Throttle and Debounce Functions in TypeScript
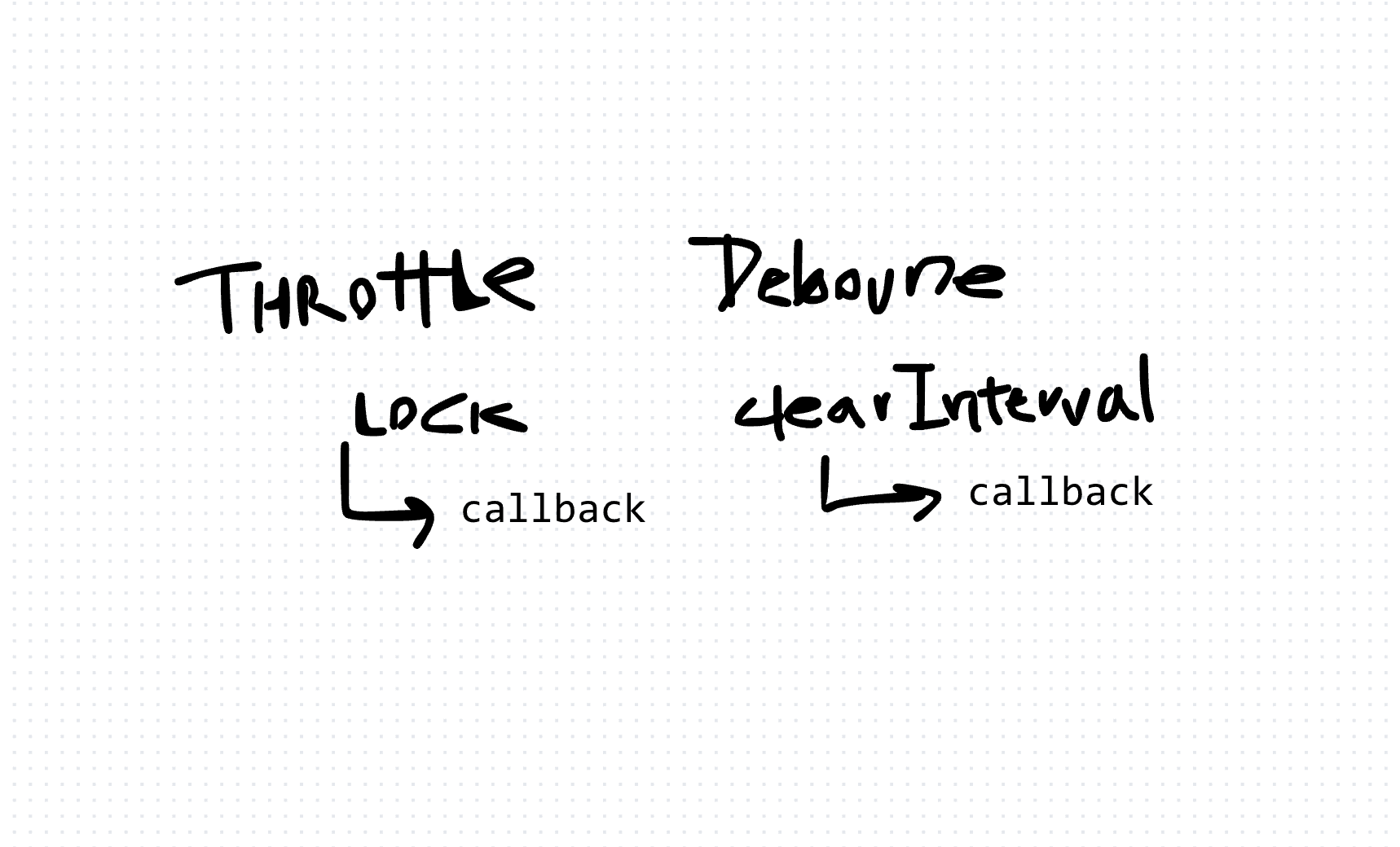
Throttling is a technique used to limit the number of times a function can be called within a specified time period. This is particularly useful when dealing with events that may trigger frequently, such as scrolling or resizing the window. By throttling the function, we ensure that it is executed at a controlled rate, preventing performance issues and excessive resource consumption.
const throttle = (callback: Function, delay: number) => {
var wait = false;
return function () {
if (!wait) {
callback();
wait = true;
setTimeout(function () {
wait = false;
}, delay);
}
};
};
window.addEventListener(
"scroll",
throttle(() => {
console.log("Scrolled!");
}, 200)
);
And debounce:
const debounce = (callback: Function, delay: number) => {
let timeout: NodeJS.Timeout | undefined;
return function () {
clearTimeout(timeout);
timeout = setTimeout(() => {
callback();
}, delay);
};
};
const searchInput = document.getElementById("search-input") as HTMLInputElement;
searchInput.addEventListener(
"input",
debounce((event) => {
console.log("Searching...", event.target.value);
}, 300)
);
Throttle and debounce functions are powerful tools in a TypeScript developer's toolkit. By understanding how they work and when to use them, you can improve the performance and responsiveness of your applications, especially in scenarios involving frequent user interactions or event handling. Experiment with these examples and explore how throttle and debounce can optimize your TypeScript projects.
Also great to read;