Type Systems in TypeScript: A Comprehensive Guide
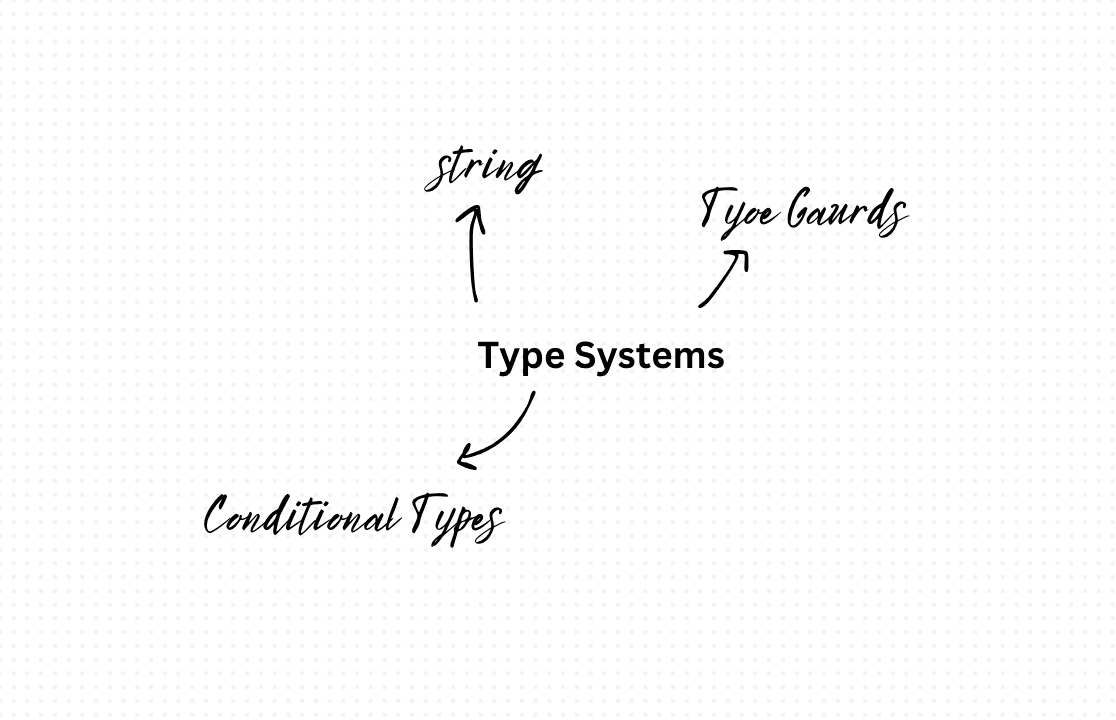
A type system is a set of rules that defines how data types are used in a programming language. In the context of TypeScript, the type system is responsible for checking the types of variables, function parameters, and return types at compile-time. This means that the type system checks the types of variables and function parameters before the code is executed, which helps to prevent type-related errors at runtime.
Type Annotations
While TypeScript can automatically infer the types of variables and function parameters, it is often necessary to explicitly define the types of variables and function parameters in your code. This is known as type annotation.
For example, consider the following code:
let name: string = 'John';
let age: number = 30;
Type Guards
Type guards are a way to narrow the type of a value within a specific scope. This is useful when you need to perform a type check on a value and then use that value in a specific way.
function isNumber<T>(value: T): value is number {
return typeof value === 'number';
}
let age: number | string = 30;
if (isNumber(age)) {
console.log(`Age: ${age}`);
} else {
console.log('Not a number');
}
Conditional Types
Conditional types are a way to define types that depend on the value of a variable. This is useful when you need to define a type that depends on the value of a variable.
type IsString<T> = T extends string ? string : never;
let name = 'John';
let age = 30;
type NameType = IsString<typeof name>;
type AgeType = IsString<typeof age>;
console.log(NameType); // Output: string
console.log(AgeType); // Output: never
In this article, we have explored the concept of type systems in TypeScript and provided examples to illustrate how they work. We have seen how TypeScript's type system can help you catch errors early in the development process and improve the overall maintainability of your code. We have also seen how type inference, type annotations, type guards, and conditional types can be used to define the types of variables and function parameters in your code.