Omit, Pick, and Exclude in TypeScript
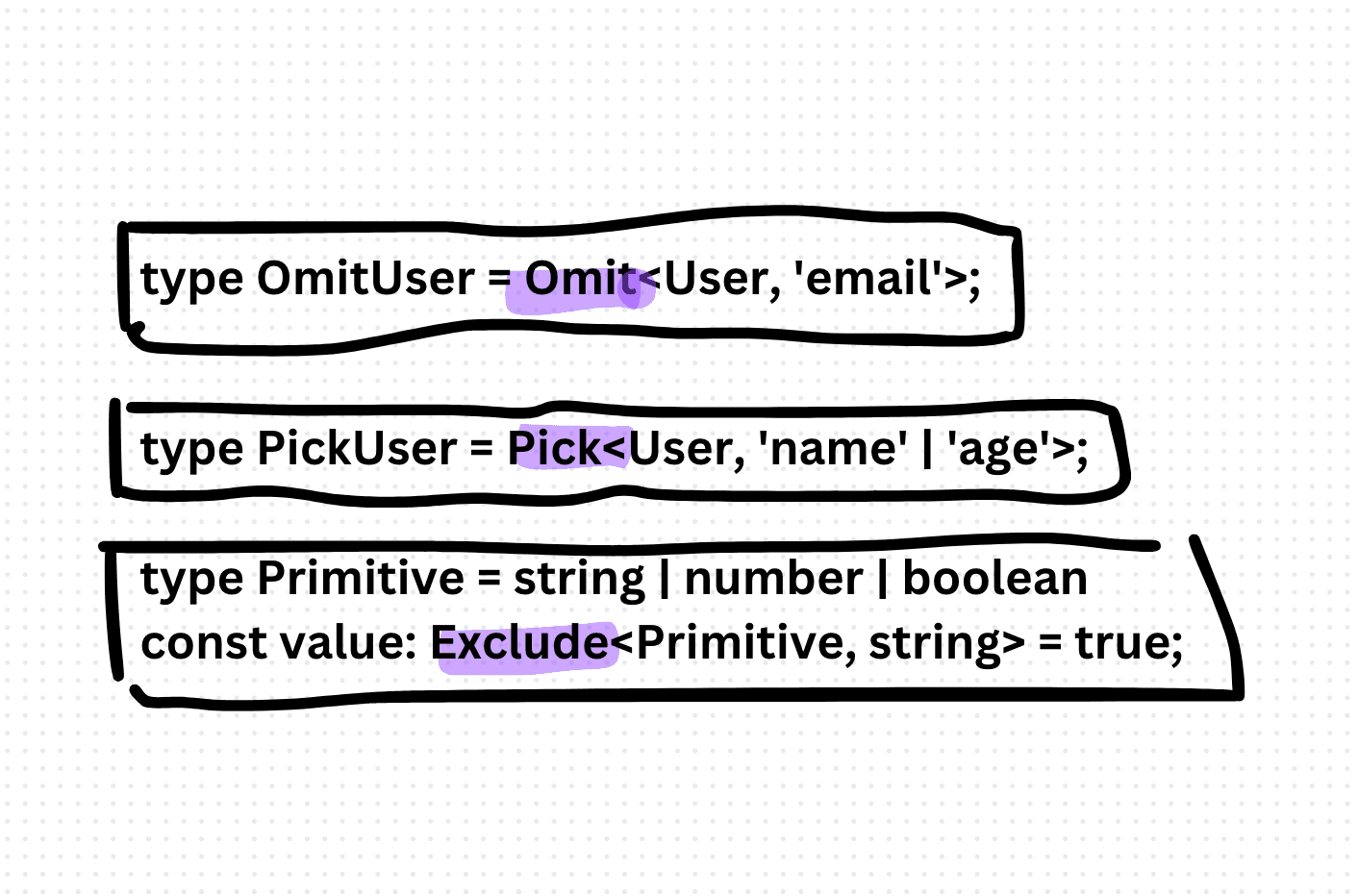
Omit is a type that represents a type that is the result of omitting certain properties from another type. Pick is a type that represents a type that is the result of picking certain properties from another type. Exclude is a type that represents a type that is the result of excluding certain properties from another type.
interface User {
name: string;
age: number;
email: string;
}
type OmitUser = Omit<User, 'email'>;
The OmitUser type will be a type that represents an object that has the same properties as the User interface, but without the email property.
type PickUser = Pick<User, 'name' | 'age'>;
The PickUser type will be a type that represents an object that has only the name and age properties from the User interface.
type ExcludeUser = Exclude<User, 'name' | 'age'>;
type Primitive = string | number | boolean
const value: Exclude<Primitive, string> = true;
The ExcludeUser type will be a type that represents an object that has the same properties as the User interface, but without the name and age properties.
Omit, Pick, and Exclude are powerful types in TypeScript that can be used to create powerful type guards that enforce the structure of an object. By using these types, you can improve the type safety, maintainability, and reusability of your code. In this article, we've seen how to use Omit, Pick, and Exclude to create type guards that enforce the structure of an object. With these types, you can write more robust and maintainable code.