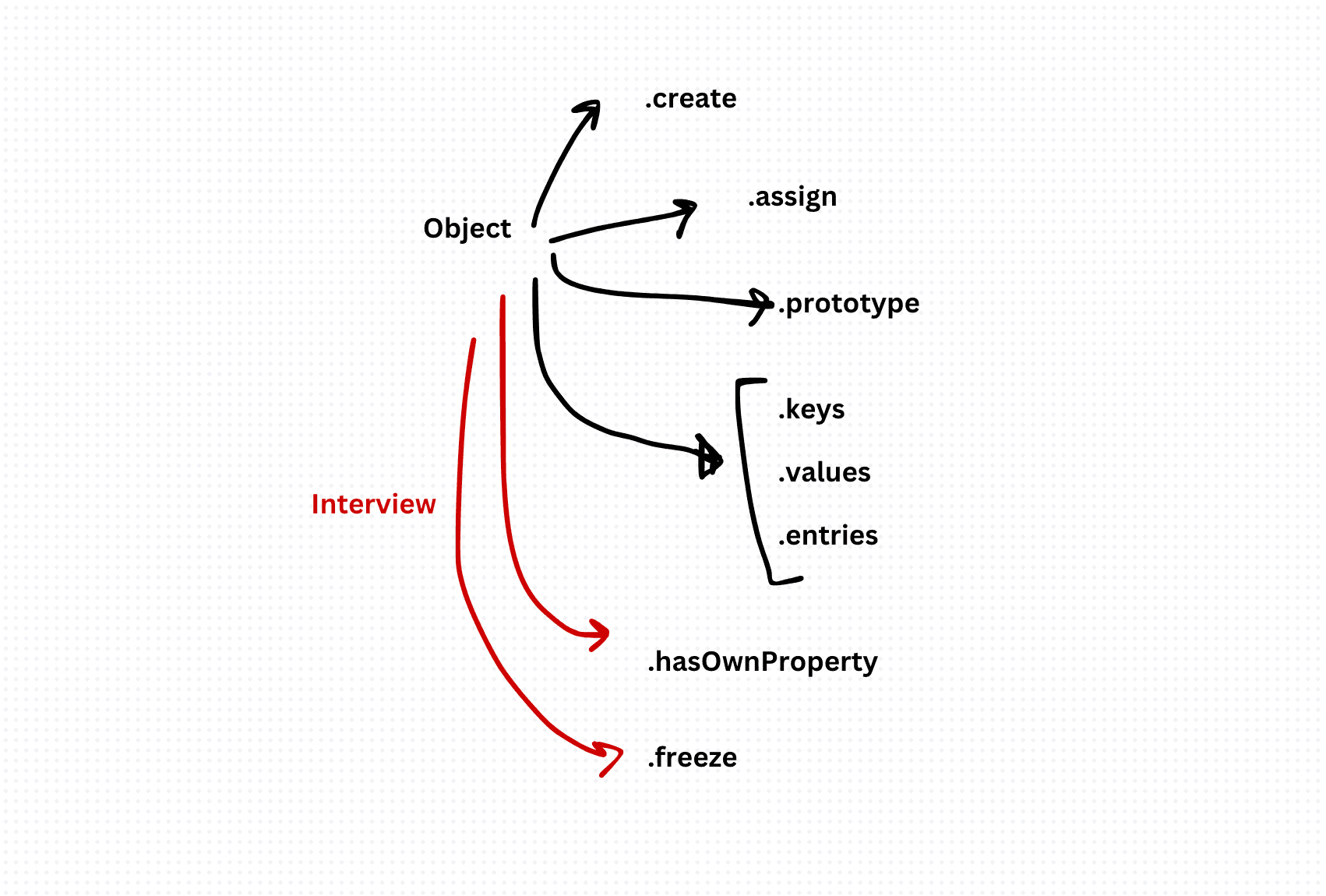
Exploring common object properties and methods that frequently appear in JavaScript interviews, covering everything from basic concepts to advanced techniques.
Object Properties:
Let's start by examining common object properties that you're likely to encounter in JavaScript interviews:
- Object.prototype: This property represents the prototype object associated with the Object constructor function. It provides shared properties and methods that are available to all objects created with the Object constructor.
console.log(Object.prototype); // Output: {}
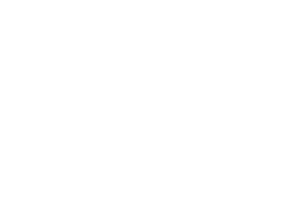
- Object.keys(): This method returns an array of a given object's own enumerable property names. It's often used to iterate over an object's properties or to check if an object has specific properties.
const obj = { a: 1, b: 2, c: 3 };
console.log(Object.keys(obj)); // Output: ["a", "b", "c"]
- Object.values(): This method returns an array of a given object's own enumerable property values. It complements Object.keys() and is commonly used when you need to access the values of an object's properties.
const obj = { a: 1, b: 2, c: 3 };
console.log(Object.values(obj)); // Output: [1, 2, 3]
- Object.entries(): This method returns an array of a given object's own enumerable property [key, value] pairs. It's useful for iterating over both keys and values simultaneously or converting an object into an array of key-value pairs.
const obj = { a: 1, b: 2, c: 3 };
console.log(Object.entries(obj)); // Output: [["a", 1], ["b", 2], ["c", 3]]
Next, let's explore commonly used object methods that are important for JavaScript developers:
- Object.assign(): This method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It's often used for object cloning or merging multiple objects into one.
const target = { a: 1, b: 2 };
const source = { b: 3, c: 4 };
const merged = Object.assign(target, source);
console.log(merged); // Output: { a: 1, b: 3, c: 4 }
- Object.create(): This method creates a new object with the specified prototype object and properties. It's commonly used to create objects with specific prototypes or to implement inheritance in JavaScript.
const person = {
greet() {
console.log('Hello!');
}
};
const john = Object.create(person);
john.greet(); // Output: Hello!
- Object.freeze(): This method freezes an object, preventing new properties from being added to it, existing properties from being removed, and changes to the values of existing properties. It's useful for creating immutable objects and ensuring data integrity.
const obj = { a: 1, b: 2 };
Object.freeze(obj);
obj.c = 3; // This assignment will be ignored in strict mode
console.log(obj); // Output: { a: 1, b: 2 }
- Object.hasOwnProperty(): This method returns a boolean indicating whether the specified object has the specified property as its own property (not inherited from its prototype chain). It's commonly used to check if an object has a specific property directly assigned to it.
const obj = { a: 1, b: 2 };
console.log(obj.hasOwnProperty('a')); // Output: true
console.log(obj.hasOwnProperty('toString')); // Output: false
Understanding JavaScript object properties and methods is crucial for mastering the language and excelling in interviews. By familiarizing yourself with common object properties like Object.keys() and Object.values() and methods like Object.assign() and Object.freeze(), you'll be better equipped to tackle JavaScript interview questions with confidence. So dive deep into the world of JavaScript objects, explore their capabilities, and elevate your JavaScript skills to new heights!