Difference between Static and Instance Methods in TypeScript
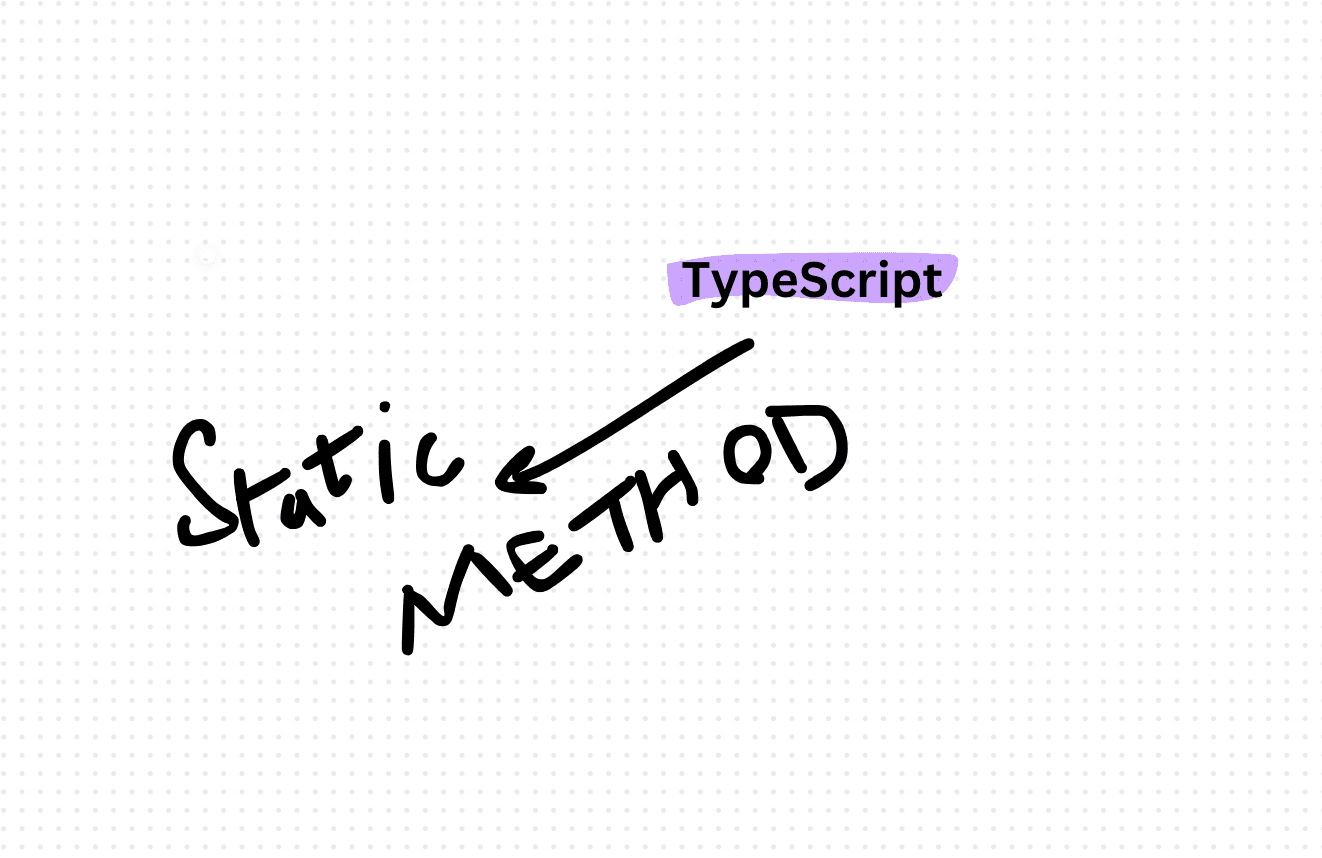
A static method is a method that is associated with a class, rather than an instance of the class. This means that a static method can be called without creating an instance of the class. Static methods are often used to provide utility functions that can be used by multiple instances of a class.
Static:
class MathUtil {
static add(a: number, b: number): number {
return a + b;
}
}
console.log(MathUtil.add(2, 3)); // Output: 5
In this example, we've defined a static method add that takes two numbers as arguments and returns their sum. We can call this method without creating an instance of the MathUtil class.
An instance method is a method that is associated with an instance of a class. This means that an instance method can only be called on an instance of the class. Instance methods are often used to provide functionality that is specific to a particular instance of a class.
class Person {
private name: string;
constructor(name: string) {
this.name = name;
}
sayHello(): void {
console.log(`Hello, my name is ${this.name}!`);
}
}
const person = new Person('John');
person.sayHello();
In this example, we've defined an instance method sayHello that is associated with an instance of the Person class. We can call this method on an instance of the Person class, but not on the class itself.
In conclusion, static methods are associated with a class, while instance methods are associated with an instance of a class. Static methods can be called without creating an instance of the class, while instance methods can only be called on an instance of the class. Understanding the difference between these two types of methods is important for writing effective and maintainable code.